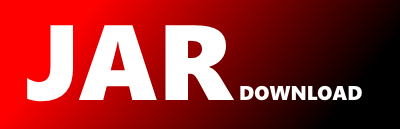
com.algolia.model.search.IgnorePlurals Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.algolia.utils.CompoundType;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import java.io.IOException;
import java.util.List;
import java.util.logging.Logger;
/**
* Treats singular, plurals, and other forms of declensions as matching terms. `ignorePlurals` is
* used in conjunction with the `queryLanguages` setting. _list_: language ISO codes for which
* ignoring plurals should be enabled. This list will override any values that you may have set in
* `queryLanguages`. _true_: enables the ignore plurals feature, where singulars and plurals are
* considered equivalent (\"foot\" = \"feet\"). The languages supported here are either [every
* language](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/supported-languages/)
* (this is the default) or those set by `queryLanguages`. _false_: turns off the ignore plurals
* feature, so that singulars and plurals aren't considered to be the same (\"foot\" will not find
* \"feet\").
*/
@JsonDeserialize(using = IgnorePlurals.Deserializer.class)
@JsonSerialize(using = IgnorePlurals.Serializer.class)
public interface IgnorePlurals extends CompoundType {
static IgnorePlurals of(Boolean inside) {
return new IgnorePluralsBoolean(inside);
}
static IgnorePlurals> of(List inside) {
return new IgnorePluralsListOfString(inside);
}
class Serializer extends StdSerializer {
public Serializer(Class t) {
super(t);
}
public Serializer() {
this(null);
}
@Override
public void serialize(IgnorePlurals value, JsonGenerator jgen, SerializerProvider provider) throws IOException {
jgen.writeObject(value.get());
}
}
class Deserializer extends StdDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
public Deserializer() {
this(IgnorePlurals.class);
}
public Deserializer(Class> vc) {
super(vc);
}
@Override
public IgnorePlurals deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize Boolean
if (tree.isValueNode()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Boolean value = parser.readValueAs(new TypeReference() {});
return IgnorePlurals.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Boolean (error: " + e.getMessage() + ") (type: Boolean)");
}
}
// deserialize List
if (tree.isArray()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
List value = parser.readValueAs(new TypeReference>() {});
return IgnorePlurals.of(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf List (error: " + e.getMessage() + ") (type: List)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public IgnorePlurals getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "IgnorePlurals cannot be null");
}
}
}
class IgnorePluralsBoolean implements IgnorePlurals {
private final Boolean value;
IgnorePluralsBoolean(Boolean value) {
this.value = value;
}
@Override
public Boolean get() {
return value;
}
}
class IgnorePluralsListOfString implements IgnorePlurals> {
private final List value;
IgnorePluralsListOfString(List value) {
this.value = value;
}
@Override
public List get() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy