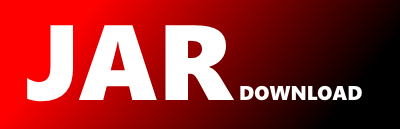
com.algolia.model.search.IndexSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/** Algolia index settings. */
public class IndexSettings {
@JsonProperty("replicas")
private List replicas;
@JsonProperty("paginationLimitedTo")
private Integer paginationLimitedTo;
@JsonProperty("unretrievableAttributes")
private List unretrievableAttributes;
@JsonProperty("disableTypoToleranceOnWords")
private List disableTypoToleranceOnWords;
@JsonProperty("attributesToTransliterate")
private List attributesToTransliterate;
@JsonProperty("camelCaseAttributes")
private List camelCaseAttributes;
@JsonProperty("decompoundedAttributes")
private Object decompoundedAttributes;
@JsonProperty("indexLanguages")
private List indexLanguages;
@JsonProperty("disablePrefixOnAttributes")
private List disablePrefixOnAttributes;
@JsonProperty("allowCompressionOfIntegerArray")
private Boolean allowCompressionOfIntegerArray;
@JsonProperty("numericAttributesForFiltering")
private List numericAttributesForFiltering;
@JsonProperty("separatorsToIndex")
private String separatorsToIndex;
@JsonProperty("searchableAttributes")
private List searchableAttributes;
@JsonProperty("userData")
private Object userData;
@JsonProperty("customNormalization")
private Map> customNormalization;
@JsonProperty("attributesForFaceting")
private List attributesForFaceting;
@JsonProperty("attributesToRetrieve")
private List attributesToRetrieve;
@JsonProperty("ranking")
private List ranking;
@JsonProperty("customRanking")
private List customRanking;
@JsonProperty("relevancyStrictness")
private Integer relevancyStrictness;
@JsonProperty("attributesToHighlight")
private List attributesToHighlight;
@JsonProperty("attributesToSnippet")
private List attributesToSnippet;
@JsonProperty("highlightPreTag")
private String highlightPreTag;
@JsonProperty("highlightPostTag")
private String highlightPostTag;
@JsonProperty("snippetEllipsisText")
private String snippetEllipsisText;
@JsonProperty("restrictHighlightAndSnippetArrays")
private Boolean restrictHighlightAndSnippetArrays;
@JsonProperty("hitsPerPage")
private Integer hitsPerPage;
@JsonProperty("minWordSizefor1Typo")
private Integer minWordSizefor1Typo;
@JsonProperty("minWordSizefor2Typos")
private Integer minWordSizefor2Typos;
@JsonProperty("typoTolerance")
private TypoTolerance typoTolerance;
@JsonProperty("allowTyposOnNumericTokens")
private Boolean allowTyposOnNumericTokens;
@JsonProperty("disableTypoToleranceOnAttributes")
private List disableTypoToleranceOnAttributes;
@JsonProperty("ignorePlurals")
private IgnorePlurals ignorePlurals;
@JsonProperty("removeStopWords")
private RemoveStopWords removeStopWords;
@JsonProperty("keepDiacriticsOnCharacters")
private String keepDiacriticsOnCharacters;
@JsonProperty("queryLanguages")
private List queryLanguages;
@JsonProperty("decompoundQuery")
private Boolean decompoundQuery;
@JsonProperty("enableRules")
private Boolean enableRules;
@JsonProperty("enablePersonalization")
private Boolean enablePersonalization;
@JsonProperty("queryType")
private QueryType queryType;
@JsonProperty("removeWordsIfNoResults")
private RemoveWordsIfNoResults removeWordsIfNoResults;
@JsonProperty("mode")
private Mode mode;
@JsonProperty("semanticSearch")
private SemanticSearch semanticSearch;
@JsonProperty("advancedSyntax")
private Boolean advancedSyntax;
@JsonProperty("optionalWords")
private List optionalWords;
@JsonProperty("disableExactOnAttributes")
private List disableExactOnAttributes;
@JsonProperty("exactOnSingleWordQuery")
private ExactOnSingleWordQuery exactOnSingleWordQuery;
@JsonProperty("alternativesAsExact")
private List alternativesAsExact;
@JsonProperty("advancedSyntaxFeatures")
private List advancedSyntaxFeatures;
@JsonProperty("distinct")
private Distinct distinct;
@JsonProperty("attributeForDistinct")
private String attributeForDistinct;
@JsonProperty("replaceSynonymsInHighlight")
private Boolean replaceSynonymsInHighlight;
@JsonProperty("minProximity")
private Integer minProximity;
@JsonProperty("responseFields")
private List responseFields;
@JsonProperty("maxFacetHits")
private Integer maxFacetHits;
@JsonProperty("maxValuesPerFacet")
private Integer maxValuesPerFacet;
@JsonProperty("sortFacetValuesBy")
private String sortFacetValuesBy;
@JsonProperty("attributeCriteriaComputedByMinProximity")
private Boolean attributeCriteriaComputedByMinProximity;
@JsonProperty("renderingContent")
private RenderingContent renderingContent;
@JsonProperty("enableReRanking")
private Boolean enableReRanking;
@JsonProperty("reRankingApplyFilter")
private ReRankingApplyFilter reRankingApplyFilter;
public IndexSettings setReplicas(List replicas) {
this.replicas = replicas;
return this;
}
public IndexSettings addReplicas(String replicasItem) {
if (this.replicas == null) {
this.replicas = new ArrayList<>();
}
this.replicas.add(replicasItem);
return this;
}
/**
* Creates
* [replicas](https://www.algolia.com/doc/guides/managing-results/refine-results/sorting/in-depth/replicas/),
* which are copies of a primary index with the same records but different settings.
*/
@javax.annotation.Nullable
public List getReplicas() {
return replicas;
}
public IndexSettings setPaginationLimitedTo(Integer paginationLimitedTo) {
this.paginationLimitedTo = paginationLimitedTo;
return this;
}
/** Maximum number of hits accessible through pagination. */
@javax.annotation.Nullable
public Integer getPaginationLimitedTo() {
return paginationLimitedTo;
}
public IndexSettings setUnretrievableAttributes(List unretrievableAttributes) {
this.unretrievableAttributes = unretrievableAttributes;
return this;
}
public IndexSettings addUnretrievableAttributes(String unretrievableAttributesItem) {
if (this.unretrievableAttributes == null) {
this.unretrievableAttributes = new ArrayList<>();
}
this.unretrievableAttributes.add(unretrievableAttributesItem);
return this;
}
/** Attributes that can't be retrieved at query time. */
@javax.annotation.Nullable
public List getUnretrievableAttributes() {
return unretrievableAttributes;
}
public IndexSettings setDisableTypoToleranceOnWords(List disableTypoToleranceOnWords) {
this.disableTypoToleranceOnWords = disableTypoToleranceOnWords;
return this;
}
public IndexSettings addDisableTypoToleranceOnWords(String disableTypoToleranceOnWordsItem) {
if (this.disableTypoToleranceOnWords == null) {
this.disableTypoToleranceOnWords = new ArrayList<>();
}
this.disableTypoToleranceOnWords.add(disableTypoToleranceOnWordsItem);
return this;
}
/**
* Words for which you want to turn off [typo
* tolerance](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/).
*/
@javax.annotation.Nullable
public List getDisableTypoToleranceOnWords() {
return disableTypoToleranceOnWords;
}
public IndexSettings setAttributesToTransliterate(List attributesToTransliterate) {
this.attributesToTransliterate = attributesToTransliterate;
return this;
}
public IndexSettings addAttributesToTransliterate(String attributesToTransliterateItem) {
if (this.attributesToTransliterate == null) {
this.attributesToTransliterate = new ArrayList<>();
}
this.attributesToTransliterate.add(attributesToTransliterateItem);
return this;
}
/**
* Attributes in your index to which [Japanese
* transliteration](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/language-specific-configurations/#japanese-transliteration-and-type-ahead)
* applies. This will ensure that words indexed in Katakana or Kanji can also be searched in
* Hiragana.
*/
@javax.annotation.Nullable
public List getAttributesToTransliterate() {
return attributesToTransliterate;
}
public IndexSettings setCamelCaseAttributes(List camelCaseAttributes) {
this.camelCaseAttributes = camelCaseAttributes;
return this;
}
public IndexSettings addCamelCaseAttributes(String camelCaseAttributesItem) {
if (this.camelCaseAttributes == null) {
this.camelCaseAttributes = new ArrayList<>();
}
this.camelCaseAttributes.add(camelCaseAttributesItem);
return this;
}
/** Attributes on which to split [camel case](https://wikipedia.org/wiki/Camel_case) words. */
@javax.annotation.Nullable
public List getCamelCaseAttributes() {
return camelCaseAttributes;
}
public IndexSettings setDecompoundedAttributes(Object decompoundedAttributes) {
this.decompoundedAttributes = decompoundedAttributes;
return this;
}
/**
* Attributes in your index to which [word
* segmentation](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/how-to/customize-segmentation/)
* (decompounding) applies.
*/
@javax.annotation.Nullable
public Object getDecompoundedAttributes() {
return decompoundedAttributes;
}
public IndexSettings setIndexLanguages(List indexLanguages) {
this.indexLanguages = indexLanguages;
return this;
}
public IndexSettings addIndexLanguages(String indexLanguagesItem) {
if (this.indexLanguages == null) {
this.indexLanguages = new ArrayList<>();
}
this.indexLanguages.add(indexLanguagesItem);
return this;
}
/**
* Set the languages of your index, for language-specific processing steps such as
* [tokenization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/tokenization/)
* and
* [normalization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/).
*/
@javax.annotation.Nullable
public List getIndexLanguages() {
return indexLanguages;
}
public IndexSettings setDisablePrefixOnAttributes(List disablePrefixOnAttributes) {
this.disablePrefixOnAttributes = disablePrefixOnAttributes;
return this;
}
public IndexSettings addDisablePrefixOnAttributes(String disablePrefixOnAttributesItem) {
if (this.disablePrefixOnAttributes == null) {
this.disablePrefixOnAttributes = new ArrayList<>();
}
this.disablePrefixOnAttributes.add(disablePrefixOnAttributesItem);
return this;
}
/**
* Attributes for which you want to turn off [prefix
* matching](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/#adjusting-prefix-search).
*/
@javax.annotation.Nullable
public List getDisablePrefixOnAttributes() {
return disablePrefixOnAttributes;
}
public IndexSettings setAllowCompressionOfIntegerArray(Boolean allowCompressionOfIntegerArray) {
this.allowCompressionOfIntegerArray = allowCompressionOfIntegerArray;
return this;
}
/**
* Incidates whether the engine compresses arrays with exclusively non-negative integers. When
* enabled, the compressed arrays may be reordered.
*/
@javax.annotation.Nullable
public Boolean getAllowCompressionOfIntegerArray() {
return allowCompressionOfIntegerArray;
}
public IndexSettings setNumericAttributesForFiltering(List numericAttributesForFiltering) {
this.numericAttributesForFiltering = numericAttributesForFiltering;
return this;
}
public IndexSettings addNumericAttributesForFiltering(String numericAttributesForFilteringItem) {
if (this.numericAttributesForFiltering == null) {
this.numericAttributesForFiltering = new ArrayList<>();
}
this.numericAttributesForFiltering.add(numericAttributesForFilteringItem);
return this;
}
/**
* Numeric attributes that can be used as [numerical
* filters](https://www.algolia.com/doc/guides/managing-results/rules/detecting-intent/how-to/applying-a-custom-filter-for-a-specific-query/#numerical-filters).
*/
@javax.annotation.Nullable
public List getNumericAttributesForFiltering() {
return numericAttributesForFiltering;
}
public IndexSettings setSeparatorsToIndex(String separatorsToIndex) {
this.separatorsToIndex = separatorsToIndex;
return this;
}
/**
* Controls which separators are added to an Algolia index as part of
* [normalization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/#what-does-normalization-mean).
* Separators are all non-letter characters except spaces and currency characters, such as $€£¥.
*/
@javax.annotation.Nullable
public String getSeparatorsToIndex() {
return separatorsToIndex;
}
public IndexSettings setSearchableAttributes(List searchableAttributes) {
this.searchableAttributes = searchableAttributes;
return this;
}
public IndexSettings addSearchableAttributes(String searchableAttributesItem) {
if (this.searchableAttributes == null) {
this.searchableAttributes = new ArrayList<>();
}
this.searchableAttributes.add(searchableAttributesItem);
return this;
}
/**
* [Attributes used for
* searching](https://www.algolia.com/doc/guides/managing-results/must-do/searchable-attributes/),
* including determining [if matches at the beginning of a word are important (ordered) or not
* (unordered)](https://www.algolia.com/doc/guides/managing-results/must-do/searchable-attributes/how-to/configuring-searchable-attributes-the-right-way/#understanding-word-position).
*/
@javax.annotation.Nullable
public List getSearchableAttributes() {
return searchableAttributes;
}
public IndexSettings setUserData(Object userData) {
this.userData = userData;
return this;
}
/** Lets you store custom data in your indices. */
@javax.annotation.Nullable
public Object getUserData() {
return userData;
}
public IndexSettings setCustomNormalization(Map> customNormalization) {
this.customNormalization = customNormalization;
return this;
}
public IndexSettings putCustomNormalization(String key, Map customNormalizationItem) {
if (this.customNormalization == null) {
this.customNormalization = new HashMap<>();
}
this.customNormalization.put(key, customNormalizationItem);
return this;
}
/**
* A list of characters and their normalized replacements to override Algolia's default
* [normalization](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/).
*/
@javax.annotation.Nullable
public Map> getCustomNormalization() {
return customNormalization;
}
public IndexSettings setAttributesForFaceting(List attributesForFaceting) {
this.attributesForFaceting = attributesForFaceting;
return this;
}
public IndexSettings addAttributesForFaceting(String attributesForFacetingItem) {
if (this.attributesForFaceting == null) {
this.attributesForFaceting = new ArrayList<>();
}
this.attributesForFaceting.add(attributesForFacetingItem);
return this;
}
/**
* Attributes used for
* [faceting](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/) and
* the
* [modifiers](https://www.algolia.com/doc/api-reference/api-parameters/attributesForFaceting/#modifiers)
* that can be applied: `filterOnly`, `searchable`, and `afterDistinct`.
*/
@javax.annotation.Nullable
public List getAttributesForFaceting() {
return attributesForFaceting;
}
public IndexSettings setAttributesToRetrieve(List attributesToRetrieve) {
this.attributesToRetrieve = attributesToRetrieve;
return this;
}
public IndexSettings addAttributesToRetrieve(String attributesToRetrieveItem) {
if (this.attributesToRetrieve == null) {
this.attributesToRetrieve = new ArrayList<>();
}
this.attributesToRetrieve.add(attributesToRetrieveItem);
return this;
}
/**
* Attributes to include in the API response. To reduce the size of your response, you can
* retrieve only some of the attributes. By default, the response includes all attributes.
*/
@javax.annotation.Nullable
public List getAttributesToRetrieve() {
return attributesToRetrieve;
}
public IndexSettings setRanking(List ranking) {
this.ranking = ranking;
return this;
}
public IndexSettings addRanking(String rankingItem) {
if (this.ranking == null) {
this.ranking = new ArrayList<>();
}
this.ranking.add(rankingItem);
return this;
}
/**
* Determines the order in which Algolia [returns your
* results](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/).
*/
@javax.annotation.Nullable
public List getRanking() {
return ranking;
}
public IndexSettings setCustomRanking(List customRanking) {
this.customRanking = customRanking;
return this;
}
public IndexSettings addCustomRanking(String customRankingItem) {
if (this.customRanking == null) {
this.customRanking = new ArrayList<>();
}
this.customRanking.add(customRankingItem);
return this;
}
/**
* Specifies the [Custom ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/must-do/custom-ranking/). Use
* the `asc` and `desc` modifiers to specify the ranking order: ascending or descending.
*/
@javax.annotation.Nullable
public List getCustomRanking() {
return customRanking;
}
public IndexSettings setRelevancyStrictness(Integer relevancyStrictness) {
this.relevancyStrictness = relevancyStrictness;
return this;
}
/** Relevancy threshold below which less relevant results aren't included in the results. */
@javax.annotation.Nullable
public Integer getRelevancyStrictness() {
return relevancyStrictness;
}
public IndexSettings setAttributesToHighlight(List attributesToHighlight) {
this.attributesToHighlight = attributesToHighlight;
return this;
}
public IndexSettings addAttributesToHighlight(String attributesToHighlightItem) {
if (this.attributesToHighlight == null) {
this.attributesToHighlight = new ArrayList<>();
}
this.attributesToHighlight.add(attributesToHighlightItem);
return this;
}
/**
* Attributes to highlight. Strings that match the search query in the attributes are highlighted
* by surrounding them with HTML tags (`highlightPreTag` and `highlightPostTag`).
*/
@javax.annotation.Nullable
public List getAttributesToHighlight() {
return attributesToHighlight;
}
public IndexSettings setAttributesToSnippet(List attributesToSnippet) {
this.attributesToSnippet = attributesToSnippet;
return this;
}
public IndexSettings addAttributesToSnippet(String attributesToSnippetItem) {
if (this.attributesToSnippet == null) {
this.attributesToSnippet = new ArrayList<>();
}
this.attributesToSnippet.add(attributesToSnippetItem);
return this;
}
/**
* Attributes to _snippet_. 'Snippeting' is shortening the attribute to a certain number of words.
* If not specified, the attribute is shortened to the 10 words around the matching string but you
* can specify the number. For example: `body:20`.
*/
@javax.annotation.Nullable
public List getAttributesToSnippet() {
return attributesToSnippet;
}
public IndexSettings setHighlightPreTag(String highlightPreTag) {
this.highlightPreTag = highlightPreTag;
return this;
}
/** HTML string to insert before the highlighted parts in all highlight and snippet results. */
@javax.annotation.Nullable
public String getHighlightPreTag() {
return highlightPreTag;
}
public IndexSettings setHighlightPostTag(String highlightPostTag) {
this.highlightPostTag = highlightPostTag;
return this;
}
/** HTML string to insert after the highlighted parts in all highlight and snippet results. */
@javax.annotation.Nullable
public String getHighlightPostTag() {
return highlightPostTag;
}
public IndexSettings setSnippetEllipsisText(String snippetEllipsisText) {
this.snippetEllipsisText = snippetEllipsisText;
return this;
}
/** String used as an ellipsis indicator when a snippet is truncated. */
@javax.annotation.Nullable
public String getSnippetEllipsisText() {
return snippetEllipsisText;
}
public IndexSettings setRestrictHighlightAndSnippetArrays(Boolean restrictHighlightAndSnippetArrays) {
this.restrictHighlightAndSnippetArrays = restrictHighlightAndSnippetArrays;
return this;
}
/** Restrict highlighting and snippeting to items that matched the query. */
@javax.annotation.Nullable
public Boolean getRestrictHighlightAndSnippetArrays() {
return restrictHighlightAndSnippetArrays;
}
public IndexSettings setHitsPerPage(Integer hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
/** Number of hits per page. minimum: 1 maximum: 1000 */
@javax.annotation.Nullable
public Integer getHitsPerPage() {
return hitsPerPage;
}
public IndexSettings setMinWordSizefor1Typo(Integer minWordSizefor1Typo) {
this.minWordSizefor1Typo = minWordSizefor1Typo;
return this;
}
/**
* Minimum number of characters a word in the query string must contain to accept matches with
* [one
* typo](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor1Typo() {
return minWordSizefor1Typo;
}
public IndexSettings setMinWordSizefor2Typos(Integer minWordSizefor2Typos) {
this.minWordSizefor2Typos = minWordSizefor2Typos;
return this;
}
/**
* Minimum number of characters a word in the query string must contain to accept matches with
* [two
* typos](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor2Typos() {
return minWordSizefor2Typos;
}
public IndexSettings setTypoTolerance(TypoTolerance typoTolerance) {
this.typoTolerance = typoTolerance;
return this;
}
/** Get typoTolerance */
@javax.annotation.Nullable
public TypoTolerance getTypoTolerance() {
return typoTolerance;
}
public IndexSettings setAllowTyposOnNumericTokens(Boolean allowTyposOnNumericTokens) {
this.allowTyposOnNumericTokens = allowTyposOnNumericTokens;
return this;
}
/** Whether to allow typos on numbers (\"numeric tokens\") in the query string. */
@javax.annotation.Nullable
public Boolean getAllowTyposOnNumericTokens() {
return allowTyposOnNumericTokens;
}
public IndexSettings setDisableTypoToleranceOnAttributes(List disableTypoToleranceOnAttributes) {
this.disableTypoToleranceOnAttributes = disableTypoToleranceOnAttributes;
return this;
}
public IndexSettings addDisableTypoToleranceOnAttributes(String disableTypoToleranceOnAttributesItem) {
if (this.disableTypoToleranceOnAttributes == null) {
this.disableTypoToleranceOnAttributes = new ArrayList<>();
}
this.disableTypoToleranceOnAttributes.add(disableTypoToleranceOnAttributesItem);
return this;
}
/**
* Attributes for which you want to turn off [typo
* tolerance](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/).
*/
@javax.annotation.Nullable
public List getDisableTypoToleranceOnAttributes() {
return disableTypoToleranceOnAttributes;
}
public IndexSettings setIgnorePlurals(IgnorePlurals ignorePlurals) {
this.ignorePlurals = ignorePlurals;
return this;
}
/** Get ignorePlurals */
@javax.annotation.Nullable
public IgnorePlurals getIgnorePlurals() {
return ignorePlurals;
}
public IndexSettings setRemoveStopWords(RemoveStopWords removeStopWords) {
this.removeStopWords = removeStopWords;
return this;
}
/** Get removeStopWords */
@javax.annotation.Nullable
public RemoveStopWords getRemoveStopWords() {
return removeStopWords;
}
public IndexSettings setKeepDiacriticsOnCharacters(String keepDiacriticsOnCharacters) {
this.keepDiacriticsOnCharacters = keepDiacriticsOnCharacters;
return this;
}
/**
* Characters that the engine shouldn't automatically
* [normalize](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/).
*/
@javax.annotation.Nullable
public String getKeepDiacriticsOnCharacters() {
return keepDiacriticsOnCharacters;
}
public IndexSettings setQueryLanguages(List queryLanguages) {
this.queryLanguages = queryLanguages;
return this;
}
public IndexSettings addQueryLanguages(String queryLanguagesItem) {
if (this.queryLanguages == null) {
this.queryLanguages = new ArrayList<>();
}
this.queryLanguages.add(queryLanguagesItem);
return this;
}
/**
* Sets your user's search language. This adjusts language-specific settings and features such as
* `ignorePlurals`, `removeStopWords`, and
* [CJK](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/#normalization-for-logogram-based-languages-cjk)
* word detection.
*/
@javax.annotation.Nullable
public List getQueryLanguages() {
return queryLanguages;
}
public IndexSettings setDecompoundQuery(Boolean decompoundQuery) {
this.decompoundQuery = decompoundQuery;
return this;
}
/**
* [Splits compound
* words](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/language-specific-configurations/#splitting-compound-words)
* into their component word parts in the query.
*/
@javax.annotation.Nullable
public Boolean getDecompoundQuery() {
return decompoundQuery;
}
public IndexSettings setEnableRules(Boolean enableRules) {
this.enableRules = enableRules;
return this;
}
/**
* Incidates whether
* [Rules](https://www.algolia.com/doc/guides/managing-results/rules/rules-overview/) are enabled.
*/
@javax.annotation.Nullable
public Boolean getEnableRules() {
return enableRules;
}
public IndexSettings setEnablePersonalization(Boolean enablePersonalization) {
this.enablePersonalization = enablePersonalization;
return this;
}
/**
* Incidates whether
* [Personalization](https://www.algolia.com/doc/guides/personalization/what-is-personalization/)
* is enabled.
*/
@javax.annotation.Nullable
public Boolean getEnablePersonalization() {
return enablePersonalization;
}
public IndexSettings setQueryType(QueryType queryType) {
this.queryType = queryType;
return this;
}
/** Get queryType */
@javax.annotation.Nullable
public QueryType getQueryType() {
return queryType;
}
public IndexSettings setRemoveWordsIfNoResults(RemoveWordsIfNoResults removeWordsIfNoResults) {
this.removeWordsIfNoResults = removeWordsIfNoResults;
return this;
}
/** Get removeWordsIfNoResults */
@javax.annotation.Nullable
public RemoveWordsIfNoResults getRemoveWordsIfNoResults() {
return removeWordsIfNoResults;
}
public IndexSettings setMode(Mode mode) {
this.mode = mode;
return this;
}
/** Get mode */
@javax.annotation.Nullable
public Mode getMode() {
return mode;
}
public IndexSettings setSemanticSearch(SemanticSearch semanticSearch) {
this.semanticSearch = semanticSearch;
return this;
}
/** Get semanticSearch */
@javax.annotation.Nullable
public SemanticSearch getSemanticSearch() {
return semanticSearch;
}
public IndexSettings setAdvancedSyntax(Boolean advancedSyntax) {
this.advancedSyntax = advancedSyntax;
return this;
}
/**
* Enables the [advanced query
* syntax](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/#advanced-syntax).
*/
@javax.annotation.Nullable
public Boolean getAdvancedSyntax() {
return advancedSyntax;
}
public IndexSettings setOptionalWords(List optionalWords) {
this.optionalWords = optionalWords;
return this;
}
public IndexSettings addOptionalWords(String optionalWordsItem) {
if (this.optionalWords == null) {
this.optionalWords = new ArrayList<>();
}
this.optionalWords.add(optionalWordsItem);
return this;
}
/**
* Words which should be considered
* [optional](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/empty-or-insufficient-results/#creating-a-list-of-optional-words)
* when found in a query.
*/
@javax.annotation.Nullable
public List getOptionalWords() {
return optionalWords;
}
public IndexSettings setDisableExactOnAttributes(List disableExactOnAttributes) {
this.disableExactOnAttributes = disableExactOnAttributes;
return this;
}
public IndexSettings addDisableExactOnAttributes(String disableExactOnAttributesItem) {
if (this.disableExactOnAttributes == null) {
this.disableExactOnAttributes = new ArrayList<>();
}
this.disableExactOnAttributes.add(disableExactOnAttributesItem);
return this;
}
/**
* Attributes for which you want to [turn off the exact ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/in-depth/adjust-exact-settings/#turn-off-exact-for-some-attributes).
*/
@javax.annotation.Nullable
public List getDisableExactOnAttributes() {
return disableExactOnAttributes;
}
public IndexSettings setExactOnSingleWordQuery(ExactOnSingleWordQuery exactOnSingleWordQuery) {
this.exactOnSingleWordQuery = exactOnSingleWordQuery;
return this;
}
/** Get exactOnSingleWordQuery */
@javax.annotation.Nullable
public ExactOnSingleWordQuery getExactOnSingleWordQuery() {
return exactOnSingleWordQuery;
}
public IndexSettings setAlternativesAsExact(List alternativesAsExact) {
this.alternativesAsExact = alternativesAsExact;
return this;
}
public IndexSettings addAlternativesAsExact(AlternativesAsExact alternativesAsExactItem) {
if (this.alternativesAsExact == null) {
this.alternativesAsExact = new ArrayList<>();
}
this.alternativesAsExact.add(alternativesAsExactItem);
return this;
}
/**
* Alternatives that should be considered an exact match by [the exact ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/in-depth/adjust-exact-settings/#turn-off-exact-for-some-attributes).
*/
@javax.annotation.Nullable
public List getAlternativesAsExact() {
return alternativesAsExact;
}
public IndexSettings setAdvancedSyntaxFeatures(List advancedSyntaxFeatures) {
this.advancedSyntaxFeatures = advancedSyntaxFeatures;
return this;
}
public IndexSettings addAdvancedSyntaxFeatures(AdvancedSyntaxFeatures advancedSyntaxFeaturesItem) {
if (this.advancedSyntaxFeatures == null) {
this.advancedSyntaxFeatures = new ArrayList<>();
}
this.advancedSyntaxFeatures.add(advancedSyntaxFeaturesItem);
return this;
}
/**
* Allows you to specify which advanced syntax features are active when `advancedSyntax` is
* enabled.
*/
@javax.annotation.Nullable
public List getAdvancedSyntaxFeatures() {
return advancedSyntaxFeatures;
}
public IndexSettings setDistinct(Distinct distinct) {
this.distinct = distinct;
return this;
}
/** Get distinct */
@javax.annotation.Nullable
public Distinct getDistinct() {
return distinct;
}
public IndexSettings setAttributeForDistinct(String attributeForDistinct) {
this.attributeForDistinct = attributeForDistinct;
return this;
}
/**
* Name of the deduplication attribute to be used with Algolia's [_distinct_
* feature](https://www.algolia.com/doc/guides/managing-results/refine-results/grouping/#introducing-algolias-distinct-feature).
*/
@javax.annotation.Nullable
public String getAttributeForDistinct() {
return attributeForDistinct;
}
public IndexSettings setReplaceSynonymsInHighlight(Boolean replaceSynonymsInHighlight) {
this.replaceSynonymsInHighlight = replaceSynonymsInHighlight;
return this;
}
/**
* Whether to highlight and snippet the original word that matches the synonym or the synonym
* itself.
*/
@javax.annotation.Nullable
public Boolean getReplaceSynonymsInHighlight() {
return replaceSynonymsInHighlight;
}
public IndexSettings setMinProximity(Integer minProximity) {
this.minProximity = minProximity;
return this;
}
/**
* Precision of the [proximity ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#proximity).
* minimum: 1 maximum: 7
*/
@javax.annotation.Nullable
public Integer getMinProximity() {
return minProximity;
}
public IndexSettings setResponseFields(List responseFields) {
this.responseFields = responseFields;
return this;
}
public IndexSettings addResponseFields(String responseFieldsItem) {
if (this.responseFields == null) {
this.responseFields = new ArrayList<>();
}
this.responseFields.add(responseFieldsItem);
return this;
}
/** Attributes to include in the API response for search and browse queries. */
@javax.annotation.Nullable
public List getResponseFields() {
return responseFields;
}
public IndexSettings setMaxFacetHits(Integer maxFacetHits) {
this.maxFacetHits = maxFacetHits;
return this;
}
/**
* Maximum number of facet hits to return when [searching for facet
* values](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/#search-for-facet-values).
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getMaxFacetHits() {
return maxFacetHits;
}
public IndexSettings setMaxValuesPerFacet(Integer maxValuesPerFacet) {
this.maxValuesPerFacet = maxValuesPerFacet;
return this;
}
/** Maximum number of facet values to return for each facet. */
@javax.annotation.Nullable
public Integer getMaxValuesPerFacet() {
return maxValuesPerFacet;
}
public IndexSettings setSortFacetValuesBy(String sortFacetValuesBy) {
this.sortFacetValuesBy = sortFacetValuesBy;
return this;
}
/** Controls how facet values are fetched. */
@javax.annotation.Nullable
public String getSortFacetValuesBy() {
return sortFacetValuesBy;
}
public IndexSettings setAttributeCriteriaComputedByMinProximity(Boolean attributeCriteriaComputedByMinProximity) {
this.attributeCriteriaComputedByMinProximity = attributeCriteriaComputedByMinProximity;
return this;
}
/**
* When the [Attribute criterion is ranked above
* Proximity](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#attribute-and-proximity-combinations)
* in your ranking formula, Proximity is used to select which searchable attribute is matched in
* the Attribute ranking stage.
*/
@javax.annotation.Nullable
public Boolean getAttributeCriteriaComputedByMinProximity() {
return attributeCriteriaComputedByMinProximity;
}
public IndexSettings setRenderingContent(RenderingContent renderingContent) {
this.renderingContent = renderingContent;
return this;
}
/** Get renderingContent */
@javax.annotation.Nullable
public RenderingContent getRenderingContent() {
return renderingContent;
}
public IndexSettings setEnableReRanking(Boolean enableReRanking) {
this.enableReRanking = enableReRanking;
return this;
}
/**
* Indicates whether this search will use [Dynamic
* Re-Ranking](https://www.algolia.com/doc/guides/algolia-ai/re-ranking/).
*/
@javax.annotation.Nullable
public Boolean getEnableReRanking() {
return enableReRanking;
}
public IndexSettings setReRankingApplyFilter(ReRankingApplyFilter reRankingApplyFilter) {
this.reRankingApplyFilter = reRankingApplyFilter;
return this;
}
/** Get reRankingApplyFilter */
@javax.annotation.Nullable
public ReRankingApplyFilter getReRankingApplyFilter() {
return reRankingApplyFilter;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IndexSettings indexSettings = (IndexSettings) o;
return (
Objects.equals(this.replicas, indexSettings.replicas) &&
Objects.equals(this.paginationLimitedTo, indexSettings.paginationLimitedTo) &&
Objects.equals(this.unretrievableAttributes, indexSettings.unretrievableAttributes) &&
Objects.equals(this.disableTypoToleranceOnWords, indexSettings.disableTypoToleranceOnWords) &&
Objects.equals(this.attributesToTransliterate, indexSettings.attributesToTransliterate) &&
Objects.equals(this.camelCaseAttributes, indexSettings.camelCaseAttributes) &&
Objects.equals(this.decompoundedAttributes, indexSettings.decompoundedAttributes) &&
Objects.equals(this.indexLanguages, indexSettings.indexLanguages) &&
Objects.equals(this.disablePrefixOnAttributes, indexSettings.disablePrefixOnAttributes) &&
Objects.equals(this.allowCompressionOfIntegerArray, indexSettings.allowCompressionOfIntegerArray) &&
Objects.equals(this.numericAttributesForFiltering, indexSettings.numericAttributesForFiltering) &&
Objects.equals(this.separatorsToIndex, indexSettings.separatorsToIndex) &&
Objects.equals(this.searchableAttributes, indexSettings.searchableAttributes) &&
Objects.equals(this.userData, indexSettings.userData) &&
Objects.equals(this.customNormalization, indexSettings.customNormalization) &&
Objects.equals(this.attributesForFaceting, indexSettings.attributesForFaceting) &&
Objects.equals(this.attributesToRetrieve, indexSettings.attributesToRetrieve) &&
Objects.equals(this.ranking, indexSettings.ranking) &&
Objects.equals(this.customRanking, indexSettings.customRanking) &&
Objects.equals(this.relevancyStrictness, indexSettings.relevancyStrictness) &&
Objects.equals(this.attributesToHighlight, indexSettings.attributesToHighlight) &&
Objects.equals(this.attributesToSnippet, indexSettings.attributesToSnippet) &&
Objects.equals(this.highlightPreTag, indexSettings.highlightPreTag) &&
Objects.equals(this.highlightPostTag, indexSettings.highlightPostTag) &&
Objects.equals(this.snippetEllipsisText, indexSettings.snippetEllipsisText) &&
Objects.equals(this.restrictHighlightAndSnippetArrays, indexSettings.restrictHighlightAndSnippetArrays) &&
Objects.equals(this.hitsPerPage, indexSettings.hitsPerPage) &&
Objects.equals(this.minWordSizefor1Typo, indexSettings.minWordSizefor1Typo) &&
Objects.equals(this.minWordSizefor2Typos, indexSettings.minWordSizefor2Typos) &&
Objects.equals(this.typoTolerance, indexSettings.typoTolerance) &&
Objects.equals(this.allowTyposOnNumericTokens, indexSettings.allowTyposOnNumericTokens) &&
Objects.equals(this.disableTypoToleranceOnAttributes, indexSettings.disableTypoToleranceOnAttributes) &&
Objects.equals(this.ignorePlurals, indexSettings.ignorePlurals) &&
Objects.equals(this.removeStopWords, indexSettings.removeStopWords) &&
Objects.equals(this.keepDiacriticsOnCharacters, indexSettings.keepDiacriticsOnCharacters) &&
Objects.equals(this.queryLanguages, indexSettings.queryLanguages) &&
Objects.equals(this.decompoundQuery, indexSettings.decompoundQuery) &&
Objects.equals(this.enableRules, indexSettings.enableRules) &&
Objects.equals(this.enablePersonalization, indexSettings.enablePersonalization) &&
Objects.equals(this.queryType, indexSettings.queryType) &&
Objects.equals(this.removeWordsIfNoResults, indexSettings.removeWordsIfNoResults) &&
Objects.equals(this.mode, indexSettings.mode) &&
Objects.equals(this.semanticSearch, indexSettings.semanticSearch) &&
Objects.equals(this.advancedSyntax, indexSettings.advancedSyntax) &&
Objects.equals(this.optionalWords, indexSettings.optionalWords) &&
Objects.equals(this.disableExactOnAttributes, indexSettings.disableExactOnAttributes) &&
Objects.equals(this.exactOnSingleWordQuery, indexSettings.exactOnSingleWordQuery) &&
Objects.equals(this.alternativesAsExact, indexSettings.alternativesAsExact) &&
Objects.equals(this.advancedSyntaxFeatures, indexSettings.advancedSyntaxFeatures) &&
Objects.equals(this.distinct, indexSettings.distinct) &&
Objects.equals(this.attributeForDistinct, indexSettings.attributeForDistinct) &&
Objects.equals(this.replaceSynonymsInHighlight, indexSettings.replaceSynonymsInHighlight) &&
Objects.equals(this.minProximity, indexSettings.minProximity) &&
Objects.equals(this.responseFields, indexSettings.responseFields) &&
Objects.equals(this.maxFacetHits, indexSettings.maxFacetHits) &&
Objects.equals(this.maxValuesPerFacet, indexSettings.maxValuesPerFacet) &&
Objects.equals(this.sortFacetValuesBy, indexSettings.sortFacetValuesBy) &&
Objects.equals(this.attributeCriteriaComputedByMinProximity, indexSettings.attributeCriteriaComputedByMinProximity) &&
Objects.equals(this.renderingContent, indexSettings.renderingContent) &&
Objects.equals(this.enableReRanking, indexSettings.enableReRanking) &&
Objects.equals(this.reRankingApplyFilter, indexSettings.reRankingApplyFilter)
);
}
@Override
public int hashCode() {
return Objects.hash(
replicas,
paginationLimitedTo,
unretrievableAttributes,
disableTypoToleranceOnWords,
attributesToTransliterate,
camelCaseAttributes,
decompoundedAttributes,
indexLanguages,
disablePrefixOnAttributes,
allowCompressionOfIntegerArray,
numericAttributesForFiltering,
separatorsToIndex,
searchableAttributes,
userData,
customNormalization,
attributesForFaceting,
attributesToRetrieve,
ranking,
customRanking,
relevancyStrictness,
attributesToHighlight,
attributesToSnippet,
highlightPreTag,
highlightPostTag,
snippetEllipsisText,
restrictHighlightAndSnippetArrays,
hitsPerPage,
minWordSizefor1Typo,
minWordSizefor2Typos,
typoTolerance,
allowTyposOnNumericTokens,
disableTypoToleranceOnAttributes,
ignorePlurals,
removeStopWords,
keepDiacriticsOnCharacters,
queryLanguages,
decompoundQuery,
enableRules,
enablePersonalization,
queryType,
removeWordsIfNoResults,
mode,
semanticSearch,
advancedSyntax,
optionalWords,
disableExactOnAttributes,
exactOnSingleWordQuery,
alternativesAsExact,
advancedSyntaxFeatures,
distinct,
attributeForDistinct,
replaceSynonymsInHighlight,
minProximity,
responseFields,
maxFacetHits,
maxValuesPerFacet,
sortFacetValuesBy,
attributeCriteriaComputedByMinProximity,
renderingContent,
enableReRanking,
reRankingApplyFilter
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class IndexSettings {\n");
sb.append(" replicas: ").append(toIndentedString(replicas)).append("\n");
sb.append(" paginationLimitedTo: ").append(toIndentedString(paginationLimitedTo)).append("\n");
sb.append(" unretrievableAttributes: ").append(toIndentedString(unretrievableAttributes)).append("\n");
sb.append(" disableTypoToleranceOnWords: ").append(toIndentedString(disableTypoToleranceOnWords)).append("\n");
sb.append(" attributesToTransliterate: ").append(toIndentedString(attributesToTransliterate)).append("\n");
sb.append(" camelCaseAttributes: ").append(toIndentedString(camelCaseAttributes)).append("\n");
sb.append(" decompoundedAttributes: ").append(toIndentedString(decompoundedAttributes)).append("\n");
sb.append(" indexLanguages: ").append(toIndentedString(indexLanguages)).append("\n");
sb.append(" disablePrefixOnAttributes: ").append(toIndentedString(disablePrefixOnAttributes)).append("\n");
sb.append(" allowCompressionOfIntegerArray: ").append(toIndentedString(allowCompressionOfIntegerArray)).append("\n");
sb.append(" numericAttributesForFiltering: ").append(toIndentedString(numericAttributesForFiltering)).append("\n");
sb.append(" separatorsToIndex: ").append(toIndentedString(separatorsToIndex)).append("\n");
sb.append(" searchableAttributes: ").append(toIndentedString(searchableAttributes)).append("\n");
sb.append(" userData: ").append(toIndentedString(userData)).append("\n");
sb.append(" customNormalization: ").append(toIndentedString(customNormalization)).append("\n");
sb.append(" attributesForFaceting: ").append(toIndentedString(attributesForFaceting)).append("\n");
sb.append(" attributesToRetrieve: ").append(toIndentedString(attributesToRetrieve)).append("\n");
sb.append(" ranking: ").append(toIndentedString(ranking)).append("\n");
sb.append(" customRanking: ").append(toIndentedString(customRanking)).append("\n");
sb.append(" relevancyStrictness: ").append(toIndentedString(relevancyStrictness)).append("\n");
sb.append(" attributesToHighlight: ").append(toIndentedString(attributesToHighlight)).append("\n");
sb.append(" attributesToSnippet: ").append(toIndentedString(attributesToSnippet)).append("\n");
sb.append(" highlightPreTag: ").append(toIndentedString(highlightPreTag)).append("\n");
sb.append(" highlightPostTag: ").append(toIndentedString(highlightPostTag)).append("\n");
sb.append(" snippetEllipsisText: ").append(toIndentedString(snippetEllipsisText)).append("\n");
sb.append(" restrictHighlightAndSnippetArrays: ").append(toIndentedString(restrictHighlightAndSnippetArrays)).append("\n");
sb.append(" hitsPerPage: ").append(toIndentedString(hitsPerPage)).append("\n");
sb.append(" minWordSizefor1Typo: ").append(toIndentedString(minWordSizefor1Typo)).append("\n");
sb.append(" minWordSizefor2Typos: ").append(toIndentedString(minWordSizefor2Typos)).append("\n");
sb.append(" typoTolerance: ").append(toIndentedString(typoTolerance)).append("\n");
sb.append(" allowTyposOnNumericTokens: ").append(toIndentedString(allowTyposOnNumericTokens)).append("\n");
sb.append(" disableTypoToleranceOnAttributes: ").append(toIndentedString(disableTypoToleranceOnAttributes)).append("\n");
sb.append(" ignorePlurals: ").append(toIndentedString(ignorePlurals)).append("\n");
sb.append(" removeStopWords: ").append(toIndentedString(removeStopWords)).append("\n");
sb.append(" keepDiacriticsOnCharacters: ").append(toIndentedString(keepDiacriticsOnCharacters)).append("\n");
sb.append(" queryLanguages: ").append(toIndentedString(queryLanguages)).append("\n");
sb.append(" decompoundQuery: ").append(toIndentedString(decompoundQuery)).append("\n");
sb.append(" enableRules: ").append(toIndentedString(enableRules)).append("\n");
sb.append(" enablePersonalization: ").append(toIndentedString(enablePersonalization)).append("\n");
sb.append(" queryType: ").append(toIndentedString(queryType)).append("\n");
sb.append(" removeWordsIfNoResults: ").append(toIndentedString(removeWordsIfNoResults)).append("\n");
sb.append(" mode: ").append(toIndentedString(mode)).append("\n");
sb.append(" semanticSearch: ").append(toIndentedString(semanticSearch)).append("\n");
sb.append(" advancedSyntax: ").append(toIndentedString(advancedSyntax)).append("\n");
sb.append(" optionalWords: ").append(toIndentedString(optionalWords)).append("\n");
sb.append(" disableExactOnAttributes: ").append(toIndentedString(disableExactOnAttributes)).append("\n");
sb.append(" exactOnSingleWordQuery: ").append(toIndentedString(exactOnSingleWordQuery)).append("\n");
sb.append(" alternativesAsExact: ").append(toIndentedString(alternativesAsExact)).append("\n");
sb.append(" advancedSyntaxFeatures: ").append(toIndentedString(advancedSyntaxFeatures)).append("\n");
sb.append(" distinct: ").append(toIndentedString(distinct)).append("\n");
sb.append(" attributeForDistinct: ").append(toIndentedString(attributeForDistinct)).append("\n");
sb.append(" replaceSynonymsInHighlight: ").append(toIndentedString(replaceSynonymsInHighlight)).append("\n");
sb.append(" minProximity: ").append(toIndentedString(minProximity)).append("\n");
sb.append(" responseFields: ").append(toIndentedString(responseFields)).append("\n");
sb.append(" maxFacetHits: ").append(toIndentedString(maxFacetHits)).append("\n");
sb.append(" maxValuesPerFacet: ").append(toIndentedString(maxValuesPerFacet)).append("\n");
sb.append(" sortFacetValuesBy: ").append(toIndentedString(sortFacetValuesBy)).append("\n");
sb
.append(" attributeCriteriaComputedByMinProximity: ")
.append(toIndentedString(attributeCriteriaComputedByMinProximity))
.append("\n");
sb.append(" renderingContent: ").append(toIndentedString(renderingContent)).append("\n");
sb.append(" enableReRanking: ").append(toIndentedString(enableReRanking)).append("\n");
sb.append(" reRankingApplyFilter: ").append(toIndentedString(reRankingApplyFilter)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy