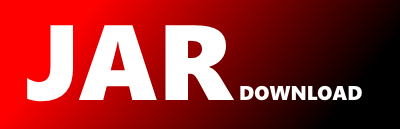
com.algolia.model.search.OperationIndexParams Maven / Gradle / Ivy
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** OperationIndexParams */
public class OperationIndexParams {
@JsonProperty("operation")
private OperationType operation;
@JsonProperty("destination")
private String destination;
@JsonProperty("scope")
private List scope;
public OperationIndexParams setOperation(OperationType operation) {
this.operation = operation;
return this;
}
/** Get operation */
@javax.annotation.Nonnull
public OperationType getOperation() {
return operation;
}
public OperationIndexParams setDestination(String destination) {
this.destination = destination;
return this;
}
/** Index name (case-sensitive). */
@javax.annotation.Nonnull
public String getDestination() {
return destination;
}
public OperationIndexParams setScope(List scope) {
this.scope = scope;
return this;
}
public OperationIndexParams addScope(ScopeType scopeItem) {
if (this.scope == null) {
this.scope = new ArrayList<>();
}
this.scope.add(scopeItem);
return this;
}
/**
* **Only for copying.** If you specify a scope, only the selected scopes are copied. Records and
* the other scopes are left unchanged. If you omit the `scope` parameter, everything is copied:
* records, settings, synonyms, and rules.
*/
@javax.annotation.Nullable
public List getScope() {
return scope;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OperationIndexParams operationIndexParams = (OperationIndexParams) o;
return (
Objects.equals(this.operation, operationIndexParams.operation) &&
Objects.equals(this.destination, operationIndexParams.destination) &&
Objects.equals(this.scope, operationIndexParams.scope)
);
}
@Override
public int hashCode() {
return Objects.hash(operation, destination, scope);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OperationIndexParams {\n");
sb.append(" operation: ").append(toIndentedString(operation)).append("\n");
sb.append(" destination: ").append(toIndentedString(destination)).append("\n");
sb.append(" scope: ").append(toIndentedString(scope)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy