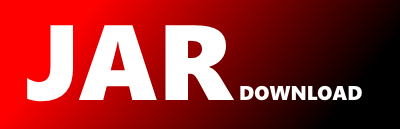
com.algolia.config.ClientOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
package com.algolia.config;
import com.algolia.internal.HttpRequester;
import com.algolia.utils.ExecutorUtils;
import com.fasterxml.jackson.databind.json.JsonMapper;
import java.time.Duration;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ExecutorService;
import java.util.function.Consumer;
import javax.annotation.Nonnull;
public final class ClientOptions implements ClientConfig {
public static Builder builder() {
return new Builder();
}
private final List algoliaAgentSegments;
private final List hosts;
private final LogLevel logLevel;
private final Duration connectTimeout;
private final Duration writeTimeout;
private final Duration readTimeout;
private final Map defaultHeaders;
private final CompressionType compressionType;
private final Requester customRequester;
private final Logger logger;
private final Consumer requesterConfig;
private final Consumer mapperConfig;
private final ExecutorService executor;
public ClientOptions() {
this(new Builder());
}
ClientOptions(Builder builder) {
this.algoliaAgentSegments = builder.algoliaAgentSegments;
this.hosts = builder.hosts;
this.customRequester = builder.customRequester;
this.logLevel = builder.logLevel;
this.connectTimeout = builder.connectTimeout;
this.writeTimeout = builder.writeTimeout;
this.readTimeout = builder.readTimeout;
this.defaultHeaders = builder.defaultHeaders;
this.compressionType = builder.compressionType;
this.logger = builder.logger;
this.requesterConfig = builder.requesterConfig;
this.mapperConfig = builder.mapperConfig;
this.executor = builder.executor != null ? builder.executor : ExecutorUtils.newThreadPool();
}
@Nonnull
public List getAlgoliaAgentSegments() {
return algoliaAgentSegments;
}
public List getHosts() {
return hosts;
}
@Nonnull
public LogLevel getLogLevel() {
return logLevel;
}
@Nonnull
public Duration getConnectTimeout() {
return connectTimeout;
}
@Nonnull
public Duration getWriteTimeout() {
return writeTimeout;
}
@Nonnull
public Duration getReadTimeout() {
return readTimeout;
}
@Nonnull
public Map getDefaultHeaders() {
return defaultHeaders;
}
@Nonnull
public CompressionType getCompressionType() {
return compressionType;
}
public Requester getCustomRequester() {
return customRequester;
}
@Nonnull
public Logger getLogger() {
return logger;
}
public Consumer getRequesterConfig() {
return requesterConfig;
}
public Consumer getMapperConfig() {
return mapperConfig;
}
public ExecutorService getExecutor() {
return executor;
}
public static class Builder {
public ExecutorService executor;
private Requester customRequester;
private List hosts;
private Logger logger;
private Consumer requesterConfig;
private Consumer mapperConfig;
private final List algoliaAgentSegments = new ArrayList<>();
private final Map defaultHeaders = new HashMap<>();
private LogLevel logLevel = LogLevel.NONE;
private Duration connectTimeout = Duration.ZERO;
private Duration writeTimeout = Duration.ZERO;
private Duration readTimeout = Duration.ZERO;
private CompressionType compressionType = CompressionType.NONE;
public Builder setRequester(Requester requester) {
this.customRequester = requester;
return this;
}
public Builder addAlgoliaAgentSegment(AlgoliaAgent.Segment segment) {
this.algoliaAgentSegments.add(segment);
return this;
}
public Builder addAlgoliaAgentSegment(String value, String version) {
AlgoliaAgent.Segment segment = new AlgoliaAgent.Segment(value, version);
return addAlgoliaAgentSegment(segment);
}
public Builder addAlgoliaAgentSegment(String value) {
AlgoliaAgent.Segment segment = new AlgoliaAgent.Segment(value);
return addAlgoliaAgentSegment(segment);
}
public Builder addAlgoliaAgentSegments(List segments) {
this.algoliaAgentSegments.addAll(segments);
return this;
}
public Builder addDefaultHeader(String header, String value) {
this.defaultHeaders.put(header, value);
return this;
}
public Builder setHosts(List hosts) {
this.hosts = hosts;
return this;
}
public Builder setLogLevel(LogLevel logLevel) {
this.logLevel = logLevel;
return this;
}
public Builder setConnectTimeout(Duration connectTimeout) {
this.connectTimeout = connectTimeout;
return this;
}
public Builder setWriteTimeout(Duration writeTimeout) {
this.writeTimeout = writeTimeout;
return this;
}
public Builder setReadTimeout(Duration readTimeout) {
this.readTimeout = readTimeout;
return this;
}
public Builder setCompressionType(CompressionType compressionType) {
this.compressionType = compressionType;
return this;
}
public Builder setCustomRequester(Requester customRequester) {
this.customRequester = customRequester;
return this;
}
public Builder setLogger(Logger logger) {
this.logger = logger;
return this;
}
public Builder setRequesterConfig(Consumer requesterConfig) {
this.requesterConfig = requesterConfig;
return this;
}
public Builder setMapperConfig(Consumer mapperConfig) {
this.mapperConfig = mapperConfig;
return this;
}
public Builder setExecutor(ExecutorService executor) {
this.executor = executor;
return this;
}
public ClientOptions build() {
return new ClientOptions(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy