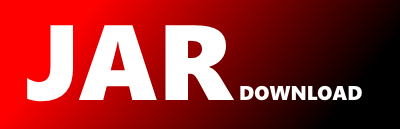
com.algolia.model.abtesting.ABTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.abtesting;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/** ABTest */
public class ABTest {
@JsonProperty("abTestID")
private Integer abTestID;
@JsonProperty("clickSignificance")
private Double clickSignificance;
@JsonProperty("conversionSignificance")
private Double conversionSignificance;
@JsonProperty("addToCartSignificance")
private Double addToCartSignificance;
@JsonProperty("purchaseSignificance")
private Double purchaseSignificance;
@JsonProperty("revenueSignificance")
private Map revenueSignificance;
@JsonProperty("updatedAt")
private String updatedAt;
@JsonProperty("createdAt")
private String createdAt;
@JsonProperty("endAt")
private String endAt;
@JsonProperty("name")
private String name;
@JsonProperty("status")
private Status status;
@JsonProperty("variants")
private List variants = new ArrayList<>();
@JsonProperty("configuration")
private ABTestConfiguration configuration;
public ABTest setAbTestID(Integer abTestID) {
this.abTestID = abTestID;
return this;
}
/** Unique A/B test identifier. */
@javax.annotation.Nonnull
public Integer getAbTestID() {
return abTestID;
}
public ABTest setClickSignificance(Double clickSignificance) {
this.clickSignificance = clickSignificance;
return this;
}
/** Get clickSignificance */
@javax.annotation.Nullable
public Double getClickSignificance() {
return clickSignificance;
}
public ABTest setConversionSignificance(Double conversionSignificance) {
this.conversionSignificance = conversionSignificance;
return this;
}
/** Get conversionSignificance */
@javax.annotation.Nullable
public Double getConversionSignificance() {
return conversionSignificance;
}
public ABTest setAddToCartSignificance(Double addToCartSignificance) {
this.addToCartSignificance = addToCartSignificance;
return this;
}
/** Get addToCartSignificance */
@javax.annotation.Nullable
public Double getAddToCartSignificance() {
return addToCartSignificance;
}
public ABTest setPurchaseSignificance(Double purchaseSignificance) {
this.purchaseSignificance = purchaseSignificance;
return this;
}
/** Get purchaseSignificance */
@javax.annotation.Nullable
public Double getPurchaseSignificance() {
return purchaseSignificance;
}
public ABTest setRevenueSignificance(Map revenueSignificance) {
this.revenueSignificance = revenueSignificance;
return this;
}
public ABTest putRevenueSignificance(String key, Double revenueSignificanceItem) {
if (this.revenueSignificance == null) {
this.revenueSignificance = new HashMap<>();
}
this.revenueSignificance.put(key, revenueSignificanceItem);
return this;
}
/** Get revenueSignificance */
@javax.annotation.Nullable
public Map getRevenueSignificance() {
return revenueSignificance;
}
public ABTest setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/** Date and time when the A/B test was last updated, in RFC 3339 format. */
@javax.annotation.Nonnull
public String getUpdatedAt() {
return updatedAt;
}
public ABTest setCreatedAt(String createdAt) {
this.createdAt = createdAt;
return this;
}
/** Date and time when the A/B test was created, in RFC 3339 format. */
@javax.annotation.Nonnull
public String getCreatedAt() {
return createdAt;
}
public ABTest setEndAt(String endAt) {
this.endAt = endAt;
return this;
}
/** End date and time of the A/B test, in RFC 3339 format. */
@javax.annotation.Nonnull
public String getEndAt() {
return endAt;
}
public ABTest setName(String name) {
this.name = name;
return this;
}
/** A/B test name. */
@javax.annotation.Nonnull
public String getName() {
return name;
}
public ABTest setStatus(Status status) {
this.status = status;
return this;
}
/** Get status */
@javax.annotation.Nonnull
public Status getStatus() {
return status;
}
public ABTest setVariants(List variants) {
this.variants = variants;
return this;
}
public ABTest addVariants(Variant variantsItem) {
this.variants.add(variantsItem);
return this;
}
/**
* A/B test variants. The first variant is your _control_ index, typically your production index.
* The second variant is an index with changed settings that you want to test against the control.
*/
@javax.annotation.Nonnull
public List getVariants() {
return variants;
}
public ABTest setConfiguration(ABTestConfiguration configuration) {
this.configuration = configuration;
return this;
}
/** Get configuration */
@javax.annotation.Nullable
public ABTestConfiguration getConfiguration() {
return configuration;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ABTest abTest = (ABTest) o;
return (
Objects.equals(this.abTestID, abTest.abTestID) &&
Objects.equals(this.clickSignificance, abTest.clickSignificance) &&
Objects.equals(this.conversionSignificance, abTest.conversionSignificance) &&
Objects.equals(this.addToCartSignificance, abTest.addToCartSignificance) &&
Objects.equals(this.purchaseSignificance, abTest.purchaseSignificance) &&
Objects.equals(this.revenueSignificance, abTest.revenueSignificance) &&
Objects.equals(this.updatedAt, abTest.updatedAt) &&
Objects.equals(this.createdAt, abTest.createdAt) &&
Objects.equals(this.endAt, abTest.endAt) &&
Objects.equals(this.name, abTest.name) &&
Objects.equals(this.status, abTest.status) &&
Objects.equals(this.variants, abTest.variants) &&
Objects.equals(this.configuration, abTest.configuration)
);
}
@Override
public int hashCode() {
return Objects.hash(
abTestID,
clickSignificance,
conversionSignificance,
addToCartSignificance,
purchaseSignificance,
revenueSignificance,
updatedAt,
createdAt,
endAt,
name,
status,
variants,
configuration
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ABTest {\n");
sb.append(" abTestID: ").append(toIndentedString(abTestID)).append("\n");
sb.append(" clickSignificance: ").append(toIndentedString(clickSignificance)).append("\n");
sb.append(" conversionSignificance: ").append(toIndentedString(conversionSignificance)).append("\n");
sb.append(" addToCartSignificance: ").append(toIndentedString(addToCartSignificance)).append("\n");
sb.append(" purchaseSignificance: ").append(toIndentedString(purchaseSignificance)).append("\n");
sb.append(" revenueSignificance: ").append(toIndentedString(revenueSignificance)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" endAt: ").append(toIndentedString(endAt)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" variants: ").append(toIndentedString(variants)).append("\n");
sb.append(" configuration: ").append(toIndentedString(configuration)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy