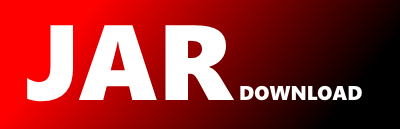
com.algolia.model.analytics.GetClickThroughRateResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.analytics;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** GetClickThroughRateResponse */
public class GetClickThroughRateResponse {
@JsonProperty("rate")
private Double rate;
@JsonProperty("clickCount")
private Integer clickCount;
@JsonProperty("trackedSearchCount")
private Integer trackedSearchCount;
@JsonProperty("dates")
private List dates = new ArrayList<>();
public GetClickThroughRateResponse setRate(Double rate) {
this.rate = rate;
return this;
}
/**
* Click-through rate, calculated as number of tracked searches with at least one click event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getRate() {
return rate;
}
public GetClickThroughRateResponse setClickCount(Integer clickCount) {
this.clickCount = clickCount;
return this;
}
/** Number of clicks associated with this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getClickCount() {
return clickCount;
}
public GetClickThroughRateResponse setTrackedSearchCount(Integer trackedSearchCount) {
this.trackedSearchCount = trackedSearchCount;
return this;
}
/**
* Number of tracked searches. Tracked searches are search requests where the `clickAnalytics`
* parameter is true.
*/
@javax.annotation.Nonnull
public Integer getTrackedSearchCount() {
return trackedSearchCount;
}
public GetClickThroughRateResponse setDates(List dates) {
this.dates = dates;
return this;
}
public GetClickThroughRateResponse addDates(DailyClickThroughRates datesItem) {
this.dates.add(datesItem);
return this;
}
/** Daily click-through rates. */
@javax.annotation.Nonnull
public List getDates() {
return dates;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetClickThroughRateResponse getClickThroughRateResponse = (GetClickThroughRateResponse) o;
return (
Objects.equals(this.rate, getClickThroughRateResponse.rate) &&
Objects.equals(this.clickCount, getClickThroughRateResponse.clickCount) &&
Objects.equals(this.trackedSearchCount, getClickThroughRateResponse.trackedSearchCount) &&
Objects.equals(this.dates, getClickThroughRateResponse.dates)
);
}
@Override
public int hashCode() {
return Objects.hash(rate, clickCount, trackedSearchCount, dates);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetClickThroughRateResponse {\n");
sb.append(" rate: ").append(toIndentedString(rate)).append("\n");
sb.append(" clickCount: ").append(toIndentedString(clickCount)).append("\n");
sb.append(" trackedSearchCount: ").append(toIndentedString(trackedSearchCount)).append("\n");
sb.append(" dates: ").append(toIndentedString(dates)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy