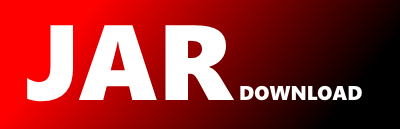
com.algolia.model.analytics.TopHitWithRevenueAnalytics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.analytics;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/** TopHitWithRevenueAnalytics */
public class TopHitWithRevenueAnalytics {
@JsonProperty("hit")
private String hit;
@JsonProperty("count")
private Integer count;
@JsonProperty("clickThroughRate")
private Double clickThroughRate;
@JsonProperty("conversionRate")
private Double conversionRate;
@JsonProperty("trackedHitCount")
private Integer trackedHitCount;
@JsonProperty("clickCount")
private Integer clickCount;
@JsonProperty("conversionCount")
private Integer conversionCount;
@JsonProperty("addToCartRate")
private Double addToCartRate;
@JsonProperty("addToCartCount")
private Integer addToCartCount;
@JsonProperty("purchaseRate")
private Double purchaseRate;
@JsonProperty("purchaseCount")
private Integer purchaseCount;
@JsonProperty("currencies")
private Map currencies = new HashMap<>();
public TopHitWithRevenueAnalytics setHit(String hit) {
this.hit = hit;
return this;
}
/** Object ID of a record that's returned as a search result. */
@javax.annotation.Nonnull
public String getHit() {
return hit;
}
public TopHitWithRevenueAnalytics setCount(Integer count) {
this.count = count;
return this;
}
/** Number of occurrences. */
@javax.annotation.Nonnull
public Integer getCount() {
return count;
}
public TopHitWithRevenueAnalytics setClickThroughRate(Double clickThroughRate) {
this.clickThroughRate = clickThroughRate;
return this;
}
/**
* Click-through rate, calculated as number of tracked searches with at least one click event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getClickThroughRate() {
return clickThroughRate;
}
public TopHitWithRevenueAnalytics setConversionRate(Double conversionRate) {
this.conversionRate = conversionRate;
return this;
}
/**
* Conversion rate, calculated as number of tracked searches with at least one conversion event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getConversionRate() {
return conversionRate;
}
public TopHitWithRevenueAnalytics setTrackedHitCount(Integer trackedHitCount) {
this.trackedHitCount = trackedHitCount;
return this;
}
/**
* Number of tracked searches. Tracked searches are search requests where the `clickAnalytics`
* parameter is true.
*/
@javax.annotation.Nonnull
public Integer getTrackedHitCount() {
return trackedHitCount;
}
public TopHitWithRevenueAnalytics setClickCount(Integer clickCount) {
this.clickCount = clickCount;
return this;
}
/** Number of clicks associated with this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getClickCount() {
return clickCount;
}
public TopHitWithRevenueAnalytics setConversionCount(Integer conversionCount) {
this.conversionCount = conversionCount;
return this;
}
/** Number of conversions from this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getConversionCount() {
return conversionCount;
}
public TopHitWithRevenueAnalytics setAddToCartRate(Double addToCartRate) {
this.addToCartRate = addToCartRate;
return this;
}
/**
* Add-to-cart rate, calculated as number of tracked searches with at least one add-to-cart event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getAddToCartRate() {
return addToCartRate;
}
public TopHitWithRevenueAnalytics setAddToCartCount(Integer addToCartCount) {
this.addToCartCount = addToCartCount;
return this;
}
/** Number of add-to-cart events from this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getAddToCartCount() {
return addToCartCount;
}
public TopHitWithRevenueAnalytics setPurchaseRate(Double purchaseRate) {
this.purchaseRate = purchaseRate;
return this;
}
/**
* Purchase rate, calculated as number of tracked searches with at least one purchase event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getPurchaseRate() {
return purchaseRate;
}
public TopHitWithRevenueAnalytics setPurchaseCount(Integer purchaseCount) {
this.purchaseCount = purchaseCount;
return this;
}
/** Number of purchase events from this search. */
@javax.annotation.Nonnull
public Integer getPurchaseCount() {
return purchaseCount;
}
public TopHitWithRevenueAnalytics setCurrencies(Map currencies) {
this.currencies = currencies;
return this;
}
public TopHitWithRevenueAnalytics putCurrencies(String key, CurrencyCode currenciesItem) {
this.currencies.put(key, currenciesItem);
return this;
}
/** Revenue associated with this search, broken-down by currencies. */
@javax.annotation.Nonnull
public Map getCurrencies() {
return currencies;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TopHitWithRevenueAnalytics topHitWithRevenueAnalytics = (TopHitWithRevenueAnalytics) o;
return (
Objects.equals(this.hit, topHitWithRevenueAnalytics.hit) &&
Objects.equals(this.count, topHitWithRevenueAnalytics.count) &&
Objects.equals(this.clickThroughRate, topHitWithRevenueAnalytics.clickThroughRate) &&
Objects.equals(this.conversionRate, topHitWithRevenueAnalytics.conversionRate) &&
Objects.equals(this.trackedHitCount, topHitWithRevenueAnalytics.trackedHitCount) &&
Objects.equals(this.clickCount, topHitWithRevenueAnalytics.clickCount) &&
Objects.equals(this.conversionCount, topHitWithRevenueAnalytics.conversionCount) &&
Objects.equals(this.addToCartRate, topHitWithRevenueAnalytics.addToCartRate) &&
Objects.equals(this.addToCartCount, topHitWithRevenueAnalytics.addToCartCount) &&
Objects.equals(this.purchaseRate, topHitWithRevenueAnalytics.purchaseRate) &&
Objects.equals(this.purchaseCount, topHitWithRevenueAnalytics.purchaseCount) &&
Objects.equals(this.currencies, topHitWithRevenueAnalytics.currencies)
);
}
@Override
public int hashCode() {
return Objects.hash(
hit,
count,
clickThroughRate,
conversionRate,
trackedHitCount,
clickCount,
conversionCount,
addToCartRate,
addToCartCount,
purchaseRate,
purchaseCount,
currencies
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TopHitWithRevenueAnalytics {\n");
sb.append(" hit: ").append(toIndentedString(hit)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" clickThroughRate: ").append(toIndentedString(clickThroughRate)).append("\n");
sb.append(" conversionRate: ").append(toIndentedString(conversionRate)).append("\n");
sb.append(" trackedHitCount: ").append(toIndentedString(trackedHitCount)).append("\n");
sb.append(" clickCount: ").append(toIndentedString(clickCount)).append("\n");
sb.append(" conversionCount: ").append(toIndentedString(conversionCount)).append("\n");
sb.append(" addToCartRate: ").append(toIndentedString(addToCartRate)).append("\n");
sb.append(" addToCartCount: ").append(toIndentedString(addToCartCount)).append("\n");
sb.append(" purchaseRate: ").append(toIndentedString(purchaseRate)).append("\n");
sb.append(" purchaseCount: ").append(toIndentedString(purchaseCount)).append("\n");
sb.append(" currencies: ").append(toIndentedString(currencies)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy