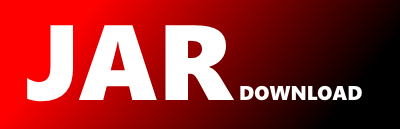
com.algolia.model.analytics.TopSearchWithAnalytics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.analytics;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** TopSearchWithAnalytics */
public class TopSearchWithAnalytics {
@JsonProperty("search")
private String search;
@JsonProperty("count")
private Integer count;
@JsonProperty("clickThroughRate")
private Double clickThroughRate;
@JsonProperty("averageClickPosition")
private Double averageClickPosition;
@JsonProperty("clickPositions")
private List clickPositions = new ArrayList<>();
@JsonProperty("conversionRate")
private Double conversionRate;
@JsonProperty("trackedSearchCount")
private Integer trackedSearchCount;
@JsonProperty("clickCount")
private Integer clickCount;
@JsonProperty("conversionCount")
private Integer conversionCount;
@JsonProperty("nbHits")
private Integer nbHits;
public TopSearchWithAnalytics setSearch(String search) {
this.search = search;
return this;
}
/** Search query. */
@javax.annotation.Nonnull
public String getSearch() {
return search;
}
public TopSearchWithAnalytics setCount(Integer count) {
this.count = count;
return this;
}
/** Number of searches. */
@javax.annotation.Nonnull
public Integer getCount() {
return count;
}
public TopSearchWithAnalytics setClickThroughRate(Double clickThroughRate) {
this.clickThroughRate = clickThroughRate;
return this;
}
/**
* Click-through rate, calculated as number of tracked searches with at least one click event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getClickThroughRate() {
return clickThroughRate;
}
public TopSearchWithAnalytics setAverageClickPosition(Double averageClickPosition) {
this.averageClickPosition = averageClickPosition;
return this;
}
/**
* Average position of a clicked search result in the list of search results. If null, Algolia
* didn't receive any search requests with `clickAnalytics` set to true. minimum: 1
*/
@javax.annotation.Nullable
public Double getAverageClickPosition() {
return averageClickPosition;
}
public TopSearchWithAnalytics setClickPositions(List clickPositions) {
this.clickPositions = clickPositions;
return this;
}
public TopSearchWithAnalytics addClickPositions(ClickPosition clickPositionsItem) {
this.clickPositions.add(clickPositionsItem);
return this;
}
/** List of positions in the search results and clicks associated with this search. */
@javax.annotation.Nonnull
public List getClickPositions() {
return clickPositions;
}
public TopSearchWithAnalytics setConversionRate(Double conversionRate) {
this.conversionRate = conversionRate;
return this;
}
/**
* Conversion rate, calculated as number of tracked searches with at least one conversion event
* divided by the number of tracked searches. If null, Algolia didn't receive any search requests
* with `clickAnalytics` set to true. minimum: 0 maximum: 1
*/
@javax.annotation.Nullable
public Double getConversionRate() {
return conversionRate;
}
public TopSearchWithAnalytics setTrackedSearchCount(Integer trackedSearchCount) {
this.trackedSearchCount = trackedSearchCount;
return this;
}
/**
* Number of tracked searches. Tracked searches are search requests where the `clickAnalytics`
* parameter is true.
*/
@javax.annotation.Nonnull
public Integer getTrackedSearchCount() {
return trackedSearchCount;
}
public TopSearchWithAnalytics setClickCount(Integer clickCount) {
this.clickCount = clickCount;
return this;
}
/** Number of clicks associated with this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getClickCount() {
return clickCount;
}
public TopSearchWithAnalytics setConversionCount(Integer conversionCount) {
this.conversionCount = conversionCount;
return this;
}
/** Number of conversions from this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getConversionCount() {
return conversionCount;
}
public TopSearchWithAnalytics setNbHits(Integer nbHits) {
this.nbHits = nbHits;
return this;
}
/** Number of results (hits). */
@javax.annotation.Nonnull
public Integer getNbHits() {
return nbHits;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TopSearchWithAnalytics topSearchWithAnalytics = (TopSearchWithAnalytics) o;
return (
Objects.equals(this.search, topSearchWithAnalytics.search) &&
Objects.equals(this.count, topSearchWithAnalytics.count) &&
Objects.equals(this.clickThroughRate, topSearchWithAnalytics.clickThroughRate) &&
Objects.equals(this.averageClickPosition, topSearchWithAnalytics.averageClickPosition) &&
Objects.equals(this.clickPositions, topSearchWithAnalytics.clickPositions) &&
Objects.equals(this.conversionRate, topSearchWithAnalytics.conversionRate) &&
Objects.equals(this.trackedSearchCount, topSearchWithAnalytics.trackedSearchCount) &&
Objects.equals(this.clickCount, topSearchWithAnalytics.clickCount) &&
Objects.equals(this.conversionCount, topSearchWithAnalytics.conversionCount) &&
Objects.equals(this.nbHits, topSearchWithAnalytics.nbHits)
);
}
@Override
public int hashCode() {
return Objects.hash(
search,
count,
clickThroughRate,
averageClickPosition,
clickPositions,
conversionRate,
trackedSearchCount,
clickCount,
conversionCount,
nbHits
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TopSearchWithAnalytics {\n");
sb.append(" search: ").append(toIndentedString(search)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" clickThroughRate: ").append(toIndentedString(clickThroughRate)).append("\n");
sb.append(" averageClickPosition: ").append(toIndentedString(averageClickPosition)).append("\n");
sb.append(" clickPositions: ").append(toIndentedString(clickPositions)).append("\n");
sb.append(" conversionRate: ").append(toIndentedString(conversionRate)).append("\n");
sb.append(" trackedSearchCount: ").append(toIndentedString(trackedSearchCount)).append("\n");
sb.append(" clickCount: ").append(toIndentedString(clickCount)).append("\n");
sb.append(" conversionCount: ").append(toIndentedString(conversionCount)).append("\n");
sb.append(" nbHits: ").append(toIndentedString(nbHits)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy