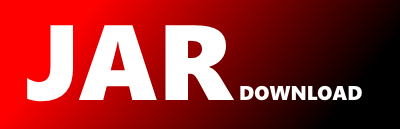
com.algolia.model.ingestion.AuthOAuth Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** Credentials for authenticating with OAuth 2.0. */
@JsonDeserialize(as = AuthOAuth.class)
public class AuthOAuth implements AuthInput {
@JsonProperty("url")
private String url;
@JsonProperty("client_id")
private String clientId;
@JsonProperty("client_secret")
private String clientSecret;
@JsonProperty("scope")
private String scope;
public AuthOAuth setUrl(String url) {
this.url = url;
return this;
}
/** URL for the OAuth endpoint. */
@javax.annotation.Nonnull
public String getUrl() {
return url;
}
public AuthOAuth setClientId(String clientId) {
this.clientId = clientId;
return this;
}
/** Client ID. */
@javax.annotation.Nonnull
public String getClientId() {
return clientId;
}
public AuthOAuth setClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/** Client secret. This field is `null` in the API response. */
@javax.annotation.Nonnull
public String getClientSecret() {
return clientSecret;
}
public AuthOAuth setScope(String scope) {
this.scope = scope;
return this;
}
/** OAuth scope. */
@javax.annotation.Nullable
public String getScope() {
return scope;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuthOAuth authOAuth = (AuthOAuth) o;
return (
Objects.equals(this.url, authOAuth.url) &&
Objects.equals(this.clientId, authOAuth.clientId) &&
Objects.equals(this.clientSecret, authOAuth.clientSecret) &&
Objects.equals(this.scope, authOAuth.scope)
);
}
@Override
public int hashCode() {
return Objects.hash(url, clientId, clientSecret, scope);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AuthOAuth {\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" clientSecret: ").append(toIndentedString(clientSecret)).append("\n");
sb.append(" scope: ").append(toIndentedString(scope)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy