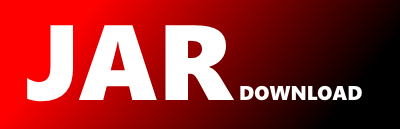
com.algolia.model.ingestion.Destination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Destinations are Algolia resources like indices or event streams. */
public class Destination {
@JsonProperty("destinationID")
private String destinationID;
@JsonProperty("type")
private DestinationType type;
@JsonProperty("name")
private String name;
@JsonProperty("input")
private DestinationInput input;
@JsonProperty("createdAt")
private String createdAt;
@JsonProperty("updatedAt")
private String updatedAt;
@JsonProperty("authenticationID")
private String authenticationID;
@JsonProperty("transformationIDs")
private List transformationIDs;
public Destination setDestinationID(String destinationID) {
this.destinationID = destinationID;
return this;
}
/** Universally unique identifier (UUID) of a destination resource. */
@javax.annotation.Nonnull
public String getDestinationID() {
return destinationID;
}
public Destination setType(DestinationType type) {
this.type = type;
return this;
}
/** Get type */
@javax.annotation.Nonnull
public DestinationType getType() {
return type;
}
public Destination setName(String name) {
this.name = name;
return this;
}
/** Descriptive name for the resource. */
@javax.annotation.Nonnull
public String getName() {
return name;
}
public Destination setInput(DestinationInput input) {
this.input = input;
return this;
}
/** Get input */
@javax.annotation.Nonnull
public DestinationInput getInput() {
return input;
}
public Destination setCreatedAt(String createdAt) {
this.createdAt = createdAt;
return this;
}
/** Date of creation in RFC 3339 format. */
@javax.annotation.Nonnull
public String getCreatedAt() {
return createdAt;
}
public Destination setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/** Date of last update in RFC 3339 format. */
@javax.annotation.Nullable
public String getUpdatedAt() {
return updatedAt;
}
public Destination setAuthenticationID(String authenticationID) {
this.authenticationID = authenticationID;
return this;
}
/** Universally unique identifier (UUID) of an authentication resource. */
@javax.annotation.Nullable
public String getAuthenticationID() {
return authenticationID;
}
public Destination setTransformationIDs(List transformationIDs) {
this.transformationIDs = transformationIDs;
return this;
}
public Destination addTransformationIDs(String transformationIDsItem) {
if (this.transformationIDs == null) {
this.transformationIDs = new ArrayList<>();
}
this.transformationIDs.add(transformationIDsItem);
return this;
}
/** Get transformationIDs */
@javax.annotation.Nullable
public List getTransformationIDs() {
return transformationIDs;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Destination destination = (Destination) o;
return (
Objects.equals(this.destinationID, destination.destinationID) &&
Objects.equals(this.type, destination.type) &&
Objects.equals(this.name, destination.name) &&
Objects.equals(this.input, destination.input) &&
Objects.equals(this.createdAt, destination.createdAt) &&
Objects.equals(this.updatedAt, destination.updatedAt) &&
Objects.equals(this.authenticationID, destination.authenticationID) &&
Objects.equals(this.transformationIDs, destination.transformationIDs)
);
}
@Override
public int hashCode() {
return Objects.hash(destinationID, type, name, input, createdAt, updatedAt, authenticationID, transformationIDs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Destination {\n");
sb.append(" destinationID: ").append(toIndentedString(destinationID)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" authenticationID: ").append(toIndentedString(authenticationID)).append("\n");
sb.append(" transformationIDs: ").append(toIndentedString(transformationIDs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy