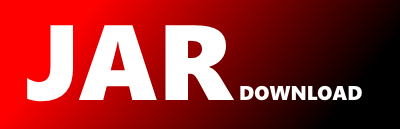
com.algolia.model.ingestion.ShopifyMarket Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Represents a market in Shopify. */
public class ShopifyMarket {
@JsonProperty("countries")
private List countries = new ArrayList<>();
@JsonProperty("currencies")
private List currencies = new ArrayList<>();
@JsonProperty("locales")
private List locales = new ArrayList<>();
public ShopifyMarket setCountries(List countries) {
this.countries = countries;
return this;
}
public ShopifyMarket addCountries(String countriesItem) {
this.countries.add(countriesItem);
return this;
}
/** Get countries */
@javax.annotation.Nonnull
public List getCountries() {
return countries;
}
public ShopifyMarket setCurrencies(List currencies) {
this.currencies = currencies;
return this;
}
public ShopifyMarket addCurrencies(String currenciesItem) {
this.currencies.add(currenciesItem);
return this;
}
/** Get currencies */
@javax.annotation.Nonnull
public List getCurrencies() {
return currencies;
}
public ShopifyMarket setLocales(List locales) {
this.locales = locales;
return this;
}
public ShopifyMarket addLocales(String localesItem) {
this.locales.add(localesItem);
return this;
}
/** Get locales */
@javax.annotation.Nonnull
public List getLocales() {
return locales;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ShopifyMarket shopifyMarket = (ShopifyMarket) o;
return (
Objects.equals(this.countries, shopifyMarket.countries) &&
Objects.equals(this.currencies, shopifyMarket.currencies) &&
Objects.equals(this.locales, shopifyMarket.locales)
);
}
@Override
public int hashCode() {
return Objects.hash(countries, currencies, locales);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ShopifyMarket {\n");
sb.append(" countries: ").append(toIndentedString(countries)).append("\n");
sb.append(" currencies: ").append(toIndentedString(currencies)).append("\n");
sb.append(" locales: ").append(toIndentedString(locales)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy