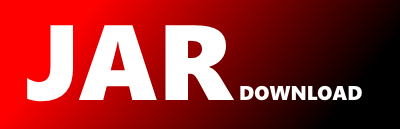
com.algolia.model.ingestion.SourceBigCommerce Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SourceBigCommerce */
@JsonDeserialize(as = SourceBigCommerce.class)
public class SourceBigCommerce implements SourceInput {
@JsonProperty("storeHash")
private String storeHash;
@JsonProperty("channel")
private BigCommerceChannel channel;
@JsonProperty("customFields")
private List customFields;
@JsonProperty("productMetafields")
private List productMetafields;
@JsonProperty("variantMetafields")
private List variantMetafields;
public SourceBigCommerce setStoreHash(String storeHash) {
this.storeHash = storeHash;
return this;
}
/** Store hash identifying your BigCommerce store. */
@javax.annotation.Nonnull
public String getStoreHash() {
return storeHash;
}
public SourceBigCommerce setChannel(BigCommerceChannel channel) {
this.channel = channel;
return this;
}
/** Get channel */
@javax.annotation.Nullable
public BigCommerceChannel getChannel() {
return channel;
}
public SourceBigCommerce setCustomFields(List customFields) {
this.customFields = customFields;
return this;
}
public SourceBigCommerce addCustomFields(String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new ArrayList<>();
}
this.customFields.add(customFieldsItem);
return this;
}
/** Get customFields */
@javax.annotation.Nullable
public List getCustomFields() {
return customFields;
}
public SourceBigCommerce setProductMetafields(List productMetafields) {
this.productMetafields = productMetafields;
return this;
}
public SourceBigCommerce addProductMetafields(BigCommerceMetafield productMetafieldsItem) {
if (this.productMetafields == null) {
this.productMetafields = new ArrayList<>();
}
this.productMetafields.add(productMetafieldsItem);
return this;
}
/** Get productMetafields */
@javax.annotation.Nullable
public List getProductMetafields() {
return productMetafields;
}
public SourceBigCommerce setVariantMetafields(List variantMetafields) {
this.variantMetafields = variantMetafields;
return this;
}
public SourceBigCommerce addVariantMetafields(BigCommerceMetafield variantMetafieldsItem) {
if (this.variantMetafields == null) {
this.variantMetafields = new ArrayList<>();
}
this.variantMetafields.add(variantMetafieldsItem);
return this;
}
/** Get variantMetafields */
@javax.annotation.Nullable
public List getVariantMetafields() {
return variantMetafields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceBigCommerce sourceBigCommerce = (SourceBigCommerce) o;
return (
Objects.equals(this.storeHash, sourceBigCommerce.storeHash) &&
Objects.equals(this.channel, sourceBigCommerce.channel) &&
Objects.equals(this.customFields, sourceBigCommerce.customFields) &&
Objects.equals(this.productMetafields, sourceBigCommerce.productMetafields) &&
Objects.equals(this.variantMetafields, sourceBigCommerce.variantMetafields)
);
}
@Override
public int hashCode() {
return Objects.hash(storeHash, channel, customFields, productMetafields, variantMetafields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SourceBigCommerce {\n");
sb.append(" storeHash: ").append(toIndentedString(storeHash)).append("\n");
sb.append(" channel: ").append(toIndentedString(channel)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" productMetafields: ").append(toIndentedString(productMetafields)).append("\n");
sb.append(" variantMetafields: ").append(toIndentedString(variantMetafields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy