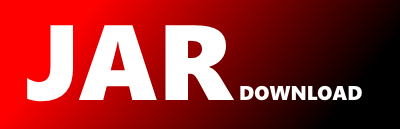
com.algolia.model.ingestion.SourceBigQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** SourceBigQuery */
@JsonDeserialize(as = SourceBigQuery.class)
public class SourceBigQuery implements SourceInput, SourceUpdateInput {
@JsonProperty("projectID")
private String projectID;
@JsonProperty("datasetID")
private String datasetID;
@JsonProperty("dataType")
private BigQueryDataType dataType;
@JsonProperty("table")
private String table;
@JsonProperty("tablePrefix")
private String tablePrefix;
@JsonProperty("customSQLRequest")
private String customSQLRequest;
@JsonProperty("uniqueIDColumn")
private String uniqueIDColumn;
public SourceBigQuery setProjectID(String projectID) {
this.projectID = projectID;
return this;
}
/** Project ID of the BigQuery source. */
@javax.annotation.Nonnull
public String getProjectID() {
return projectID;
}
public SourceBigQuery setDatasetID(String datasetID) {
this.datasetID = datasetID;
return this;
}
/** Dataset ID of the BigQuery source. */
@javax.annotation.Nonnull
public String getDatasetID() {
return datasetID;
}
public SourceBigQuery setDataType(BigQueryDataType dataType) {
this.dataType = dataType;
return this;
}
/** Get dataType */
@javax.annotation.Nullable
public BigQueryDataType getDataType() {
return dataType;
}
public SourceBigQuery setTable(String table) {
this.table = table;
return this;
}
/** Table name for the BigQuery export. */
@javax.annotation.Nullable
public String getTable() {
return table;
}
public SourceBigQuery setTablePrefix(String tablePrefix) {
this.tablePrefix = tablePrefix;
return this;
}
/** Table prefix for a Google Analytics 4 data export to BigQuery. */
@javax.annotation.Nullable
public String getTablePrefix() {
return tablePrefix;
}
public SourceBigQuery setCustomSQLRequest(String customSQLRequest) {
this.customSQLRequest = customSQLRequest;
return this;
}
/** Custom SQL request to extract data from the BigQuery table. */
@javax.annotation.Nullable
public String getCustomSQLRequest() {
return customSQLRequest;
}
public SourceBigQuery setUniqueIDColumn(String uniqueIDColumn) {
this.uniqueIDColumn = uniqueIDColumn;
return this;
}
/** Name of a column that contains a unique ID which will be used as `objectID` in Algolia. */
@javax.annotation.Nullable
public String getUniqueIDColumn() {
return uniqueIDColumn;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceBigQuery sourceBigQuery = (SourceBigQuery) o;
return (
Objects.equals(this.projectID, sourceBigQuery.projectID) &&
Objects.equals(this.datasetID, sourceBigQuery.datasetID) &&
Objects.equals(this.dataType, sourceBigQuery.dataType) &&
Objects.equals(this.table, sourceBigQuery.table) &&
Objects.equals(this.tablePrefix, sourceBigQuery.tablePrefix) &&
Objects.equals(this.customSQLRequest, sourceBigQuery.customSQLRequest) &&
Objects.equals(this.uniqueIDColumn, sourceBigQuery.uniqueIDColumn)
);
}
@Override
public int hashCode() {
return Objects.hash(projectID, datasetID, dataType, table, tablePrefix, customSQLRequest, uniqueIDColumn);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SourceBigQuery {\n");
sb.append(" projectID: ").append(toIndentedString(projectID)).append("\n");
sb.append(" datasetID: ").append(toIndentedString(datasetID)).append("\n");
sb.append(" dataType: ").append(toIndentedString(dataType)).append("\n");
sb.append(" table: ").append(toIndentedString(table)).append("\n");
sb.append(" tablePrefix: ").append(toIndentedString(tablePrefix)).append("\n");
sb.append(" customSQLRequest: ").append(toIndentedString(customSQLRequest)).append("\n");
sb.append(" uniqueIDColumn: ").append(toIndentedString(uniqueIDColumn)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy