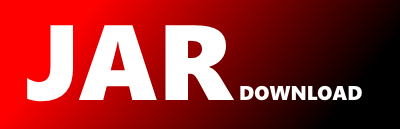
com.algolia.model.ingestion.SourceCommercetools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SourceCommercetools */
@JsonDeserialize(as = SourceCommercetools.class)
public class SourceCommercetools implements SourceInput {
@JsonProperty("storeKeys")
private List storeKeys;
@JsonProperty("locales")
private List locales;
@JsonProperty("url")
private String url;
@JsonProperty("projectKey")
private String projectKey;
@JsonProperty("fallbackIsInStockValue")
private Boolean fallbackIsInStockValue;
@JsonProperty("customFields")
private CommercetoolsCustomFields customFields;
public SourceCommercetools setStoreKeys(List storeKeys) {
this.storeKeys = storeKeys;
return this;
}
public SourceCommercetools addStoreKeys(String storeKeysItem) {
if (this.storeKeys == null) {
this.storeKeys = new ArrayList<>();
}
this.storeKeys.add(storeKeysItem);
return this;
}
/** Get storeKeys */
@javax.annotation.Nullable
public List getStoreKeys() {
return storeKeys;
}
public SourceCommercetools setLocales(List locales) {
this.locales = locales;
return this;
}
public SourceCommercetools addLocales(String localesItem) {
if (this.locales == null) {
this.locales = new ArrayList<>();
}
this.locales.add(localesItem);
return this;
}
/** Locales for your commercetools stores. */
@javax.annotation.Nullable
public List getLocales() {
return locales;
}
public SourceCommercetools setUrl(String url) {
this.url = url;
return this;
}
/** Get url */
@javax.annotation.Nonnull
public String getUrl() {
return url;
}
public SourceCommercetools setProjectKey(String projectKey) {
this.projectKey = projectKey;
return this;
}
/** Get projectKey */
@javax.annotation.Nonnull
public String getProjectKey() {
return projectKey;
}
public SourceCommercetools setFallbackIsInStockValue(Boolean fallbackIsInStockValue) {
this.fallbackIsInStockValue = fallbackIsInStockValue;
return this;
}
/**
* Whether a fallback value is stored in the Algolia record if there's no inventory information
* about the product.
*/
@javax.annotation.Nullable
public Boolean getFallbackIsInStockValue() {
return fallbackIsInStockValue;
}
public SourceCommercetools setCustomFields(CommercetoolsCustomFields customFields) {
this.customFields = customFields;
return this;
}
/** Get customFields */
@javax.annotation.Nullable
public CommercetoolsCustomFields getCustomFields() {
return customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceCommercetools sourceCommercetools = (SourceCommercetools) o;
return (
Objects.equals(this.storeKeys, sourceCommercetools.storeKeys) &&
Objects.equals(this.locales, sourceCommercetools.locales) &&
Objects.equals(this.url, sourceCommercetools.url) &&
Objects.equals(this.projectKey, sourceCommercetools.projectKey) &&
Objects.equals(this.fallbackIsInStockValue, sourceCommercetools.fallbackIsInStockValue) &&
Objects.equals(this.customFields, sourceCommercetools.customFields)
);
}
@Override
public int hashCode() {
return Objects.hash(storeKeys, locales, url, projectKey, fallbackIsInStockValue, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SourceCommercetools {\n");
sb.append(" storeKeys: ").append(toIndentedString(storeKeys)).append("\n");
sb.append(" locales: ").append(toIndentedString(locales)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" projectKey: ").append(toIndentedString(projectKey)).append("\n");
sb.append(" fallbackIsInStockValue: ").append(toIndentedString(fallbackIsInStockValue)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy