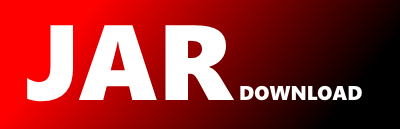
com.algolia.model.ingestion.SourceDocker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** SourceDocker */
@JsonDeserialize(as = SourceDocker.class)
public class SourceDocker implements SourceInput {
@JsonProperty("imageType")
private DockerImageType imageType;
@JsonProperty("registry")
private DockerRegistry registry;
@JsonProperty("image")
private String image;
@JsonProperty("version")
private String version;
@JsonProperty("configuration")
private Object configuration;
public SourceDocker setImageType(DockerImageType imageType) {
this.imageType = imageType;
return this;
}
/** Get imageType */
@javax.annotation.Nonnull
public DockerImageType getImageType() {
return imageType;
}
public SourceDocker setRegistry(DockerRegistry registry) {
this.registry = registry;
return this;
}
/** Get registry */
@javax.annotation.Nonnull
public DockerRegistry getRegistry() {
return registry;
}
public SourceDocker setImage(String image) {
this.image = image;
return this;
}
/** Docker image name. */
@javax.annotation.Nonnull
public String getImage() {
return image;
}
public SourceDocker setVersion(String version) {
this.version = version;
return this;
}
/** Docker image version. */
@javax.annotation.Nullable
public String getVersion() {
return version;
}
public SourceDocker setConfiguration(Object configuration) {
this.configuration = configuration;
return this;
}
/** Configuration of the spec. */
@javax.annotation.Nonnull
public Object getConfiguration() {
return configuration;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceDocker sourceDocker = (SourceDocker) o;
return (
Objects.equals(this.imageType, sourceDocker.imageType) &&
Objects.equals(this.registry, sourceDocker.registry) &&
Objects.equals(this.image, sourceDocker.image) &&
Objects.equals(this.version, sourceDocker.version) &&
Objects.equals(this.configuration, sourceDocker.configuration)
);
}
@Override
public int hashCode() {
return Objects.hash(imageType, registry, image, version, configuration);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SourceDocker {\n");
sb.append(" imageType: ").append(toIndentedString(imageType)).append("\n");
sb.append(" registry: ").append(toIndentedString(registry)).append("\n");
sb.append(" image: ").append(toIndentedString(image)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" configuration: ").append(toIndentedString(configuration)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy