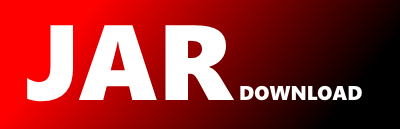
com.algolia.model.ingestion.SourceUpdateCommercetools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SourceUpdateCommercetools */
@JsonDeserialize(as = SourceUpdateCommercetools.class)
public class SourceUpdateCommercetools implements SourceUpdateInput {
@JsonProperty("storeKeys")
private List storeKeys;
@JsonProperty("locales")
private List locales;
@JsonProperty("url")
private String url;
@JsonProperty("fallbackIsInStockValue")
private Boolean fallbackIsInStockValue;
@JsonProperty("customFields")
private CommercetoolsCustomFields customFields;
public SourceUpdateCommercetools setStoreKeys(List storeKeys) {
this.storeKeys = storeKeys;
return this;
}
public SourceUpdateCommercetools addStoreKeys(String storeKeysItem) {
if (this.storeKeys == null) {
this.storeKeys = new ArrayList<>();
}
this.storeKeys.add(storeKeysItem);
return this;
}
/** Get storeKeys */
@javax.annotation.Nullable
public List getStoreKeys() {
return storeKeys;
}
public SourceUpdateCommercetools setLocales(List locales) {
this.locales = locales;
return this;
}
public SourceUpdateCommercetools addLocales(String localesItem) {
if (this.locales == null) {
this.locales = new ArrayList<>();
}
this.locales.add(localesItem);
return this;
}
/** Locales for your commercetools stores. */
@javax.annotation.Nullable
public List getLocales() {
return locales;
}
public SourceUpdateCommercetools setUrl(String url) {
this.url = url;
return this;
}
/** Get url */
@javax.annotation.Nullable
public String getUrl() {
return url;
}
public SourceUpdateCommercetools setFallbackIsInStockValue(Boolean fallbackIsInStockValue) {
this.fallbackIsInStockValue = fallbackIsInStockValue;
return this;
}
/**
* Whether a fallback value is stored in the Algolia record if there's no inventory information
* about the product.
*/
@javax.annotation.Nullable
public Boolean getFallbackIsInStockValue() {
return fallbackIsInStockValue;
}
public SourceUpdateCommercetools setCustomFields(CommercetoolsCustomFields customFields) {
this.customFields = customFields;
return this;
}
/** Get customFields */
@javax.annotation.Nullable
public CommercetoolsCustomFields getCustomFields() {
return customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceUpdateCommercetools sourceUpdateCommercetools = (SourceUpdateCommercetools) o;
return (
Objects.equals(this.storeKeys, sourceUpdateCommercetools.storeKeys) &&
Objects.equals(this.locales, sourceUpdateCommercetools.locales) &&
Objects.equals(this.url, sourceUpdateCommercetools.url) &&
Objects.equals(this.fallbackIsInStockValue, sourceUpdateCommercetools.fallbackIsInStockValue) &&
Objects.equals(this.customFields, sourceUpdateCommercetools.customFields)
);
}
@Override
public int hashCode() {
return Objects.hash(storeKeys, locales, url, fallbackIsInStockValue, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SourceUpdateCommercetools {\n");
sb.append(" storeKeys: ").append(toIndentedString(storeKeys)).append("\n");
sb.append(" locales: ").append(toIndentedString(locales)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" fallbackIsInStockValue: ").append(toIndentedString(fallbackIsInStockValue)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy