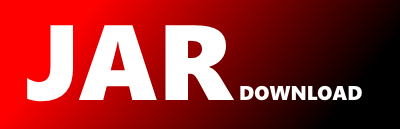
com.algolia.model.ingestion.TaskCreate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** API request body for creating a task. */
public class TaskCreate {
@JsonProperty("sourceID")
private String sourceID;
@JsonProperty("destinationID")
private String destinationID;
@JsonProperty("action")
private ActionType action;
@JsonProperty("cron")
private String cron;
@JsonProperty("enabled")
private Boolean enabled;
@JsonProperty("failureThreshold")
private Integer failureThreshold;
@JsonProperty("input")
private TaskInput input;
@JsonProperty("cursor")
private String cursor;
public TaskCreate setSourceID(String sourceID) {
this.sourceID = sourceID;
return this;
}
/** Universally uniqud identifier (UUID) of a source. */
@javax.annotation.Nonnull
public String getSourceID() {
return sourceID;
}
public TaskCreate setDestinationID(String destinationID) {
this.destinationID = destinationID;
return this;
}
/** Universally unique identifier (UUID) of a destination resource. */
@javax.annotation.Nonnull
public String getDestinationID() {
return destinationID;
}
public TaskCreate setAction(ActionType action) {
this.action = action;
return this;
}
/** Get action */
@javax.annotation.Nonnull
public ActionType getAction() {
return action;
}
public TaskCreate setCron(String cron) {
this.cron = cron;
return this;
}
/** Cron expression for the task's schedule. */
@javax.annotation.Nullable
public String getCron() {
return cron;
}
public TaskCreate setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/** Whether the task is enabled. */
@javax.annotation.Nullable
public Boolean getEnabled() {
return enabled;
}
public TaskCreate setFailureThreshold(Integer failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
/**
* Maximum accepted percentage of failures for a task run to finish successfully. minimum: 0
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getFailureThreshold() {
return failureThreshold;
}
public TaskCreate setInput(TaskInput input) {
this.input = input;
return this;
}
/** Get input */
@javax.annotation.Nullable
public TaskInput getInput() {
return input;
}
public TaskCreate setCursor(String cursor) {
this.cursor = cursor;
return this;
}
/** Date of the last cursor in RFC 3339 format. */
@javax.annotation.Nullable
public String getCursor() {
return cursor;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskCreate taskCreate = (TaskCreate) o;
return (
Objects.equals(this.sourceID, taskCreate.sourceID) &&
Objects.equals(this.destinationID, taskCreate.destinationID) &&
Objects.equals(this.action, taskCreate.action) &&
Objects.equals(this.cron, taskCreate.cron) &&
Objects.equals(this.enabled, taskCreate.enabled) &&
Objects.equals(this.failureThreshold, taskCreate.failureThreshold) &&
Objects.equals(this.input, taskCreate.input) &&
Objects.equals(this.cursor, taskCreate.cursor)
);
}
@Override
public int hashCode() {
return Objects.hash(sourceID, destinationID, action, cron, enabled, failureThreshold, input, cursor);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskCreate {\n");
sb.append(" sourceID: ").append(toIndentedString(sourceID)).append("\n");
sb.append(" destinationID: ").append(toIndentedString(destinationID)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" cron: ").append(toIndentedString(cron)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" failureThreshold: ").append(toIndentedString(failureThreshold)).append("\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" cursor: ").append(toIndentedString(cursor)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy