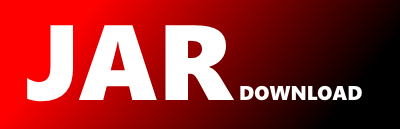
com.algolia.model.ingestion.TaskV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/**
* The V1 task object, please use methods and types that don't contain the V1 suffix.
*
* @deprecated
*/
@Deprecated
public class TaskV1 {
@JsonProperty("taskID")
private String taskID;
@JsonProperty("sourceID")
private String sourceID;
@JsonProperty("destinationID")
private String destinationID;
@JsonProperty("trigger")
private Trigger trigger;
@JsonProperty("input")
private TaskInput input;
@JsonProperty("enabled")
private Boolean enabled;
@JsonProperty("failureThreshold")
private Integer failureThreshold;
@JsonProperty("action")
private ActionType action;
@JsonProperty("cursor")
private String cursor;
@JsonProperty("createdAt")
private String createdAt;
@JsonProperty("updatedAt")
private String updatedAt;
public TaskV1 setTaskID(String taskID) {
this.taskID = taskID;
return this;
}
/** Universally unique identifier (UUID) of a task. */
@javax.annotation.Nonnull
public String getTaskID() {
return taskID;
}
public TaskV1 setSourceID(String sourceID) {
this.sourceID = sourceID;
return this;
}
/** Universally uniqud identifier (UUID) of a source. */
@javax.annotation.Nonnull
public String getSourceID() {
return sourceID;
}
public TaskV1 setDestinationID(String destinationID) {
this.destinationID = destinationID;
return this;
}
/** Universally unique identifier (UUID) of a destination resource. */
@javax.annotation.Nonnull
public String getDestinationID() {
return destinationID;
}
public TaskV1 setTrigger(Trigger trigger) {
this.trigger = trigger;
return this;
}
/** Get trigger */
@javax.annotation.Nonnull
public Trigger getTrigger() {
return trigger;
}
public TaskV1 setInput(TaskInput input) {
this.input = input;
return this;
}
/** Get input */
@javax.annotation.Nullable
public TaskInput getInput() {
return input;
}
public TaskV1 setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/** Whether the task is enabled. */
@javax.annotation.Nonnull
public Boolean getEnabled() {
return enabled;
}
public TaskV1 setFailureThreshold(Integer failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
/**
* Maximum accepted percentage of failures for a task run to finish successfully. minimum: 0
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getFailureThreshold() {
return failureThreshold;
}
public TaskV1 setAction(ActionType action) {
this.action = action;
return this;
}
/** Get action */
@javax.annotation.Nullable
public ActionType getAction() {
return action;
}
public TaskV1 setCursor(String cursor) {
this.cursor = cursor;
return this;
}
/** Date of the last cursor in RFC 3339 format. */
@javax.annotation.Nullable
public String getCursor() {
return cursor;
}
public TaskV1 setCreatedAt(String createdAt) {
this.createdAt = createdAt;
return this;
}
/** Date of creation in RFC 3339 format. */
@javax.annotation.Nonnull
public String getCreatedAt() {
return createdAt;
}
public TaskV1 setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/** Date of last update in RFC 3339 format. */
@javax.annotation.Nullable
public String getUpdatedAt() {
return updatedAt;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskV1 taskV1 = (TaskV1) o;
return (
Objects.equals(this.taskID, taskV1.taskID) &&
Objects.equals(this.sourceID, taskV1.sourceID) &&
Objects.equals(this.destinationID, taskV1.destinationID) &&
Objects.equals(this.trigger, taskV1.trigger) &&
Objects.equals(this.input, taskV1.input) &&
Objects.equals(this.enabled, taskV1.enabled) &&
Objects.equals(this.failureThreshold, taskV1.failureThreshold) &&
Objects.equals(this.action, taskV1.action) &&
Objects.equals(this.cursor, taskV1.cursor) &&
Objects.equals(this.createdAt, taskV1.createdAt) &&
Objects.equals(this.updatedAt, taskV1.updatedAt)
);
}
@Override
public int hashCode() {
return Objects.hash(taskID, sourceID, destinationID, trigger, input, enabled, failureThreshold, action, cursor, createdAt, updatedAt);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskV1 {\n");
sb.append(" taskID: ").append(toIndentedString(taskID)).append("\n");
sb.append(" sourceID: ").append(toIndentedString(sourceID)).append("\n");
sb.append(" destinationID: ").append(toIndentedString(destinationID)).append("\n");
sb.append(" trigger: ").append(toIndentedString(trigger)).append("\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" failureThreshold: ").append(toIndentedString(failureThreshold)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" cursor: ").append(toIndentedString(cursor)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy