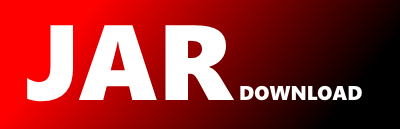
com.algolia.model.insights.ConvertedObjectIDs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.insights;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Use this event to track when users convert on items unrelated to a previous Algolia request. For
* example, if you don't use Algolia to build your category pages, use this event. To track
* conversion events related to Algolia requests, use the \"Converted object IDs after search\"
* event.
*/
@JsonDeserialize(as = ConvertedObjectIDs.class)
public class ConvertedObjectIDs implements EventsItems {
@JsonProperty("eventName")
private String eventName;
@JsonProperty("eventType")
private ConversionEvent eventType;
@JsonProperty("index")
private String index;
@JsonProperty("objectIDs")
private List objectIDs = new ArrayList<>();
@JsonProperty("userToken")
private String userToken;
@JsonProperty("authenticatedUserToken")
private String authenticatedUserToken;
@JsonProperty("timestamp")
private Long timestamp;
public ConvertedObjectIDs setEventName(String eventName) {
this.eventName = eventName;
return this;
}
/**
* Event name, up to 64 ASCII characters. Consider naming events consistently—for example, by
* adopting Segment's
* [object-action](https://segment.com/academy/collecting-data/naming-conventions-for-clean-data/#the-object-action-framework)
* framework.
*/
@javax.annotation.Nonnull
public String getEventName() {
return eventName;
}
public ConvertedObjectIDs setEventType(ConversionEvent eventType) {
this.eventType = eventType;
return this;
}
/** Get eventType */
@javax.annotation.Nonnull
public ConversionEvent getEventType() {
return eventType;
}
public ConvertedObjectIDs setIndex(String index) {
this.index = index;
return this;
}
/** Index name (case-sensitive) to which the event's items belong. */
@javax.annotation.Nonnull
public String getIndex() {
return index;
}
public ConvertedObjectIDs setObjectIDs(List objectIDs) {
this.objectIDs = objectIDs;
return this;
}
public ConvertedObjectIDs addObjectIDs(String objectIDsItem) {
this.objectIDs.add(objectIDsItem);
return this;
}
/** Object IDs of the records that are part of the event. */
@javax.annotation.Nonnull
public List getObjectIDs() {
return objectIDs;
}
public ConvertedObjectIDs setUserToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* Anonymous or pseudonymous user identifier. Don't use personally identifiable information in
* user tokens. For more information, see [User
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/).
*/
@javax.annotation.Nonnull
public String getUserToken() {
return userToken;
}
public ConvertedObjectIDs setAuthenticatedUserToken(String authenticatedUserToken) {
this.authenticatedUserToken = authenticatedUserToken;
return this;
}
/**
* Identifier for authenticated users. When the user signs in, you can get an identifier from your
* system and send it as `authenticatedUserToken`. This lets you keep using the `userToken` from
* before the user signed in, while providing a reliable way to identify users across sessions.
* Don't use personally identifiable information in user tokens. For more information, see [User
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/).
*/
@javax.annotation.Nullable
public String getAuthenticatedUserToken() {
return authenticatedUserToken;
}
public ConvertedObjectIDs setTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Timestamp of the event, measured in milliseconds since the Unix epoch. By default, the Insights
* API uses the time it receives an event as its timestamp.
*/
@javax.annotation.Nullable
public Long getTimestamp() {
return timestamp;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConvertedObjectIDs convertedObjectIDs = (ConvertedObjectIDs) o;
return (
Objects.equals(this.eventName, convertedObjectIDs.eventName) &&
Objects.equals(this.eventType, convertedObjectIDs.eventType) &&
Objects.equals(this.index, convertedObjectIDs.index) &&
Objects.equals(this.objectIDs, convertedObjectIDs.objectIDs) &&
Objects.equals(this.userToken, convertedObjectIDs.userToken) &&
Objects.equals(this.authenticatedUserToken, convertedObjectIDs.authenticatedUserToken) &&
Objects.equals(this.timestamp, convertedObjectIDs.timestamp)
);
}
@Override
public int hashCode() {
return Objects.hash(eventName, eventType, index, objectIDs, userToken, authenticatedUserToken, timestamp);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConvertedObjectIDs {\n");
sb.append(" eventName: ").append(toIndentedString(eventName)).append("\n");
sb.append(" eventType: ").append(toIndentedString(eventType)).append("\n");
sb.append(" index: ").append(toIndentedString(index)).append("\n");
sb.append(" objectIDs: ").append(toIndentedString(objectIDs)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append(" authenticatedUserToken: ").append(toIndentedString(authenticatedUserToken)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy