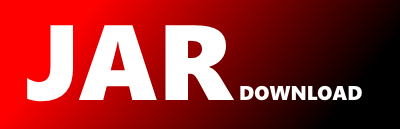
com.algolia.model.monitoring.Metrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.monitoring;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/** Metrics */
public class Metrics {
@JsonProperty("cpu_usage")
private Map> cpuUsage;
@JsonProperty("ram_indexing_usage")
private Map> ramIndexingUsage;
@JsonProperty("ram_search_usage")
private Map> ramSearchUsage;
@JsonProperty("ssd_usage")
private Map> ssdUsage;
@JsonProperty("avg_build_time")
private Map> avgBuildTime;
public Metrics setCpuUsage(Map> cpuUsage) {
this.cpuUsage = cpuUsage;
return this;
}
public Metrics putCpuUsage(String key, List cpuUsageItem) {
if (this.cpuUsage == null) {
this.cpuUsage = new HashMap<>();
}
this.cpuUsage.put(key, cpuUsageItem);
return this;
}
/** CPU idleness in %. */
@javax.annotation.Nullable
public Map> getCpuUsage() {
return cpuUsage;
}
public Metrics setRamIndexingUsage(Map> ramIndexingUsage) {
this.ramIndexingUsage = ramIndexingUsage;
return this;
}
public Metrics putRamIndexingUsage(String key, List ramIndexingUsageItem) {
if (this.ramIndexingUsage == null) {
this.ramIndexingUsage = new HashMap<>();
}
this.ramIndexingUsage.put(key, ramIndexingUsageItem);
return this;
}
/** RAM used for indexing in MB. */
@javax.annotation.Nullable
public Map> getRamIndexingUsage() {
return ramIndexingUsage;
}
public Metrics setRamSearchUsage(Map> ramSearchUsage) {
this.ramSearchUsage = ramSearchUsage;
return this;
}
public Metrics putRamSearchUsage(String key, List ramSearchUsageItem) {
if (this.ramSearchUsage == null) {
this.ramSearchUsage = new HashMap<>();
}
this.ramSearchUsage.put(key, ramSearchUsageItem);
return this;
}
/** RAM used for search in MB. */
@javax.annotation.Nullable
public Map> getRamSearchUsage() {
return ramSearchUsage;
}
public Metrics setSsdUsage(Map> ssdUsage) {
this.ssdUsage = ssdUsage;
return this;
}
public Metrics putSsdUsage(String key, List ssdUsageItem) {
if (this.ssdUsage == null) {
this.ssdUsage = new HashMap<>();
}
this.ssdUsage.put(key, ssdUsageItem);
return this;
}
/**
* Solid-state disk (SSD) usage expressed as % of RAM. 0% means no SSD usage. A value of 50%
* indicates 32 GB SSD usage for a machine with 64 RAM.
*/
@javax.annotation.Nullable
public Map> getSsdUsage() {
return ssdUsage;
}
public Metrics setAvgBuildTime(Map> avgBuildTime) {
this.avgBuildTime = avgBuildTime;
return this;
}
public Metrics putAvgBuildTime(String key, List avgBuildTimeItem) {
if (this.avgBuildTime == null) {
this.avgBuildTime = new HashMap<>();
}
this.avgBuildTime.put(key, avgBuildTimeItem);
return this;
}
/** Average build time of the indices in seconds. */
@javax.annotation.Nullable
public Map> getAvgBuildTime() {
return avgBuildTime;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Metrics metrics = (Metrics) o;
return (
Objects.equals(this.cpuUsage, metrics.cpuUsage) &&
Objects.equals(this.ramIndexingUsage, metrics.ramIndexingUsage) &&
Objects.equals(this.ramSearchUsage, metrics.ramSearchUsage) &&
Objects.equals(this.ssdUsage, metrics.ssdUsage) &&
Objects.equals(this.avgBuildTime, metrics.avgBuildTime)
);
}
@Override
public int hashCode() {
return Objects.hash(cpuUsage, ramIndexingUsage, ramSearchUsage, ssdUsage, avgBuildTime);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Metrics {\n");
sb.append(" cpuUsage: ").append(toIndentedString(cpuUsage)).append("\n");
sb.append(" ramIndexingUsage: ").append(toIndentedString(ramIndexingUsage)).append("\n");
sb.append(" ramSearchUsage: ").append(toIndentedString(ramSearchUsage)).append("\n");
sb.append(" ssdUsage: ").append(toIndentedString(ssdUsage)).append("\n");
sb.append(" avgBuildTime: ").append(toIndentedString(avgBuildTime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy