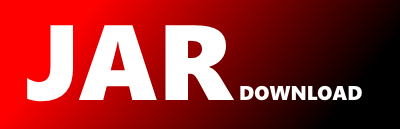
com.algolia.model.recommend.AroundPrecision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.io.IOException;
import java.util.List;
import java.util.logging.Logger;
/**
* Precision of a coordinate-based search in meters to group results with similar distances. The Geo
* ranking criterion considers all matches within the same range of distances to be equal.
*/
@JsonDeserialize(using = AroundPrecision.Deserializer.class)
public interface AroundPrecision {
// AroundPrecision as Integer wrapper.
static AroundPrecision of(Integer value) {
return new IntegerWrapper(value);
}
// AroundPrecision as List wrapper.
static AroundPrecision of(List value) {
return new ListOfRangeWrapper(value);
}
// AroundPrecision as Integer wrapper.
@JsonSerialize(using = IntegerWrapper.Serializer.class)
class IntegerWrapper implements AroundPrecision {
private final Integer value;
IntegerWrapper(Integer value) {
this.value = value;
}
public Integer getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(IntegerWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
// AroundPrecision as List wrapper.
@JsonSerialize(using = ListOfRangeWrapper.Serializer.class)
class ListOfRangeWrapper implements AroundPrecision {
private final List value;
ListOfRangeWrapper(List value) {
this.value = value;
}
public List getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(ListOfRangeWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
class Deserializer extends JsonDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
@Override
public AroundPrecision deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize Integer
if (tree.isInt()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Integer value = parser.readValueAs(Integer.class);
return new AroundPrecision.IntegerWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Integer (error: " + e.getMessage() + ") (type: Integer)");
}
}
// deserialize List
if (tree.isArray()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
List value = parser.readValueAs(new TypeReference>() {});
return new AroundPrecision.ListOfRangeWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf List (error: " + e.getMessage() + ") (type: List)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public AroundPrecision getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "AroundPrecision cannot be null");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy