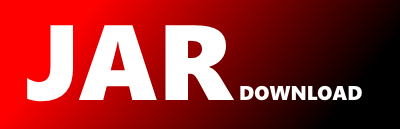
com.algolia.model.recommend.ParamsConsequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Filter or boost recommendations matching a facet filter. */
public class ParamsConsequence {
@JsonProperty("automaticFacetFilters")
private List automaticFacetFilters;
@JsonProperty("filters")
private String filters;
@JsonProperty("optionalFilters")
private List optionalFilters;
public ParamsConsequence setAutomaticFacetFilters(List automaticFacetFilters) {
this.automaticFacetFilters = automaticFacetFilters;
return this;
}
public ParamsConsequence addAutomaticFacetFilters(AutoFacetFilter automaticFacetFiltersItem) {
if (this.automaticFacetFilters == null) {
this.automaticFacetFilters = new ArrayList<>();
}
this.automaticFacetFilters.add(automaticFacetFiltersItem);
return this;
}
/**
* Filter recommendations that match or don't match the same `facet:facet_value` combination as
* the viewed item.
*/
@javax.annotation.Nullable
public List getAutomaticFacetFilters() {
return automaticFacetFilters;
}
public ParamsConsequence setFilters(String filters) {
this.filters = filters;
return this;
}
/**
* Filter expression to only include items that match the filter criteria in the response. You can
* use these filter expressions: - **Numeric filters.** ` `, where `` is
* one of `<`, `<=`, `=`, `!=`, `>`, `>=`. - **Ranges.** `: TO ` where
* `` and `` are the lower and upper limits of the range (inclusive). - **Facet
* filters.** `:` where `` is a facet attribute (case-sensitive) and
* `` a facet value. - **Tag filters.** `_tags:` or just `` (case-sensitive).
* - **Boolean filters.** `: true | false`. You can combine filters with `AND`, `OR`, and
* `NOT` operators with the following restrictions: - You can only combine filters of the same
* type with `OR`. **Not supported:** `facet:value OR num > 3`. - You can't use `NOT` with
* combinations of filters. **Not supported:** `NOT(facet:value OR facet:value)` - You can't
* combine conjunctions (`AND`) with `OR`. **Not supported:** `facet:value OR (facet:value AND
* facet:value)` Use quotes around your filters, if the facet attribute name or facet value has
* spaces, keywords (`OR`, `AND`, `NOT`), or quotes. If a facet attribute is an array, the filter
* matches if it matches at least one element of the array. For more information, see
* [Filters](https://www.algolia.com/doc/guides/managing-results/refine-results/filtering/).
*/
@javax.annotation.Nullable
public String getFilters() {
return filters;
}
public ParamsConsequence setOptionalFilters(List optionalFilters) {
this.optionalFilters = optionalFilters;
return this;
}
public ParamsConsequence addOptionalFilters(String optionalFiltersItem) {
if (this.optionalFilters == null) {
this.optionalFilters = new ArrayList<>();
}
this.optionalFilters.add(optionalFiltersItem);
return this;
}
/**
* Filters to promote or demote records in the search results. Optional filters work like facet
* filters, but they don't exclude records from the search results. Records that match the
* optional filter rank before records that don't match. Matches with higher weights (``)
* rank before matches with lower weights. If you're using a negative filter `facet:-value`,
* matching records rank after records that don't match.
*/
@javax.annotation.Nullable
public List getOptionalFilters() {
return optionalFilters;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ParamsConsequence paramsConsequence = (ParamsConsequence) o;
return (
Objects.equals(this.automaticFacetFilters, paramsConsequence.automaticFacetFilters) &&
Objects.equals(this.filters, paramsConsequence.filters) &&
Objects.equals(this.optionalFilters, paramsConsequence.optionalFilters)
);
}
@Override
public int hashCode() {
return Objects.hash(automaticFacetFilters, filters, optionalFilters);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ParamsConsequence {\n");
sb.append(" automaticFacetFilters: ").append(toIndentedString(automaticFacetFilters)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append(" optionalFilters: ").append(toIndentedString(optionalFilters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy