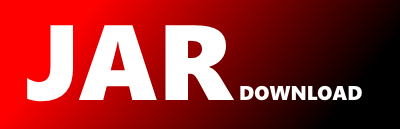
com.algolia.model.recommend.RecommendRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Recommend rule. */
public class RecommendRule {
@JsonProperty("_metadata")
private RuleMetadata metadata;
@JsonProperty("objectID")
private String objectID;
@JsonProperty("condition")
private Condition condition;
@JsonProperty("consequence")
private Consequence consequence;
@JsonProperty("description")
private String description;
@JsonProperty("enabled")
private Boolean enabled;
@JsonProperty("validity")
private List validity;
public RecommendRule setMetadata(RuleMetadata metadata) {
this.metadata = metadata;
return this;
}
/** Get metadata */
@javax.annotation.Nullable
public RuleMetadata getMetadata() {
return metadata;
}
public RecommendRule setObjectID(String objectID) {
this.objectID = objectID;
return this;
}
/** Unique identifier of a rule object. */
@javax.annotation.Nullable
public String getObjectID() {
return objectID;
}
public RecommendRule setCondition(Condition condition) {
this.condition = condition;
return this;
}
/** Get condition */
@javax.annotation.Nullable
public Condition getCondition() {
return condition;
}
public RecommendRule setConsequence(Consequence consequence) {
this.consequence = consequence;
return this;
}
/** Get consequence */
@javax.annotation.Nullable
public Consequence getConsequence() {
return consequence;
}
public RecommendRule setDescription(String description) {
this.description = description;
return this;
}
/**
* Description of the rule's purpose. This can be helpful for display in the Algolia dashboard.
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public RecommendRule setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/** Indicates whether to enable the rule. If it isn't enabled, it isn't applied at query time. */
@javax.annotation.Nullable
public Boolean getEnabled() {
return enabled;
}
public RecommendRule setValidity(List validity) {
this.validity = validity;
return this;
}
public RecommendRule addValidity(TimeRange validityItem) {
if (this.validity == null) {
this.validity = new ArrayList<>();
}
this.validity.add(validityItem);
return this;
}
/** Time periods when the rule is active. */
@javax.annotation.Nullable
public List getValidity() {
return validity;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RecommendRule recommendRule = (RecommendRule) o;
return (
Objects.equals(this.metadata, recommendRule.metadata) &&
Objects.equals(this.objectID, recommendRule.objectID) &&
Objects.equals(this.condition, recommendRule.condition) &&
Objects.equals(this.consequence, recommendRule.consequence) &&
Objects.equals(this.description, recommendRule.description) &&
Objects.equals(this.enabled, recommendRule.enabled) &&
Objects.equals(this.validity, recommendRule.validity)
);
}
@Override
public int hashCode() {
return Objects.hash(metadata, objectID, condition, consequence, description, enabled, validity);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RecommendRule {\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" objectID: ").append(toIndentedString(objectID)).append("\n");
sb.append(" condition: ").append(toIndentedString(condition)).append("\n");
sb.append(" consequence: ").append(toIndentedString(consequence)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" validity: ").append(toIndentedString(validity)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy