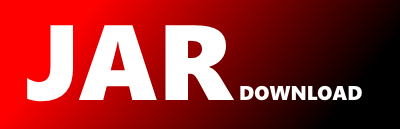
com.algolia.model.recommend.RecommendationsHit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.recommend;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.io.IOException;
import java.util.logging.Logger;
/** RecommendationsHit */
@JsonDeserialize(using = RecommendationsHit.Deserializer.class)
public interface RecommendationsHit {
// RecommendationsHit as RecommendHit wrapper.
static RecommendationsHit of(RecommendHit value) {
return new RecommendHitWrapper(value);
}
// RecommendationsHit as RecommendHit wrapper.
@JsonSerialize(using = RecommendHitWrapper.Serializer.class)
class RecommendHitWrapper implements RecommendationsHit {
private final RecommendHit value;
RecommendHitWrapper(RecommendHit value) {
this.value = value;
}
public RecommendHit getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(RecommendHitWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
class Deserializer extends JsonDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
@Override
public RecommendationsHit deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize TrendingFacetHit
if (tree.isObject() && tree.has("facetName") && tree.has("facetValue")) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
return parser.readValueAs(TrendingFacetHit.class);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf TrendingFacetHit (error: " + e.getMessage() + ") (type: TrendingFacetHit)");
}
}
// deserialize RecommendHit
if (tree.isObject()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
RecommendHit value = parser.readValueAs(RecommendHit.class);
return new RecommendationsHit.RecommendHitWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf RecommendHit (error: " + e.getMessage() + ") (type: RecommendHit)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public RecommendationsHit getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "RecommendationsHit cannot be null");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy