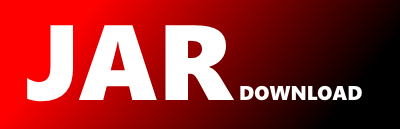
com.algolia.model.search.BuiltInOperationValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.io.IOException;
import java.util.logging.Logger;
/** BuiltInOperationValue */
@JsonDeserialize(using = BuiltInOperationValue.Deserializer.class)
public interface BuiltInOperationValue {
// BuiltInOperationValue as String wrapper.
static BuiltInOperationValue of(String value) {
return new StringWrapper(value);
}
// BuiltInOperationValue as Integer wrapper.
static BuiltInOperationValue of(Integer value) {
return new IntegerWrapper(value);
}
// BuiltInOperationValue as String wrapper.
@JsonSerialize(using = StringWrapper.Serializer.class)
class StringWrapper implements BuiltInOperationValue {
private final String value;
StringWrapper(String value) {
this.value = value;
}
public String getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(StringWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
// BuiltInOperationValue as Integer wrapper.
@JsonSerialize(using = IntegerWrapper.Serializer.class)
class IntegerWrapper implements BuiltInOperationValue {
private final Integer value;
IntegerWrapper(Integer value) {
this.value = value;
}
public Integer getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(IntegerWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
class Deserializer extends JsonDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
@Override
public BuiltInOperationValue deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize String
if (tree.isTextual()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
String value = parser.readValueAs(String.class);
return new BuiltInOperationValue.StringWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf String (error: " + e.getMessage() + ") (type: String)");
}
}
// deserialize Integer
if (tree.isInt()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Integer value = parser.readValueAs(Integer.class);
return new BuiltInOperationValue.IntegerWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Integer (error: " + e.getMessage() + ") (type: Integer)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public BuiltInOperationValue getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "BuiltInOperationValue cannot be null");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy