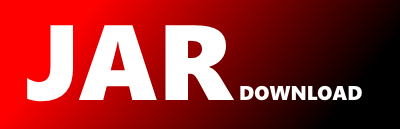
com.algolia.model.search.Condition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** Condition */
public class Condition {
@JsonProperty("pattern")
private String pattern;
@JsonProperty("anchoring")
private Anchoring anchoring;
@JsonProperty("alternatives")
private Boolean alternatives;
@JsonProperty("context")
private String context;
@JsonProperty("filters")
private String filters;
public Condition setPattern(String pattern) {
this.pattern = pattern;
return this;
}
/**
* Query pattern that triggers the rule. You can use either a literal string, or a special pattern
* `{facet:ATTRIBUTE}`, where `ATTRIBUTE` is a facet name. The rule is triggered if the query
* matches the literal string or a value of the specified facet. For example, with `pattern:
* {facet:genre}`, the rule is triggered when users search for a genre, such as \"comedy\".
*/
@javax.annotation.Nullable
public String getPattern() {
return pattern;
}
public Condition setAnchoring(Anchoring anchoring) {
this.anchoring = anchoring;
return this;
}
/** Get anchoring */
@javax.annotation.Nullable
public Anchoring getAnchoring() {
return anchoring;
}
public Condition setAlternatives(Boolean alternatives) {
this.alternatives = alternatives;
return this;
}
/** Whether the pattern should match plurals, synonyms, and typos. */
@javax.annotation.Nullable
public Boolean getAlternatives() {
return alternatives;
}
public Condition setContext(String context) {
this.context = context;
return this;
}
/**
* An additional restriction that only triggers the rule, when the search has the same value as
* `ruleContexts` parameter. For example, if `context: mobile`, the rule is only triggered when
* the search request has a matching `ruleContexts: mobile`. A rule context must only contain
* alphanumeric characters.
*/
@javax.annotation.Nullable
public String getContext() {
return context;
}
public Condition setFilters(String filters) {
this.filters = filters;
return this;
}
/**
* Filters that trigger the rule. You can add add filters using the syntax `facet:value` so that
* the rule is triggered, when the specific filter is selected. You can use `filters` on its own
* or combine it with the `pattern` parameter.
*/
@javax.annotation.Nullable
public String getFilters() {
return filters;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Condition condition = (Condition) o;
return (
Objects.equals(this.pattern, condition.pattern) &&
Objects.equals(this.anchoring, condition.anchoring) &&
Objects.equals(this.alternatives, condition.alternatives) &&
Objects.equals(this.context, condition.context) &&
Objects.equals(this.filters, condition.filters)
);
}
@Override
public int hashCode() {
return Objects.hash(pattern, anchoring, alternatives, context, filters);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Condition {\n");
sb.append(" pattern: ").append(toIndentedString(pattern)).append("\n");
sb.append(" anchoring: ").append(toIndentedString(anchoring)).append("\n");
sb.append(" alternatives: ").append(toIndentedString(alternatives)).append("\n");
sb.append(" context: ").append(toIndentedString(context)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy