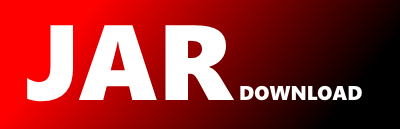
com.algolia.model.search.Consequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Effect of the rule. For more information, see
* [Consequences](https://www.algolia.com/doc/guides/managing-results/rules/rules-overview/#consequences).
*/
public class Consequence {
@JsonProperty("params")
private ConsequenceParams params;
@JsonProperty("promote")
private List promote;
@JsonProperty("filterPromotes")
private Boolean filterPromotes;
@JsonProperty("hide")
private List hide;
@JsonProperty("userData")
private Object userData;
public Consequence setParams(ConsequenceParams params) {
this.params = params;
return this;
}
/** Get params */
@javax.annotation.Nullable
public ConsequenceParams getParams() {
return params;
}
public Consequence setPromote(List promote) {
this.promote = promote;
return this;
}
public Consequence addPromote(Promote promoteItem) {
if (this.promote == null) {
this.promote = new ArrayList<>();
}
this.promote.add(promoteItem);
return this;
}
/**
* Records you want to pin to a specific position in the search results. You can promote up to 300
* records, either individually, or as groups of up to 100 records each.
*/
@javax.annotation.Nullable
public List getPromote() {
return promote;
}
public Consequence setFilterPromotes(Boolean filterPromotes) {
this.filterPromotes = filterPromotes;
return this;
}
/**
* Whether promoted records must match an active filter for the consequence to be applied. This
* ensures that user actions (filtering the search) are given a higher precendence. For example,
* if you promote a record with the `color: red` attribute, and the user filters the search for
* `color: blue`, the \"red\" record won't be shown.
*/
@javax.annotation.Nullable
public Boolean getFilterPromotes() {
return filterPromotes;
}
public Consequence setHide(List hide) {
this.hide = hide;
return this;
}
public Consequence addHide(ConsequenceHide hideItem) {
if (this.hide == null) {
this.hide = new ArrayList<>();
}
this.hide.add(hideItem);
return this;
}
/** Records you want to hide from the search results. */
@javax.annotation.Nullable
public List getHide() {
return hide;
}
public Consequence setUserData(Object userData) {
this.userData = userData;
return this;
}
/**
* A JSON object with custom data that will be appended to the `userData` array in the response.
* This object isn't interpreted by the API and is limited to 1 kB of minified JSON.
*/
@javax.annotation.Nullable
public Object getUserData() {
return userData;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Consequence consequence = (Consequence) o;
return (
Objects.equals(this.params, consequence.params) &&
Objects.equals(this.promote, consequence.promote) &&
Objects.equals(this.filterPromotes, consequence.filterPromotes) &&
Objects.equals(this.hide, consequence.hide) &&
Objects.equals(this.userData, consequence.userData)
);
}
@Override
public int hashCode() {
return Objects.hash(params, promote, filterPromotes, hide, userData);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Consequence {\n");
sb.append(" params: ").append(toIndentedString(params)).append("\n");
sb.append(" promote: ").append(toIndentedString(promote)).append("\n");
sb.append(" filterPromotes: ").append(toIndentedString(filterPromotes)).append("\n");
sb.append(" hide: ").append(toIndentedString(hide)).append("\n");
sb.append(" userData: ").append(toIndentedString(userData)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy