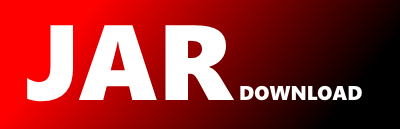
com.algolia.model.search.Distinct Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.io.IOException;
import java.util.logging.Logger;
/**
* Determines how many records of a group are included in the search results. Records with the same
* value for the `attributeForDistinct` attribute are considered a group. The `distinct` setting
* controls how many members of the group are returned. This is useful for [deduplication and
* grouping](https://www.algolia.com/doc/guides/managing-results/refine-results/grouping/#introducing-algolias-distinct-feature).
* The `distinct` setting is ignored if `attributeForDistinct` is not set.
*/
@JsonDeserialize(using = Distinct.Deserializer.class)
public interface Distinct {
// Distinct as Boolean wrapper.
static Distinct of(Boolean value) {
return new BooleanWrapper(value);
}
// Distinct as Integer wrapper.
static Distinct of(Integer value) {
return new IntegerWrapper(value);
}
// Distinct as Boolean wrapper.
@JsonSerialize(using = BooleanWrapper.Serializer.class)
class BooleanWrapper implements Distinct {
private final Boolean value;
BooleanWrapper(Boolean value) {
this.value = value;
}
public Boolean getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(BooleanWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
// Distinct as Integer wrapper.
@JsonSerialize(using = IntegerWrapper.Serializer.class)
class IntegerWrapper implements Distinct {
private final Integer value;
IntegerWrapper(Integer value) {
this.value = value;
}
public Integer getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(IntegerWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
class Deserializer extends JsonDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
@Override
public Distinct deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize Boolean
if (tree.isBoolean()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Boolean value = parser.readValueAs(Boolean.class);
return new Distinct.BooleanWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Boolean (error: " + e.getMessage() + ") (type: Boolean)");
}
}
// deserialize Integer
if (tree.isInt()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
Integer value = parser.readValueAs(Integer.class);
return new Distinct.IntegerWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf Integer (error: " + e.getMessage() + ") (type: Integer)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public Distinct getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "Distinct cannot be null");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy