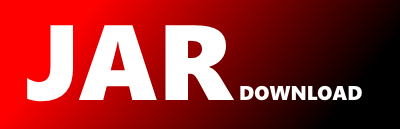
com.algolia.model.search.FetchedIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** FetchedIndex */
public class FetchedIndex {
@JsonProperty("name")
private String name;
@JsonProperty("createdAt")
private String createdAt;
@JsonProperty("updatedAt")
private String updatedAt;
@JsonProperty("entries")
private Integer entries;
@JsonProperty("dataSize")
private Integer dataSize;
@JsonProperty("fileSize")
private Integer fileSize;
@JsonProperty("lastBuildTimeS")
private Integer lastBuildTimeS;
@JsonProperty("numberOfPendingTasks")
private Integer numberOfPendingTasks;
@JsonProperty("pendingTask")
private Boolean pendingTask;
@JsonProperty("primary")
private String primary;
@JsonProperty("replicas")
private List replicas;
@JsonProperty("virtual")
private Boolean virtual;
public FetchedIndex setName(String name) {
this.name = name;
return this;
}
/** Index name. */
@javax.annotation.Nonnull
public String getName() {
return name;
}
public FetchedIndex setCreatedAt(String createdAt) {
this.createdAt = createdAt;
return this;
}
/** Index creation date. An empty string means that the index has no records. */
@javax.annotation.Nonnull
public String getCreatedAt() {
return createdAt;
}
public FetchedIndex setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/** Date and time when the object was updated, in RFC 3339 format. */
@javax.annotation.Nonnull
public String getUpdatedAt() {
return updatedAt;
}
public FetchedIndex setEntries(Integer entries) {
this.entries = entries;
return this;
}
/** Number of records contained in the index. */
@javax.annotation.Nonnull
public Integer getEntries() {
return entries;
}
public FetchedIndex setDataSize(Integer dataSize) {
this.dataSize = dataSize;
return this;
}
/** Number of bytes of the index in minified format. */
@javax.annotation.Nonnull
public Integer getDataSize() {
return dataSize;
}
public FetchedIndex setFileSize(Integer fileSize) {
this.fileSize = fileSize;
return this;
}
/** Number of bytes of the index binary file. */
@javax.annotation.Nonnull
public Integer getFileSize() {
return fileSize;
}
public FetchedIndex setLastBuildTimeS(Integer lastBuildTimeS) {
this.lastBuildTimeS = lastBuildTimeS;
return this;
}
/** Last build time. */
@javax.annotation.Nonnull
public Integer getLastBuildTimeS() {
return lastBuildTimeS;
}
public FetchedIndex setNumberOfPendingTasks(Integer numberOfPendingTasks) {
this.numberOfPendingTasks = numberOfPendingTasks;
return this;
}
/** Number of pending indexing operations. This value is deprecated and should not be used. */
@javax.annotation.Nonnull
public Integer getNumberOfPendingTasks() {
return numberOfPendingTasks;
}
public FetchedIndex setPendingTask(Boolean pendingTask) {
this.pendingTask = pendingTask;
return this;
}
/**
* A boolean which says whether the index has pending tasks. This value is deprecated and should
* not be used.
*/
@javax.annotation.Nonnull
public Boolean getPendingTask() {
return pendingTask;
}
public FetchedIndex setPrimary(String primary) {
this.primary = primary;
return this;
}
/** Only present if the index is a replica. Contains the name of the related primary index. */
@javax.annotation.Nullable
public String getPrimary() {
return primary;
}
public FetchedIndex setReplicas(List replicas) {
this.replicas = replicas;
return this;
}
public FetchedIndex addReplicas(String replicasItem) {
if (this.replicas == null) {
this.replicas = new ArrayList<>();
}
this.replicas.add(replicasItem);
return this;
}
/**
* Only present if the index is a primary index with replicas. Contains the names of all linked
* replicas.
*/
@javax.annotation.Nullable
public List getReplicas() {
return replicas;
}
public FetchedIndex setVirtual(Boolean virtual) {
this.virtual = virtual;
return this;
}
/**
* Only present if the index is a [virtual
* replica](https://www.algolia.com/doc/guides/managing-results/refine-results/sorting/how-to/sort-an-index-alphabetically/#virtual-replicas).
*/
@javax.annotation.Nullable
public Boolean getVirtual() {
return virtual;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FetchedIndex fetchedIndex = (FetchedIndex) o;
return (
Objects.equals(this.name, fetchedIndex.name) &&
Objects.equals(this.createdAt, fetchedIndex.createdAt) &&
Objects.equals(this.updatedAt, fetchedIndex.updatedAt) &&
Objects.equals(this.entries, fetchedIndex.entries) &&
Objects.equals(this.dataSize, fetchedIndex.dataSize) &&
Objects.equals(this.fileSize, fetchedIndex.fileSize) &&
Objects.equals(this.lastBuildTimeS, fetchedIndex.lastBuildTimeS) &&
Objects.equals(this.numberOfPendingTasks, fetchedIndex.numberOfPendingTasks) &&
Objects.equals(this.pendingTask, fetchedIndex.pendingTask) &&
Objects.equals(this.primary, fetchedIndex.primary) &&
Objects.equals(this.replicas, fetchedIndex.replicas) &&
Objects.equals(this.virtual, fetchedIndex.virtual)
);
}
@Override
public int hashCode() {
return Objects.hash(
name,
createdAt,
updatedAt,
entries,
dataSize,
fileSize,
lastBuildTimeS,
numberOfPendingTasks,
pendingTask,
primary,
replicas,
virtual
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FetchedIndex {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" entries: ").append(toIndentedString(entries)).append("\n");
sb.append(" dataSize: ").append(toIndentedString(dataSize)).append("\n");
sb.append(" fileSize: ").append(toIndentedString(fileSize)).append("\n");
sb.append(" lastBuildTimeS: ").append(toIndentedString(lastBuildTimeS)).append("\n");
sb.append(" numberOfPendingTasks: ").append(toIndentedString(numberOfPendingTasks)).append("\n");
sb.append(" pendingTask: ").append(toIndentedString(pendingTask)).append("\n");
sb.append(" primary: ").append(toIndentedString(primary)).append("\n");
sb.append(" replicas: ").append(toIndentedString(replicas)).append("\n");
sb.append(" virtual: ").append(toIndentedString(virtual)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy