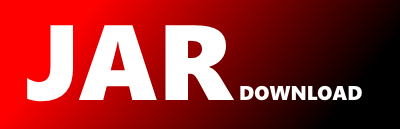
com.algolia.model.search.OptionalWords Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.algolia.exceptions.AlgoliaRuntimeException;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.io.IOException;
import java.util.List;
import java.util.logging.Logger;
/** OptionalWords */
@JsonDeserialize(using = OptionalWords.Deserializer.class)
public interface OptionalWords {
// OptionalWords as String wrapper.
static OptionalWords of(String value) {
return new StringWrapper(value);
}
// OptionalWords as List wrapper.
static OptionalWords of(List value) {
return new ListOfStringWrapper(value);
}
// OptionalWords as String wrapper.
@JsonSerialize(using = StringWrapper.Serializer.class)
class StringWrapper implements OptionalWords {
private final String value;
StringWrapper(String value) {
this.value = value;
}
public String getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(StringWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
// OptionalWords as List wrapper.
@JsonSerialize(using = ListOfStringWrapper.Serializer.class)
class ListOfStringWrapper implements OptionalWords {
private final List value;
ListOfStringWrapper(List value) {
this.value = value;
}
public List getValue() {
return value;
}
static class Serializer extends JsonSerializer {
@Override
public void serialize(ListOfStringWrapper value, JsonGenerator gen, SerializerProvider provider) throws IOException {
gen.writeObject(value.getValue());
}
}
}
class Deserializer extends JsonDeserializer {
private static final Logger LOGGER = Logger.getLogger(Deserializer.class.getName());
@Override
public OptionalWords deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
JsonNode tree = jp.readValueAsTree();
// deserialize String
if (tree.isTextual()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
String value = parser.readValueAs(String.class);
return new OptionalWords.StringWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf String (error: " + e.getMessage() + ") (type: String)");
}
}
// deserialize List
if (tree.isArray()) {
try (JsonParser parser = tree.traverse(jp.getCodec())) {
List value = parser.readValueAs(new TypeReference>() {});
return new OptionalWords.ListOfStringWrapper(value);
} catch (Exception e) {
// deserialization failed, continue
LOGGER.finest("Failed to deserialize oneOf List (error: " + e.getMessage() + ") (type: List)");
}
}
throw new AlgoliaRuntimeException(String.format("Failed to deserialize json element: %s", tree));
}
/** Handle deserialization of the 'null' value. */
@Override
public OptionalWords getNullValue(DeserializationContext ctxt) throws JsonMappingException {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy