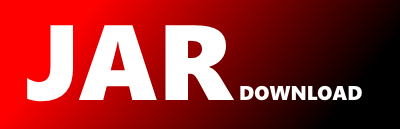
com.algolia.model.search.SearchForFacetValuesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SearchForFacetValuesResponse */
@JsonDeserialize(as = SearchForFacetValuesResponse.class)
public class SearchForFacetValuesResponse implements SearchResult {
@JsonProperty("facetHits")
private List facetHits = new ArrayList<>();
@JsonProperty("exhaustiveFacetsCount")
private Boolean exhaustiveFacetsCount;
@JsonProperty("processingTimeMS")
private Integer processingTimeMS;
public SearchForFacetValuesResponse setFacetHits(List facetHits) {
this.facetHits = facetHits;
return this;
}
public SearchForFacetValuesResponse addFacetHits(FacetHits facetHitsItem) {
this.facetHits.add(facetHitsItem);
return this;
}
/** Matching facet values. */
@javax.annotation.Nonnull
public List getFacetHits() {
return facetHits;
}
public SearchForFacetValuesResponse setExhaustiveFacetsCount(Boolean exhaustiveFacetsCount) {
this.exhaustiveFacetsCount = exhaustiveFacetsCount;
return this;
}
/**
* Whether the facet count is exhaustive (true) or approximate (false). For more information, see
* [Why are my facet and hit counts not
* accurate](https://support.algolia.com/hc/en-us/articles/4406975248145-Why-are-my-facet-and-hit-counts-not-accurate-).
*/
@javax.annotation.Nonnull
public Boolean getExhaustiveFacetsCount() {
return exhaustiveFacetsCount;
}
public SearchForFacetValuesResponse setProcessingTimeMS(Integer processingTimeMS) {
this.processingTimeMS = processingTimeMS;
return this;
}
/** Time the server took to process the request, in milliseconds. */
@javax.annotation.Nullable
public Integer getProcessingTimeMS() {
return processingTimeMS;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SearchForFacetValuesResponse searchForFacetValuesResponse = (SearchForFacetValuesResponse) o;
return (
Objects.equals(this.facetHits, searchForFacetValuesResponse.facetHits) &&
Objects.equals(this.exhaustiveFacetsCount, searchForFacetValuesResponse.exhaustiveFacetsCount) &&
Objects.equals(this.processingTimeMS, searchForFacetValuesResponse.processingTimeMS)
);
}
@Override
public int hashCode() {
return Objects.hash(facetHits, exhaustiveFacetsCount, processingTimeMS);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SearchForFacetValuesResponse {\n");
sb.append(" facetHits: ").append(toIndentedString(facetHits)).append("\n");
sb.append(" exhaustiveFacetsCount: ").append(toIndentedString(exhaustiveFacetsCount)).append("\n");
sb.append(" processingTimeMS: ").append(toIndentedString(processingTimeMS)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy