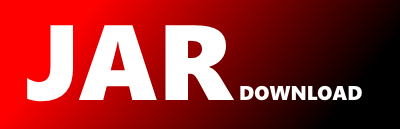
com.algolia.model.search.SearchForFacets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SearchForFacets */
@JsonDeserialize(as = SearchForFacets.class)
public class SearchForFacets implements SearchQuery {
@JsonProperty("params")
private String params;
@JsonProperty("query")
private String query;
@JsonProperty("similarQuery")
private String similarQuery;
@JsonProperty("filters")
private String filters;
@JsonProperty("facetFilters")
private FacetFilters facetFilters;
@JsonProperty("optionalFilters")
private OptionalFilters optionalFilters;
@JsonProperty("numericFilters")
private NumericFilters numericFilters;
@JsonProperty("tagFilters")
private TagFilters tagFilters;
@JsonProperty("sumOrFiltersScores")
private Boolean sumOrFiltersScores;
@JsonProperty("restrictSearchableAttributes")
private List restrictSearchableAttributes;
@JsonProperty("facets")
private List facets;
@JsonProperty("facetingAfterDistinct")
private Boolean facetingAfterDistinct;
@JsonProperty("page")
private Integer page;
@JsonProperty("offset")
private Integer offset;
@JsonProperty("length")
private Integer length;
@JsonProperty("aroundLatLng")
private String aroundLatLng;
@JsonProperty("aroundLatLngViaIP")
private Boolean aroundLatLngViaIP;
@JsonProperty("aroundRadius")
private AroundRadius aroundRadius;
@JsonProperty("aroundPrecision")
private AroundPrecision aroundPrecision;
@JsonProperty("minimumAroundRadius")
private Integer minimumAroundRadius;
@JsonProperty("insideBoundingBox")
private InsideBoundingBox insideBoundingBox;
@JsonProperty("insidePolygon")
private List> insidePolygon;
@JsonProperty("naturalLanguages")
private List naturalLanguages;
@JsonProperty("ruleContexts")
private List ruleContexts;
@JsonProperty("personalizationImpact")
private Integer personalizationImpact;
@JsonProperty("userToken")
private String userToken;
@JsonProperty("getRankingInfo")
private Boolean getRankingInfo;
@JsonProperty("synonyms")
private Boolean synonyms;
@JsonProperty("clickAnalytics")
private Boolean clickAnalytics;
@JsonProperty("analytics")
private Boolean analytics;
@JsonProperty("analyticsTags")
private List analyticsTags;
@JsonProperty("percentileComputation")
private Boolean percentileComputation;
@JsonProperty("enableABTest")
private Boolean enableABTest;
@JsonProperty("attributesToRetrieve")
private List attributesToRetrieve;
@JsonProperty("ranking")
private List ranking;
@JsonProperty("customRanking")
private List customRanking;
@JsonProperty("relevancyStrictness")
private Integer relevancyStrictness;
@JsonProperty("attributesToHighlight")
private List attributesToHighlight;
@JsonProperty("attributesToSnippet")
private List attributesToSnippet;
@JsonProperty("highlightPreTag")
private String highlightPreTag;
@JsonProperty("highlightPostTag")
private String highlightPostTag;
@JsonProperty("snippetEllipsisText")
private String snippetEllipsisText;
@JsonProperty("restrictHighlightAndSnippetArrays")
private Boolean restrictHighlightAndSnippetArrays;
@JsonProperty("hitsPerPage")
private Integer hitsPerPage;
@JsonProperty("minWordSizefor1Typo")
private Integer minWordSizefor1Typo;
@JsonProperty("minWordSizefor2Typos")
private Integer minWordSizefor2Typos;
@JsonProperty("typoTolerance")
private TypoTolerance typoTolerance;
@JsonProperty("allowTyposOnNumericTokens")
private Boolean allowTyposOnNumericTokens;
@JsonProperty("disableTypoToleranceOnAttributes")
private List disableTypoToleranceOnAttributes;
@JsonProperty("ignorePlurals")
private IgnorePlurals ignorePlurals;
@JsonProperty("removeStopWords")
private RemoveStopWords removeStopWords;
@JsonProperty("keepDiacriticsOnCharacters")
private String keepDiacriticsOnCharacters;
@JsonProperty("queryLanguages")
private List queryLanguages;
@JsonProperty("decompoundQuery")
private Boolean decompoundQuery;
@JsonProperty("enableRules")
private Boolean enableRules;
@JsonProperty("enablePersonalization")
private Boolean enablePersonalization;
@JsonProperty("queryType")
private QueryType queryType;
@JsonProperty("removeWordsIfNoResults")
private RemoveWordsIfNoResults removeWordsIfNoResults;
@JsonProperty("mode")
private Mode mode;
@JsonProperty("semanticSearch")
private SemanticSearch semanticSearch;
@JsonProperty("advancedSyntax")
private Boolean advancedSyntax;
@JsonProperty("optionalWords")
private OptionalWords optionalWords;
@JsonProperty("disableExactOnAttributes")
private List disableExactOnAttributes;
@JsonProperty("exactOnSingleWordQuery")
private ExactOnSingleWordQuery exactOnSingleWordQuery;
@JsonProperty("alternativesAsExact")
private List alternativesAsExact;
@JsonProperty("advancedSyntaxFeatures")
private List advancedSyntaxFeatures;
@JsonProperty("distinct")
private Distinct distinct;
@JsonProperty("replaceSynonymsInHighlight")
private Boolean replaceSynonymsInHighlight;
@JsonProperty("minProximity")
private Integer minProximity;
@JsonProperty("responseFields")
private List responseFields;
@JsonProperty("maxValuesPerFacet")
private Integer maxValuesPerFacet;
@JsonProperty("sortFacetValuesBy")
private String sortFacetValuesBy;
@JsonProperty("attributeCriteriaComputedByMinProximity")
private Boolean attributeCriteriaComputedByMinProximity;
@JsonProperty("renderingContent")
private RenderingContent renderingContent;
@JsonProperty("enableReRanking")
private Boolean enableReRanking;
@JsonProperty("reRankingApplyFilter")
private ReRankingApplyFilter reRankingApplyFilter;
@JsonProperty("facet")
private String facet;
@JsonProperty("indexName")
private String indexName;
@JsonProperty("facetQuery")
private String facetQuery;
@JsonProperty("maxFacetHits")
private Integer maxFacetHits;
@JsonProperty("type")
private SearchTypeFacet type;
public SearchForFacets setParams(String params) {
this.params = params;
return this;
}
/** Search parameters as a URL-encoded query string. */
@javax.annotation.Nullable
public String getParams() {
return params;
}
public SearchForFacets setQuery(String query) {
this.query = query;
return this;
}
/** Search query. */
@javax.annotation.Nullable
public String getQuery() {
return query;
}
public SearchForFacets setSimilarQuery(String similarQuery) {
this.similarQuery = similarQuery;
return this;
}
/**
* Keywords to be used instead of the search query to conduct a more broader search. Using the
* `similarQuery` parameter changes other settings: - `queryType` is set to `prefixNone`. -
* `removeStopWords` is set to true. - `words` is set as the first ranking criterion. - All
* remaining words are treated as `optionalWords`. Since the `similarQuery` is supposed to do a
* broad search, they usually return many results. Combine it with `filters` to narrow down the
* list of results.
*/
@javax.annotation.Nullable
public String getSimilarQuery() {
return similarQuery;
}
public SearchForFacets setFilters(String filters) {
this.filters = filters;
return this;
}
/**
* Filter expression to only include items that match the filter criteria in the response. You can
* use these filter expressions: - **Numeric filters.** ` `, where `` is
* one of `<`, `<=`, `=`, `!=`, `>`, `>=`. - **Ranges.** `: TO ` where
* `` and `` are the lower and upper limits of the range (inclusive). - **Facet
* filters.** `:` where `` is a facet attribute (case-sensitive) and
* `` a facet value. - **Tag filters.** `_tags:` or just `` (case-sensitive).
* - **Boolean filters.** `: true | false`. You can combine filters with `AND`, `OR`, and
* `NOT` operators with the following restrictions: - You can only combine filters of the same
* type with `OR`. **Not supported:** `facet:value OR num > 3`. - You can't use `NOT` with
* combinations of filters. **Not supported:** `NOT(facet:value OR facet:value)` - You can't
* combine conjunctions (`AND`) with `OR`. **Not supported:** `facet:value OR (facet:value AND
* facet:value)` Use quotes around your filters, if the facet attribute name or facet value has
* spaces, keywords (`OR`, `AND`, `NOT`), or quotes. If a facet attribute is an array, the filter
* matches if it matches at least one element of the array. For more information, see
* [Filters](https://www.algolia.com/doc/guides/managing-results/refine-results/filtering/).
*/
@javax.annotation.Nullable
public String getFilters() {
return filters;
}
public SearchForFacets setFacetFilters(FacetFilters facetFilters) {
this.facetFilters = facetFilters;
return this;
}
/** Get facetFilters */
@javax.annotation.Nullable
public FacetFilters getFacetFilters() {
return facetFilters;
}
public SearchForFacets setOptionalFilters(OptionalFilters optionalFilters) {
this.optionalFilters = optionalFilters;
return this;
}
/** Get optionalFilters */
@javax.annotation.Nullable
public OptionalFilters getOptionalFilters() {
return optionalFilters;
}
public SearchForFacets setNumericFilters(NumericFilters numericFilters) {
this.numericFilters = numericFilters;
return this;
}
/** Get numericFilters */
@javax.annotation.Nullable
public NumericFilters getNumericFilters() {
return numericFilters;
}
public SearchForFacets setTagFilters(TagFilters tagFilters) {
this.tagFilters = tagFilters;
return this;
}
/** Get tagFilters */
@javax.annotation.Nullable
public TagFilters getTagFilters() {
return tagFilters;
}
public SearchForFacets setSumOrFiltersScores(Boolean sumOrFiltersScores) {
this.sumOrFiltersScores = sumOrFiltersScores;
return this;
}
/**
* Whether to sum all filter scores. If true, all filter scores are summed. Otherwise, the maximum
* filter score is kept. For more information, see [filter
* scores](https://www.algolia.com/doc/guides/managing-results/refine-results/filtering/in-depth/filter-scoring/#accumulating-scores-with-sumorfiltersscores).
*/
@javax.annotation.Nullable
public Boolean getSumOrFiltersScores() {
return sumOrFiltersScores;
}
public SearchForFacets setRestrictSearchableAttributes(List restrictSearchableAttributes) {
this.restrictSearchableAttributes = restrictSearchableAttributes;
return this;
}
public SearchForFacets addRestrictSearchableAttributes(String restrictSearchableAttributesItem) {
if (this.restrictSearchableAttributes == null) {
this.restrictSearchableAttributes = new ArrayList<>();
}
this.restrictSearchableAttributes.add(restrictSearchableAttributesItem);
return this;
}
/**
* Restricts a search to a subset of your searchable attributes. Attribute names are
* case-sensitive.
*/
@javax.annotation.Nullable
public List getRestrictSearchableAttributes() {
return restrictSearchableAttributes;
}
public SearchForFacets setFacets(List facets) {
this.facets = facets;
return this;
}
public SearchForFacets addFacets(String facetsItem) {
if (this.facets == null) {
this.facets = new ArrayList<>();
}
this.facets.add(facetsItem);
return this;
}
/**
* Facets for which to retrieve facet values that match the search criteria and the number of
* matching facet values. To retrieve all facets, use the wildcard character `*`. For more
* information, see
* [facets](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/#contextual-facet-values-and-counts).
*/
@javax.annotation.Nullable
public List getFacets() {
return facets;
}
public SearchForFacets setFacetingAfterDistinct(Boolean facetingAfterDistinct) {
this.facetingAfterDistinct = facetingAfterDistinct;
return this;
}
/**
* Whether faceting should be applied after deduplication with `distinct`. This leads to accurate
* facet counts when using faceting in combination with `distinct`. It's usually better to use
* `afterDistinct` modifiers in the `attributesForFaceting` setting, as `facetingAfterDistinct`
* only computes correct facet counts if all records have the same facet values for the
* `attributeForDistinct`.
*/
@javax.annotation.Nullable
public Boolean getFacetingAfterDistinct() {
return facetingAfterDistinct;
}
public SearchForFacets setPage(Integer page) {
this.page = page;
return this;
}
/** Page of search results to retrieve. minimum: 0 */
@javax.annotation.Nullable
public Integer getPage() {
return page;
}
public SearchForFacets setOffset(Integer offset) {
this.offset = offset;
return this;
}
/** Position of the first hit to retrieve. */
@javax.annotation.Nullable
public Integer getOffset() {
return offset;
}
public SearchForFacets setLength(Integer length) {
this.length = length;
return this;
}
/** Number of hits to retrieve (used in combination with `offset`). minimum: 0 maximum: 1000 */
@javax.annotation.Nullable
public Integer getLength() {
return length;
}
public SearchForFacets setAroundLatLng(String aroundLatLng) {
this.aroundLatLng = aroundLatLng;
return this;
}
/**
* Coordinates for the center of a circle, expressed as a comma-separated string of latitude and
* longitude. Only records included within a circle around this central location are included in
* the results. The radius of the circle is determined by the `aroundRadius` and
* `minimumAroundRadius` settings. This parameter is ignored if you also specify `insidePolygon`
* or `insideBoundingBox`.
*/
@javax.annotation.Nullable
public String getAroundLatLng() {
return aroundLatLng;
}
public SearchForFacets setAroundLatLngViaIP(Boolean aroundLatLngViaIP) {
this.aroundLatLngViaIP = aroundLatLngViaIP;
return this;
}
/** Whether to obtain the coordinates from the request's IP address. */
@javax.annotation.Nullable
public Boolean getAroundLatLngViaIP() {
return aroundLatLngViaIP;
}
public SearchForFacets setAroundRadius(AroundRadius aroundRadius) {
this.aroundRadius = aroundRadius;
return this;
}
/** Get aroundRadius */
@javax.annotation.Nullable
public AroundRadius getAroundRadius() {
return aroundRadius;
}
public SearchForFacets setAroundPrecision(AroundPrecision aroundPrecision) {
this.aroundPrecision = aroundPrecision;
return this;
}
/** Get aroundPrecision */
@javax.annotation.Nullable
public AroundPrecision getAroundPrecision() {
return aroundPrecision;
}
public SearchForFacets setMinimumAroundRadius(Integer minimumAroundRadius) {
this.minimumAroundRadius = minimumAroundRadius;
return this;
}
/**
* Minimum radius (in meters) for a search around a location when `aroundRadius` isn't set.
* minimum: 1
*/
@javax.annotation.Nullable
public Integer getMinimumAroundRadius() {
return minimumAroundRadius;
}
public SearchForFacets setInsideBoundingBox(InsideBoundingBox insideBoundingBox) {
this.insideBoundingBox = insideBoundingBox;
return this;
}
/** Get insideBoundingBox */
@javax.annotation.Nullable
public InsideBoundingBox getInsideBoundingBox() {
return insideBoundingBox;
}
public SearchForFacets setInsidePolygon(List> insidePolygon) {
this.insidePolygon = insidePolygon;
return this;
}
public SearchForFacets addInsidePolygon(List insidePolygonItem) {
if (this.insidePolygon == null) {
this.insidePolygon = new ArrayList<>();
}
this.insidePolygon.add(insidePolygonItem);
return this;
}
/**
* Coordinates of a polygon in which to search. Polygons are defined by 3 to 10,000 points. Each
* point is represented by its latitude and longitude. Provide multiple polygons as nested arrays.
* For more information, see [filtering inside
* polygons](https://www.algolia.com/doc/guides/managing-results/refine-results/geolocation/#filtering-inside-rectangular-or-polygonal-areas).
* This parameter is ignored if you also specify `insideBoundingBox`.
*/
@javax.annotation.Nullable
public List> getInsidePolygon() {
return insidePolygon;
}
public SearchForFacets setNaturalLanguages(List naturalLanguages) {
this.naturalLanguages = naturalLanguages;
return this;
}
public SearchForFacets addNaturalLanguages(SupportedLanguage naturalLanguagesItem) {
if (this.naturalLanguages == null) {
this.naturalLanguages = new ArrayList<>();
}
this.naturalLanguages.add(naturalLanguagesItem);
return this;
}
/**
* ISO language codes that adjust settings that are useful for processing natural language queries
* (as opposed to keyword searches): - Sets `removeStopWords` and `ignorePlurals` to the list of
* provided languages. - Sets `removeWordsIfNoResults` to `allOptional`. - Adds a
* `natural_language` attribute to `ruleContexts` and `analyticsTags`.
*/
@javax.annotation.Nullable
public List getNaturalLanguages() {
return naturalLanguages;
}
public SearchForFacets setRuleContexts(List ruleContexts) {
this.ruleContexts = ruleContexts;
return this;
}
public SearchForFacets addRuleContexts(String ruleContextsItem) {
if (this.ruleContexts == null) {
this.ruleContexts = new ArrayList<>();
}
this.ruleContexts.add(ruleContextsItem);
return this;
}
/**
* Assigns a rule context to the search query. [Rule
* contexts](https://www.algolia.com/doc/guides/managing-results/rules/rules-overview/how-to/customize-search-results-by-platform/#whats-a-context)
* are strings that you can use to trigger matching rules.
*/
@javax.annotation.Nullable
public List getRuleContexts() {
return ruleContexts;
}
public SearchForFacets setPersonalizationImpact(Integer personalizationImpact) {
this.personalizationImpact = personalizationImpact;
return this;
}
/**
* Impact that Personalization should have on this search. The higher this value is, the more
* Personalization determines the ranking compared to other factors. For more information, see
* [Understanding Personalization
* impact](https://www.algolia.com/doc/guides/personalization/personalizing-results/in-depth/configuring-personalization/#understanding-personalization-impact).
* minimum: 0 maximum: 100
*/
@javax.annotation.Nullable
public Integer getPersonalizationImpact() {
return personalizationImpact;
}
public SearchForFacets setUserToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* Unique pseudonymous or anonymous user identifier. This helps with analytics and click and
* conversion events. For more information, see [user
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/).
*/
@javax.annotation.Nullable
public String getUserToken() {
return userToken;
}
public SearchForFacets setGetRankingInfo(Boolean getRankingInfo) {
this.getRankingInfo = getRankingInfo;
return this;
}
/** Whether the search response should include detailed ranking information. */
@javax.annotation.Nullable
public Boolean getGetRankingInfo() {
return getRankingInfo;
}
public SearchForFacets setSynonyms(Boolean synonyms) {
this.synonyms = synonyms;
return this;
}
/** Whether to take into account an index's synonyms for this search. */
@javax.annotation.Nullable
public Boolean getSynonyms() {
return synonyms;
}
public SearchForFacets setClickAnalytics(Boolean clickAnalytics) {
this.clickAnalytics = clickAnalytics;
return this;
}
/**
* Whether to include a `queryID` attribute in the response. The query ID is a unique identifier
* for a search query and is required for tracking [click and conversion
* events](https://www.algolia.com/guides/sending-events/getting-started/).
*/
@javax.annotation.Nullable
public Boolean getClickAnalytics() {
return clickAnalytics;
}
public SearchForFacets setAnalytics(Boolean analytics) {
this.analytics = analytics;
return this;
}
/** Whether this search will be included in Analytics. */
@javax.annotation.Nullable
public Boolean getAnalytics() {
return analytics;
}
public SearchForFacets setAnalyticsTags(List analyticsTags) {
this.analyticsTags = analyticsTags;
return this;
}
public SearchForFacets addAnalyticsTags(String analyticsTagsItem) {
if (this.analyticsTags == null) {
this.analyticsTags = new ArrayList<>();
}
this.analyticsTags.add(analyticsTagsItem);
return this;
}
/**
* Tags to apply to the query for [segmenting analytics
* data](https://www.algolia.com/doc/guides/search-analytics/guides/segments/).
*/
@javax.annotation.Nullable
public List getAnalyticsTags() {
return analyticsTags;
}
public SearchForFacets setPercentileComputation(Boolean percentileComputation) {
this.percentileComputation = percentileComputation;
return this;
}
/** Whether to include this search when calculating processing-time percentiles. */
@javax.annotation.Nullable
public Boolean getPercentileComputation() {
return percentileComputation;
}
public SearchForFacets setEnableABTest(Boolean enableABTest) {
this.enableABTest = enableABTest;
return this;
}
/** Whether to enable A/B testing for this search. */
@javax.annotation.Nullable
public Boolean getEnableABTest() {
return enableABTest;
}
public SearchForFacets setAttributesToRetrieve(List attributesToRetrieve) {
this.attributesToRetrieve = attributesToRetrieve;
return this;
}
public SearchForFacets addAttributesToRetrieve(String attributesToRetrieveItem) {
if (this.attributesToRetrieve == null) {
this.attributesToRetrieve = new ArrayList<>();
}
this.attributesToRetrieve.add(attributesToRetrieveItem);
return this;
}
/**
* Attributes to include in the API response. To reduce the size of your response, you can
* retrieve only some of the attributes. Attribute names are case-sensitive. - `*` retrieves all
* attributes, except attributes included in the `customRanking` and `unretrievableAttributes`
* settings. - To retrieve all attributes except a specific one, prefix the attribute with a dash
* and combine it with the `*`: `[\"*\", \"-ATTRIBUTE\"]`. - The `objectID` attribute is always
* included.
*/
@javax.annotation.Nullable
public List getAttributesToRetrieve() {
return attributesToRetrieve;
}
public SearchForFacets setRanking(List ranking) {
this.ranking = ranking;
return this;
}
public SearchForFacets addRanking(String rankingItem) {
if (this.ranking == null) {
this.ranking = new ArrayList<>();
}
this.ranking.add(rankingItem);
return this;
}
/**
* Determines the order in which Algolia returns your results. By default, each entry corresponds
* to a [ranking
* criteria](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/).
* The tie-breaking algorithm sequentially applies each criterion in the order they're specified.
* If you configure a replica index for [sorting by an
* attribute](https://www.algolia.com/doc/guides/managing-results/refine-results/sorting/how-to/sort-by-attribute/),
* you put the sorting attribute at the top of the list. **Modifiers** - `asc(\"ATTRIBUTE\")`.
* Sort the index by the values of an attribute, in ascending order. - `desc(\"ATTRIBUTE\")`. Sort
* the index by the values of an attribute, in descending order. Before you modify the default
* setting, you should test your changes in the dashboard, and by [A/B
* testing](https://www.algolia.com/doc/guides/ab-testing/what-is-ab-testing/).
*/
@javax.annotation.Nullable
public List getRanking() {
return ranking;
}
public SearchForFacets setCustomRanking(List customRanking) {
this.customRanking = customRanking;
return this;
}
public SearchForFacets addCustomRanking(String customRankingItem) {
if (this.customRanking == null) {
this.customRanking = new ArrayList<>();
}
this.customRanking.add(customRankingItem);
return this;
}
/**
* Attributes to use as [custom
* ranking](https://www.algolia.com/doc/guides/managing-results/must-do/custom-ranking/).
* Attribute names are case-sensitive. The custom ranking attributes decide which items are shown
* first if the other ranking criteria are equal. Records with missing values for your selected
* custom ranking attributes are always sorted last. Boolean attributes are sorted based on their
* alphabetical order. **Modifiers** - `asc(\"ATTRIBUTE\")`. Sort the index by the values of an
* attribute, in ascending order. - `desc(\"ATTRIBUTE\")`. Sort the index by the values of an
* attribute, in descending order. If you use two or more custom ranking attributes, [reduce the
* precision](https://www.algolia.com/doc/guides/managing-results/must-do/custom-ranking/how-to/controlling-custom-ranking-metrics-precision/)
* of your first attributes, or the other attributes will never be applied.
*/
@javax.annotation.Nullable
public List getCustomRanking() {
return customRanking;
}
public SearchForFacets setRelevancyStrictness(Integer relevancyStrictness) {
this.relevancyStrictness = relevancyStrictness;
return this;
}
/**
* Relevancy threshold below which less relevant results aren't included in the results. You can
* only set `relevancyStrictness` on [virtual replica
* indices](https://www.algolia.com/doc/guides/managing-results/refine-results/sorting/in-depth/replicas/#what-are-virtual-replicas).
* Use this setting to strike a balance between the relevance and number of returned results.
*/
@javax.annotation.Nullable
public Integer getRelevancyStrictness() {
return relevancyStrictness;
}
public SearchForFacets setAttributesToHighlight(List attributesToHighlight) {
this.attributesToHighlight = attributesToHighlight;
return this;
}
public SearchForFacets addAttributesToHighlight(String attributesToHighlightItem) {
if (this.attributesToHighlight == null) {
this.attributesToHighlight = new ArrayList<>();
}
this.attributesToHighlight.add(attributesToHighlightItem);
return this;
}
/**
* Attributes to highlight. By default, all searchable attributes are highlighted. Use `*` to
* highlight all attributes or use an empty array `[]` to turn off highlighting. Attribute names
* are case-sensitive. With highlighting, strings that match the search query are surrounded by
* HTML tags defined by `highlightPreTag` and `highlightPostTag`. You can use this to visually
* highlight matching parts of a search query in your UI. For more information, see [Highlighting
* and
* snippeting](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/highlighting-snippeting/js/).
*/
@javax.annotation.Nullable
public List getAttributesToHighlight() {
return attributesToHighlight;
}
public SearchForFacets setAttributesToSnippet(List attributesToSnippet) {
this.attributesToSnippet = attributesToSnippet;
return this;
}
public SearchForFacets addAttributesToSnippet(String attributesToSnippetItem) {
if (this.attributesToSnippet == null) {
this.attributesToSnippet = new ArrayList<>();
}
this.attributesToSnippet.add(attributesToSnippetItem);
return this;
}
/**
* Attributes for which to enable snippets. Attribute names are case-sensitive. Snippets provide
* additional context to matched words. If you enable snippets, they include 10 words, including
* the matched word. The matched word will also be wrapped by HTML tags for highlighting. You can
* adjust the number of words with the following notation: `ATTRIBUTE:NUMBER`, where `NUMBER` is
* the number of words to be extracted.
*/
@javax.annotation.Nullable
public List getAttributesToSnippet() {
return attributesToSnippet;
}
public SearchForFacets setHighlightPreTag(String highlightPreTag) {
this.highlightPreTag = highlightPreTag;
return this;
}
/** HTML tag to insert before the highlighted parts in all highlighted results and snippets. */
@javax.annotation.Nullable
public String getHighlightPreTag() {
return highlightPreTag;
}
public SearchForFacets setHighlightPostTag(String highlightPostTag) {
this.highlightPostTag = highlightPostTag;
return this;
}
/** HTML tag to insert after the highlighted parts in all highlighted results and snippets. */
@javax.annotation.Nullable
public String getHighlightPostTag() {
return highlightPostTag;
}
public SearchForFacets setSnippetEllipsisText(String snippetEllipsisText) {
this.snippetEllipsisText = snippetEllipsisText;
return this;
}
/** String used as an ellipsis indicator when a snippet is truncated. */
@javax.annotation.Nullable
public String getSnippetEllipsisText() {
return snippetEllipsisText;
}
public SearchForFacets setRestrictHighlightAndSnippetArrays(Boolean restrictHighlightAndSnippetArrays) {
this.restrictHighlightAndSnippetArrays = restrictHighlightAndSnippetArrays;
return this;
}
/**
* Whether to restrict highlighting and snippeting to items that at least partially matched the
* search query. By default, all items are highlighted and snippeted.
*/
@javax.annotation.Nullable
public Boolean getRestrictHighlightAndSnippetArrays() {
return restrictHighlightAndSnippetArrays;
}
public SearchForFacets setHitsPerPage(Integer hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
/** Number of hits per page. minimum: 1 maximum: 1000 */
@javax.annotation.Nullable
public Integer getHitsPerPage() {
return hitsPerPage;
}
public SearchForFacets setMinWordSizefor1Typo(Integer minWordSizefor1Typo) {
this.minWordSizefor1Typo = minWordSizefor1Typo;
return this;
}
/**
* Minimum number of characters a word in the search query must contain to accept matches with
* [one
* typo](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor1Typo() {
return minWordSizefor1Typo;
}
public SearchForFacets setMinWordSizefor2Typos(Integer minWordSizefor2Typos) {
this.minWordSizefor2Typos = minWordSizefor2Typos;
return this;
}
/**
* Minimum number of characters a word in the search query must contain to accept matches with
* [two
* typos](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/in-depth/configuring-typo-tolerance/#configuring-word-length-for-typos).
*/
@javax.annotation.Nullable
public Integer getMinWordSizefor2Typos() {
return minWordSizefor2Typos;
}
public SearchForFacets setTypoTolerance(TypoTolerance typoTolerance) {
this.typoTolerance = typoTolerance;
return this;
}
/** Get typoTolerance */
@javax.annotation.Nullable
public TypoTolerance getTypoTolerance() {
return typoTolerance;
}
public SearchForFacets setAllowTyposOnNumericTokens(Boolean allowTyposOnNumericTokens) {
this.allowTyposOnNumericTokens = allowTyposOnNumericTokens;
return this;
}
/**
* Whether to allow typos on numbers in the search query. Turn off this setting to reduce the
* number of irrelevant matches when searching in large sets of similar numbers.
*/
@javax.annotation.Nullable
public Boolean getAllowTyposOnNumericTokens() {
return allowTyposOnNumericTokens;
}
public SearchForFacets setDisableTypoToleranceOnAttributes(List disableTypoToleranceOnAttributes) {
this.disableTypoToleranceOnAttributes = disableTypoToleranceOnAttributes;
return this;
}
public SearchForFacets addDisableTypoToleranceOnAttributes(String disableTypoToleranceOnAttributesItem) {
if (this.disableTypoToleranceOnAttributes == null) {
this.disableTypoToleranceOnAttributes = new ArrayList<>();
}
this.disableTypoToleranceOnAttributes.add(disableTypoToleranceOnAttributesItem);
return this;
}
/**
* Attributes for which you want to turn off [typo
* tolerance](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/).
* Attribute names are case-sensitive. Returning only exact matches can help when: - [Searching in
* hyphenated
* attributes](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/typo-tolerance/how-to/how-to-search-in-hyphenated-attributes/).
* - Reducing the number of matches when you have too many. This can happen with attributes that
* are long blocks of text, such as product descriptions. Consider alternatives such as
* `disableTypoToleranceOnWords` or adding synonyms if your attributes have intentional unusual
* spellings that might look like typos.
*/
@javax.annotation.Nullable
public List getDisableTypoToleranceOnAttributes() {
return disableTypoToleranceOnAttributes;
}
public SearchForFacets setIgnorePlurals(IgnorePlurals ignorePlurals) {
this.ignorePlurals = ignorePlurals;
return this;
}
/** Get ignorePlurals */
@javax.annotation.Nullable
public IgnorePlurals getIgnorePlurals() {
return ignorePlurals;
}
public SearchForFacets setRemoveStopWords(RemoveStopWords removeStopWords) {
this.removeStopWords = removeStopWords;
return this;
}
/** Get removeStopWords */
@javax.annotation.Nullable
public RemoveStopWords getRemoveStopWords() {
return removeStopWords;
}
public SearchForFacets setKeepDiacriticsOnCharacters(String keepDiacriticsOnCharacters) {
this.keepDiacriticsOnCharacters = keepDiacriticsOnCharacters;
return this;
}
/**
* Characters for which diacritics should be preserved. By default, Algolia removes diacritics
* from letters. For example, `é` becomes `e`. If this causes issues in your search, you can
* specify characters that should keep their diacritics.
*/
@javax.annotation.Nullable
public String getKeepDiacriticsOnCharacters() {
return keepDiacriticsOnCharacters;
}
public SearchForFacets setQueryLanguages(List queryLanguages) {
this.queryLanguages = queryLanguages;
return this;
}
public SearchForFacets addQueryLanguages(SupportedLanguage queryLanguagesItem) {
if (this.queryLanguages == null) {
this.queryLanguages = new ArrayList<>();
}
this.queryLanguages.add(queryLanguagesItem);
return this;
}
/**
* Languages for language-specific query processing steps such as plurals, stop-word removal, and
* word-detection dictionaries. This setting sets a default list of languages used by the
* `removeStopWords` and `ignorePlurals` settings. This setting also sets a dictionary for word
* detection in the logogram-based
* [CJK](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/normalization/#normalization-for-logogram-based-languages-cjk)
* languages. To support this, you must place the CJK language **first**. **You should always
* specify a query language.** If you don't specify an indexing language, the search engine uses
* all [supported
* languages](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/supported-languages/),
* or the languages you specified with the `ignorePlurals` or `removeStopWords` parameters. This
* can lead to unexpected search results. For more information, see [Language-specific
* configuration](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/language-specific-configurations/).
*/
@javax.annotation.Nullable
public List getQueryLanguages() {
return queryLanguages;
}
public SearchForFacets setDecompoundQuery(Boolean decompoundQuery) {
this.decompoundQuery = decompoundQuery;
return this;
}
/**
* Whether to split compound words in the query into their building blocks. For more information,
* see [Word
* segmentation](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/language-specific-configurations/#splitting-compound-words).
* Word segmentation is supported for these languages: German, Dutch, Finnish, Swedish, and
* Norwegian. Decompounding doesn't work for words with [non-spacing mark Unicode
* characters](https://www.charactercodes.net/category/non-spacing_mark). For example,
* `Gartenstühle` won't be decompounded if the `ü` consists of `u` (U+0075) and `◌̈` (U+0308).
*/
@javax.annotation.Nullable
public Boolean getDecompoundQuery() {
return decompoundQuery;
}
public SearchForFacets setEnableRules(Boolean enableRules) {
this.enableRules = enableRules;
return this;
}
/** Whether to enable rules. */
@javax.annotation.Nullable
public Boolean getEnableRules() {
return enableRules;
}
public SearchForFacets setEnablePersonalization(Boolean enablePersonalization) {
this.enablePersonalization = enablePersonalization;
return this;
}
/** Whether to enable Personalization. */
@javax.annotation.Nullable
public Boolean getEnablePersonalization() {
return enablePersonalization;
}
public SearchForFacets setQueryType(QueryType queryType) {
this.queryType = queryType;
return this;
}
/** Get queryType */
@javax.annotation.Nullable
public QueryType getQueryType() {
return queryType;
}
public SearchForFacets setRemoveWordsIfNoResults(RemoveWordsIfNoResults removeWordsIfNoResults) {
this.removeWordsIfNoResults = removeWordsIfNoResults;
return this;
}
/** Get removeWordsIfNoResults */
@javax.annotation.Nullable
public RemoveWordsIfNoResults getRemoveWordsIfNoResults() {
return removeWordsIfNoResults;
}
public SearchForFacets setMode(Mode mode) {
this.mode = mode;
return this;
}
/** Get mode */
@javax.annotation.Nullable
public Mode getMode() {
return mode;
}
public SearchForFacets setSemanticSearch(SemanticSearch semanticSearch) {
this.semanticSearch = semanticSearch;
return this;
}
/** Get semanticSearch */
@javax.annotation.Nullable
public SemanticSearch getSemanticSearch() {
return semanticSearch;
}
public SearchForFacets setAdvancedSyntax(Boolean advancedSyntax) {
this.advancedSyntax = advancedSyntax;
return this;
}
/**
* Whether to support phrase matching and excluding words from search queries. Use the
* `advancedSyntaxFeatures` parameter to control which feature is supported.
*/
@javax.annotation.Nullable
public Boolean getAdvancedSyntax() {
return advancedSyntax;
}
public SearchForFacets setOptionalWords(OptionalWords optionalWords) {
this.optionalWords = optionalWords;
return this;
}
/** Get optionalWords */
@javax.annotation.Nullable
public OptionalWords getOptionalWords() {
return optionalWords;
}
public SearchForFacets setDisableExactOnAttributes(List disableExactOnAttributes) {
this.disableExactOnAttributes = disableExactOnAttributes;
return this;
}
public SearchForFacets addDisableExactOnAttributes(String disableExactOnAttributesItem) {
if (this.disableExactOnAttributes == null) {
this.disableExactOnAttributes = new ArrayList<>();
}
this.disableExactOnAttributes.add(disableExactOnAttributesItem);
return this;
}
/**
* Searchable attributes for which you want to [turn off the Exact ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/override-search-engine-defaults/in-depth/adjust-exact-settings/#turn-off-exact-for-some-attributes).
* Attribute names are case-sensitive. This can be useful for attributes with long values, where
* the likelihood of an exact match is high, such as product descriptions. Turning off the Exact
* ranking criterion for these attributes favors exact matching on other attributes. This reduces
* the impact of individual attributes with a lot of content on ranking.
*/
@javax.annotation.Nullable
public List getDisableExactOnAttributes() {
return disableExactOnAttributes;
}
public SearchForFacets setExactOnSingleWordQuery(ExactOnSingleWordQuery exactOnSingleWordQuery) {
this.exactOnSingleWordQuery = exactOnSingleWordQuery;
return this;
}
/** Get exactOnSingleWordQuery */
@javax.annotation.Nullable
public ExactOnSingleWordQuery getExactOnSingleWordQuery() {
return exactOnSingleWordQuery;
}
public SearchForFacets setAlternativesAsExact(List alternativesAsExact) {
this.alternativesAsExact = alternativesAsExact;
return this;
}
public SearchForFacets addAlternativesAsExact(AlternativesAsExact alternativesAsExactItem) {
if (this.alternativesAsExact == null) {
this.alternativesAsExact = new ArrayList<>();
}
this.alternativesAsExact.add(alternativesAsExactItem);
return this;
}
/**
* Determine which plurals and synonyms should be considered an exact matches. By default, Algolia
* treats singular and plural forms of a word, and single-word synonyms, as
* [exact](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#exact)
* matches when searching. For example: - \"swimsuit\" and \"swimsuits\" are treated the same -
* \"swimsuit\" and \"swimwear\" are treated the same (if they are
* [synonyms](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/adding-synonyms/#regular-synonyms)).
* - `ignorePlurals`. Plurals and similar declensions added by the `ignorePlurals` setting are
* considered exact matches. - `singleWordSynonym`. Single-word synonyms, such as \"NY\" =
* \"NYC\", are considered exact matches. - `multiWordsSynonym`. Multi-word synonyms, such as
* \"NY\" = \"New York\", are considered exact matches.
*/
@javax.annotation.Nullable
public List getAlternativesAsExact() {
return alternativesAsExact;
}
public SearchForFacets setAdvancedSyntaxFeatures(List advancedSyntaxFeatures) {
this.advancedSyntaxFeatures = advancedSyntaxFeatures;
return this;
}
public SearchForFacets addAdvancedSyntaxFeatures(AdvancedSyntaxFeatures advancedSyntaxFeaturesItem) {
if (this.advancedSyntaxFeatures == null) {
this.advancedSyntaxFeatures = new ArrayList<>();
}
this.advancedSyntaxFeatures.add(advancedSyntaxFeaturesItem);
return this;
}
/**
* Advanced search syntax features you want to support. - `exactPhrase`. Phrases in quotes must
* match exactly. For example, `sparkly blue \"iPhone case\"` only returns records with the exact
* string \"iPhone case\". - `excludeWords`. Query words prefixed with a `-` must not occur in a
* record. For example, `search -engine` matches records that contain \"search\" but not
* \"engine\". This setting only has an effect if `advancedSyntax` is true.
*/
@javax.annotation.Nullable
public List getAdvancedSyntaxFeatures() {
return advancedSyntaxFeatures;
}
public SearchForFacets setDistinct(Distinct distinct) {
this.distinct = distinct;
return this;
}
/** Get distinct */
@javax.annotation.Nullable
public Distinct getDistinct() {
return distinct;
}
public SearchForFacets setReplaceSynonymsInHighlight(Boolean replaceSynonymsInHighlight) {
this.replaceSynonymsInHighlight = replaceSynonymsInHighlight;
return this;
}
/**
* Whether to replace a highlighted word with the matched synonym. By default, the original words
* are highlighted even if a synonym matches. For example, with `home` as a synonym for `house`
* and a search for `home`, records matching either \"home\" or \"house\" are included in the
* search results, and either \"home\" or \"house\" are highlighted. With
* `replaceSynonymsInHighlight` set to `true`, a search for `home` still matches the same records,
* but all occurrences of \"house\" are replaced by \"home\" in the highlighted response.
*/
@javax.annotation.Nullable
public Boolean getReplaceSynonymsInHighlight() {
return replaceSynonymsInHighlight;
}
public SearchForFacets setMinProximity(Integer minProximity) {
this.minProximity = minProximity;
return this;
}
/**
* Minimum proximity score for two matching words. This adjusts the [Proximity ranking
* criterion](https://www.algolia.com/doc/guides/managing-results/relevance-overview/in-depth/ranking-criteria/#proximity)
* by equally scoring matches that are farther apart. For example, if `minProximity` is 2,
* neighboring matches and matches with one word between them would have the same score. minimum:
* 1 maximum: 7
*/
@javax.annotation.Nullable
public Integer getMinProximity() {
return minProximity;
}
public SearchForFacets setResponseFields(List responseFields) {
this.responseFields = responseFields;
return this;
}
public SearchForFacets addResponseFields(String responseFieldsItem) {
if (this.responseFields == null) {
this.responseFields = new ArrayList<>();
}
this.responseFields.add(responseFieldsItem);
return this;
}
/**
* Properties to include in the API response of `search` and `browse` requests. By default, all
* response properties are included. To reduce the response size, you can select, which attributes
* should be included. You can't exclude these properties: `message`, `warning`, `cursor`,
* `serverUsed`, `indexUsed`, `abTestVariantID`, `parsedQuery`, or any property triggered by the
* `getRankingInfo` parameter. Don't exclude properties that you might need in your search UI.
*/
@javax.annotation.Nullable
public List getResponseFields() {
return responseFields;
}
public SearchForFacets setMaxValuesPerFacet(Integer maxValuesPerFacet) {
this.maxValuesPerFacet = maxValuesPerFacet;
return this;
}
/** Maximum number of facet values to return for each facet. maximum: 1000 */
@javax.annotation.Nullable
public Integer getMaxValuesPerFacet() {
return maxValuesPerFacet;
}
public SearchForFacets setSortFacetValuesBy(String sortFacetValuesBy) {
this.sortFacetValuesBy = sortFacetValuesBy;
return this;
}
/**
* Order in which to retrieve facet values. - `count`. Facet values are retrieved by decreasing
* count. The count is the number of matching records containing this facet value. - `alpha`.
* Retrieve facet values alphabetically. This setting doesn't influence how facet values are
* displayed in your UI (see `renderingContent`). For more information, see [facet value
* display](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/facet-display/js/).
*/
@javax.annotation.Nullable
public String getSortFacetValuesBy() {
return sortFacetValuesBy;
}
public SearchForFacets setAttributeCriteriaComputedByMinProximity(Boolean attributeCriteriaComputedByMinProximity) {
this.attributeCriteriaComputedByMinProximity = attributeCriteriaComputedByMinProximity;
return this;
}
/**
* Whether the best matching attribute should be determined by minimum proximity. This setting
* only affects ranking if the Attribute ranking criterion comes before Proximity in the `ranking`
* setting. If true, the best matching attribute is selected based on the minimum proximity of
* multiple matches. Otherwise, the best matching attribute is determined by the order in the
* `searchableAttributes` setting.
*/
@javax.annotation.Nullable
public Boolean getAttributeCriteriaComputedByMinProximity() {
return attributeCriteriaComputedByMinProximity;
}
public SearchForFacets setRenderingContent(RenderingContent renderingContent) {
this.renderingContent = renderingContent;
return this;
}
/** Get renderingContent */
@javax.annotation.Nullable
public RenderingContent getRenderingContent() {
return renderingContent;
}
public SearchForFacets setEnableReRanking(Boolean enableReRanking) {
this.enableReRanking = enableReRanking;
return this;
}
/**
* Whether this search will use [Dynamic
* Re-Ranking](https://www.algolia.com/doc/guides/algolia-ai/re-ranking/). This setting only has
* an effect if you activated Dynamic Re-Ranking for this index in the Algolia dashboard.
*/
@javax.annotation.Nullable
public Boolean getEnableReRanking() {
return enableReRanking;
}
public SearchForFacets setReRankingApplyFilter(ReRankingApplyFilter reRankingApplyFilter) {
this.reRankingApplyFilter = reRankingApplyFilter;
return this;
}
/** Get reRankingApplyFilter */
@javax.annotation.Nullable
public ReRankingApplyFilter getReRankingApplyFilter() {
return reRankingApplyFilter;
}
public SearchForFacets setFacet(String facet) {
this.facet = facet;
return this;
}
/** Facet name. */
@javax.annotation.Nonnull
public String getFacet() {
return facet;
}
public SearchForFacets setIndexName(String indexName) {
this.indexName = indexName;
return this;
}
/** Index name (case-sensitive). */
@javax.annotation.Nonnull
public String getIndexName() {
return indexName;
}
public SearchForFacets setFacetQuery(String facetQuery) {
this.facetQuery = facetQuery;
return this;
}
/** Text to search inside the facet's values. */
@javax.annotation.Nullable
public String getFacetQuery() {
return facetQuery;
}
public SearchForFacets setMaxFacetHits(Integer maxFacetHits) {
this.maxFacetHits = maxFacetHits;
return this;
}
/**
* Maximum number of facet values to return when [searching for facet
* values](https://www.algolia.com/doc/guides/managing-results/refine-results/faceting/#search-for-facet-values).
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getMaxFacetHits() {
return maxFacetHits;
}
public SearchForFacets setType(SearchTypeFacet type) {
this.type = type;
return this;
}
/** Get type */
@javax.annotation.Nonnull
public SearchTypeFacet getType() {
return type;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SearchForFacets searchForFacets = (SearchForFacets) o;
return (
Objects.equals(this.params, searchForFacets.params) &&
Objects.equals(this.query, searchForFacets.query) &&
Objects.equals(this.similarQuery, searchForFacets.similarQuery) &&
Objects.equals(this.filters, searchForFacets.filters) &&
Objects.equals(this.facetFilters, searchForFacets.facetFilters) &&
Objects.equals(this.optionalFilters, searchForFacets.optionalFilters) &&
Objects.equals(this.numericFilters, searchForFacets.numericFilters) &&
Objects.equals(this.tagFilters, searchForFacets.tagFilters) &&
Objects.equals(this.sumOrFiltersScores, searchForFacets.sumOrFiltersScores) &&
Objects.equals(this.restrictSearchableAttributes, searchForFacets.restrictSearchableAttributes) &&
Objects.equals(this.facets, searchForFacets.facets) &&
Objects.equals(this.facetingAfterDistinct, searchForFacets.facetingAfterDistinct) &&
Objects.equals(this.page, searchForFacets.page) &&
Objects.equals(this.offset, searchForFacets.offset) &&
Objects.equals(this.length, searchForFacets.length) &&
Objects.equals(this.aroundLatLng, searchForFacets.aroundLatLng) &&
Objects.equals(this.aroundLatLngViaIP, searchForFacets.aroundLatLngViaIP) &&
Objects.equals(this.aroundRadius, searchForFacets.aroundRadius) &&
Objects.equals(this.aroundPrecision, searchForFacets.aroundPrecision) &&
Objects.equals(this.minimumAroundRadius, searchForFacets.minimumAroundRadius) &&
Objects.equals(this.insideBoundingBox, searchForFacets.insideBoundingBox) &&
Objects.equals(this.insidePolygon, searchForFacets.insidePolygon) &&
Objects.equals(this.naturalLanguages, searchForFacets.naturalLanguages) &&
Objects.equals(this.ruleContexts, searchForFacets.ruleContexts) &&
Objects.equals(this.personalizationImpact, searchForFacets.personalizationImpact) &&
Objects.equals(this.userToken, searchForFacets.userToken) &&
Objects.equals(this.getRankingInfo, searchForFacets.getRankingInfo) &&
Objects.equals(this.synonyms, searchForFacets.synonyms) &&
Objects.equals(this.clickAnalytics, searchForFacets.clickAnalytics) &&
Objects.equals(this.analytics, searchForFacets.analytics) &&
Objects.equals(this.analyticsTags, searchForFacets.analyticsTags) &&
Objects.equals(this.percentileComputation, searchForFacets.percentileComputation) &&
Objects.equals(this.enableABTest, searchForFacets.enableABTest) &&
Objects.equals(this.attributesToRetrieve, searchForFacets.attributesToRetrieve) &&
Objects.equals(this.ranking, searchForFacets.ranking) &&
Objects.equals(this.customRanking, searchForFacets.customRanking) &&
Objects.equals(this.relevancyStrictness, searchForFacets.relevancyStrictness) &&
Objects.equals(this.attributesToHighlight, searchForFacets.attributesToHighlight) &&
Objects.equals(this.attributesToSnippet, searchForFacets.attributesToSnippet) &&
Objects.equals(this.highlightPreTag, searchForFacets.highlightPreTag) &&
Objects.equals(this.highlightPostTag, searchForFacets.highlightPostTag) &&
Objects.equals(this.snippetEllipsisText, searchForFacets.snippetEllipsisText) &&
Objects.equals(this.restrictHighlightAndSnippetArrays, searchForFacets.restrictHighlightAndSnippetArrays) &&
Objects.equals(this.hitsPerPage, searchForFacets.hitsPerPage) &&
Objects.equals(this.minWordSizefor1Typo, searchForFacets.minWordSizefor1Typo) &&
Objects.equals(this.minWordSizefor2Typos, searchForFacets.minWordSizefor2Typos) &&
Objects.equals(this.typoTolerance, searchForFacets.typoTolerance) &&
Objects.equals(this.allowTyposOnNumericTokens, searchForFacets.allowTyposOnNumericTokens) &&
Objects.equals(this.disableTypoToleranceOnAttributes, searchForFacets.disableTypoToleranceOnAttributes) &&
Objects.equals(this.ignorePlurals, searchForFacets.ignorePlurals) &&
Objects.equals(this.removeStopWords, searchForFacets.removeStopWords) &&
Objects.equals(this.keepDiacriticsOnCharacters, searchForFacets.keepDiacriticsOnCharacters) &&
Objects.equals(this.queryLanguages, searchForFacets.queryLanguages) &&
Objects.equals(this.decompoundQuery, searchForFacets.decompoundQuery) &&
Objects.equals(this.enableRules, searchForFacets.enableRules) &&
Objects.equals(this.enablePersonalization, searchForFacets.enablePersonalization) &&
Objects.equals(this.queryType, searchForFacets.queryType) &&
Objects.equals(this.removeWordsIfNoResults, searchForFacets.removeWordsIfNoResults) &&
Objects.equals(this.mode, searchForFacets.mode) &&
Objects.equals(this.semanticSearch, searchForFacets.semanticSearch) &&
Objects.equals(this.advancedSyntax, searchForFacets.advancedSyntax) &&
Objects.equals(this.optionalWords, searchForFacets.optionalWords) &&
Objects.equals(this.disableExactOnAttributes, searchForFacets.disableExactOnAttributes) &&
Objects.equals(this.exactOnSingleWordQuery, searchForFacets.exactOnSingleWordQuery) &&
Objects.equals(this.alternativesAsExact, searchForFacets.alternativesAsExact) &&
Objects.equals(this.advancedSyntaxFeatures, searchForFacets.advancedSyntaxFeatures) &&
Objects.equals(this.distinct, searchForFacets.distinct) &&
Objects.equals(this.replaceSynonymsInHighlight, searchForFacets.replaceSynonymsInHighlight) &&
Objects.equals(this.minProximity, searchForFacets.minProximity) &&
Objects.equals(this.responseFields, searchForFacets.responseFields) &&
Objects.equals(this.maxValuesPerFacet, searchForFacets.maxValuesPerFacet) &&
Objects.equals(this.sortFacetValuesBy, searchForFacets.sortFacetValuesBy) &&
Objects.equals(this.attributeCriteriaComputedByMinProximity, searchForFacets.attributeCriteriaComputedByMinProximity) &&
Objects.equals(this.renderingContent, searchForFacets.renderingContent) &&
Objects.equals(this.enableReRanking, searchForFacets.enableReRanking) &&
Objects.equals(this.reRankingApplyFilter, searchForFacets.reRankingApplyFilter) &&
Objects.equals(this.facet, searchForFacets.facet) &&
Objects.equals(this.indexName, searchForFacets.indexName) &&
Objects.equals(this.facetQuery, searchForFacets.facetQuery) &&
Objects.equals(this.maxFacetHits, searchForFacets.maxFacetHits) &&
Objects.equals(this.type, searchForFacets.type)
);
}
@Override
public int hashCode() {
return Objects.hash(
params,
query,
similarQuery,
filters,
facetFilters,
optionalFilters,
numericFilters,
tagFilters,
sumOrFiltersScores,
restrictSearchableAttributes,
facets,
facetingAfterDistinct,
page,
offset,
length,
aroundLatLng,
aroundLatLngViaIP,
aroundRadius,
aroundPrecision,
minimumAroundRadius,
insideBoundingBox,
insidePolygon,
naturalLanguages,
ruleContexts,
personalizationImpact,
userToken,
getRankingInfo,
synonyms,
clickAnalytics,
analytics,
analyticsTags,
percentileComputation,
enableABTest,
attributesToRetrieve,
ranking,
customRanking,
relevancyStrictness,
attributesToHighlight,
attributesToSnippet,
highlightPreTag,
highlightPostTag,
snippetEllipsisText,
restrictHighlightAndSnippetArrays,
hitsPerPage,
minWordSizefor1Typo,
minWordSizefor2Typos,
typoTolerance,
allowTyposOnNumericTokens,
disableTypoToleranceOnAttributes,
ignorePlurals,
removeStopWords,
keepDiacriticsOnCharacters,
queryLanguages,
decompoundQuery,
enableRules,
enablePersonalization,
queryType,
removeWordsIfNoResults,
mode,
semanticSearch,
advancedSyntax,
optionalWords,
disableExactOnAttributes,
exactOnSingleWordQuery,
alternativesAsExact,
advancedSyntaxFeatures,
distinct,
replaceSynonymsInHighlight,
minProximity,
responseFields,
maxValuesPerFacet,
sortFacetValuesBy,
attributeCriteriaComputedByMinProximity,
renderingContent,
enableReRanking,
reRankingApplyFilter,
facet,
indexName,
facetQuery,
maxFacetHits,
type
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SearchForFacets {\n");
sb.append(" params: ").append(toIndentedString(params)).append("\n");
sb.append(" query: ").append(toIndentedString(query)).append("\n");
sb.append(" similarQuery: ").append(toIndentedString(similarQuery)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append(" facetFilters: ").append(toIndentedString(facetFilters)).append("\n");
sb.append(" optionalFilters: ").append(toIndentedString(optionalFilters)).append("\n");
sb.append(" numericFilters: ").append(toIndentedString(numericFilters)).append("\n");
sb.append(" tagFilters: ").append(toIndentedString(tagFilters)).append("\n");
sb.append(" sumOrFiltersScores: ").append(toIndentedString(sumOrFiltersScores)).append("\n");
sb.append(" restrictSearchableAttributes: ").append(toIndentedString(restrictSearchableAttributes)).append("\n");
sb.append(" facets: ").append(toIndentedString(facets)).append("\n");
sb.append(" facetingAfterDistinct: ").append(toIndentedString(facetingAfterDistinct)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" offset: ").append(toIndentedString(offset)).append("\n");
sb.append(" length: ").append(toIndentedString(length)).append("\n");
sb.append(" aroundLatLng: ").append(toIndentedString(aroundLatLng)).append("\n");
sb.append(" aroundLatLngViaIP: ").append(toIndentedString(aroundLatLngViaIP)).append("\n");
sb.append(" aroundRadius: ").append(toIndentedString(aroundRadius)).append("\n");
sb.append(" aroundPrecision: ").append(toIndentedString(aroundPrecision)).append("\n");
sb.append(" minimumAroundRadius: ").append(toIndentedString(minimumAroundRadius)).append("\n");
sb.append(" insideBoundingBox: ").append(toIndentedString(insideBoundingBox)).append("\n");
sb.append(" insidePolygon: ").append(toIndentedString(insidePolygon)).append("\n");
sb.append(" naturalLanguages: ").append(toIndentedString(naturalLanguages)).append("\n");
sb.append(" ruleContexts: ").append(toIndentedString(ruleContexts)).append("\n");
sb.append(" personalizationImpact: ").append(toIndentedString(personalizationImpact)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append(" getRankingInfo: ").append(toIndentedString(getRankingInfo)).append("\n");
sb.append(" synonyms: ").append(toIndentedString(synonyms)).append("\n");
sb.append(" clickAnalytics: ").append(toIndentedString(clickAnalytics)).append("\n");
sb.append(" analytics: ").append(toIndentedString(analytics)).append("\n");
sb.append(" analyticsTags: ").append(toIndentedString(analyticsTags)).append("\n");
sb.append(" percentileComputation: ").append(toIndentedString(percentileComputation)).append("\n");
sb.append(" enableABTest: ").append(toIndentedString(enableABTest)).append("\n");
sb.append(" attributesToRetrieve: ").append(toIndentedString(attributesToRetrieve)).append("\n");
sb.append(" ranking: ").append(toIndentedString(ranking)).append("\n");
sb.append(" customRanking: ").append(toIndentedString(customRanking)).append("\n");
sb.append(" relevancyStrictness: ").append(toIndentedString(relevancyStrictness)).append("\n");
sb.append(" attributesToHighlight: ").append(toIndentedString(attributesToHighlight)).append("\n");
sb.append(" attributesToSnippet: ").append(toIndentedString(attributesToSnippet)).append("\n");
sb.append(" highlightPreTag: ").append(toIndentedString(highlightPreTag)).append("\n");
sb.append(" highlightPostTag: ").append(toIndentedString(highlightPostTag)).append("\n");
sb.append(" snippetEllipsisText: ").append(toIndentedString(snippetEllipsisText)).append("\n");
sb.append(" restrictHighlightAndSnippetArrays: ").append(toIndentedString(restrictHighlightAndSnippetArrays)).append("\n");
sb.append(" hitsPerPage: ").append(toIndentedString(hitsPerPage)).append("\n");
sb.append(" minWordSizefor1Typo: ").append(toIndentedString(minWordSizefor1Typo)).append("\n");
sb.append(" minWordSizefor2Typos: ").append(toIndentedString(minWordSizefor2Typos)).append("\n");
sb.append(" typoTolerance: ").append(toIndentedString(typoTolerance)).append("\n");
sb.append(" allowTyposOnNumericTokens: ").append(toIndentedString(allowTyposOnNumericTokens)).append("\n");
sb.append(" disableTypoToleranceOnAttributes: ").append(toIndentedString(disableTypoToleranceOnAttributes)).append("\n");
sb.append(" ignorePlurals: ").append(toIndentedString(ignorePlurals)).append("\n");
sb.append(" removeStopWords: ").append(toIndentedString(removeStopWords)).append("\n");
sb.append(" keepDiacriticsOnCharacters: ").append(toIndentedString(keepDiacriticsOnCharacters)).append("\n");
sb.append(" queryLanguages: ").append(toIndentedString(queryLanguages)).append("\n");
sb.append(" decompoundQuery: ").append(toIndentedString(decompoundQuery)).append("\n");
sb.append(" enableRules: ").append(toIndentedString(enableRules)).append("\n");
sb.append(" enablePersonalization: ").append(toIndentedString(enablePersonalization)).append("\n");
sb.append(" queryType: ").append(toIndentedString(queryType)).append("\n");
sb.append(" removeWordsIfNoResults: ").append(toIndentedString(removeWordsIfNoResults)).append("\n");
sb.append(" mode: ").append(toIndentedString(mode)).append("\n");
sb.append(" semanticSearch: ").append(toIndentedString(semanticSearch)).append("\n");
sb.append(" advancedSyntax: ").append(toIndentedString(advancedSyntax)).append("\n");
sb.append(" optionalWords: ").append(toIndentedString(optionalWords)).append("\n");
sb.append(" disableExactOnAttributes: ").append(toIndentedString(disableExactOnAttributes)).append("\n");
sb.append(" exactOnSingleWordQuery: ").append(toIndentedString(exactOnSingleWordQuery)).append("\n");
sb.append(" alternativesAsExact: ").append(toIndentedString(alternativesAsExact)).append("\n");
sb.append(" advancedSyntaxFeatures: ").append(toIndentedString(advancedSyntaxFeatures)).append("\n");
sb.append(" distinct: ").append(toIndentedString(distinct)).append("\n");
sb.append(" replaceSynonymsInHighlight: ").append(toIndentedString(replaceSynonymsInHighlight)).append("\n");
sb.append(" minProximity: ").append(toIndentedString(minProximity)).append("\n");
sb.append(" responseFields: ").append(toIndentedString(responseFields)).append("\n");
sb.append(" maxValuesPerFacet: ").append(toIndentedString(maxValuesPerFacet)).append("\n");
sb.append(" sortFacetValuesBy: ").append(toIndentedString(sortFacetValuesBy)).append("\n");
sb
.append(" attributeCriteriaComputedByMinProximity: ")
.append(toIndentedString(attributeCriteriaComputedByMinProximity))
.append("\n");
sb.append(" renderingContent: ").append(toIndentedString(renderingContent)).append("\n");
sb.append(" enableReRanking: ").append(toIndentedString(enableReRanking)).append("\n");
sb.append(" reRankingApplyFilter: ").append(toIndentedString(reRankingApplyFilter)).append("\n");
sb.append(" facet: ").append(toIndentedString(facet)).append("\n");
sb.append(" indexName: ").append(toIndentedString(indexName)).append("\n");
sb.append(" facetQuery: ").append(toIndentedString(facetQuery)).append("\n");
sb.append(" maxFacetHits: ").append(toIndentedString(maxFacetHits)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy