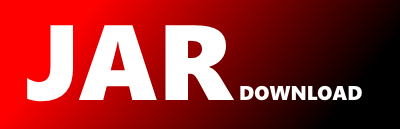
com.algolia.model.search.SearchRulesParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/** Rules search parameters. */
public class SearchRulesParams {
@JsonProperty("query")
private String query;
@JsonProperty("anchoring")
private Anchoring anchoring;
@JsonProperty("context")
private String context;
@JsonProperty("page")
private Integer page;
@JsonProperty("hitsPerPage")
private Integer hitsPerPage;
@JsonProperty("enabled")
private Boolean enabled;
public SearchRulesParams setQuery(String query) {
this.query = query;
return this;
}
/** Search query for rules. */
@javax.annotation.Nullable
public String getQuery() {
return query;
}
public SearchRulesParams setAnchoring(Anchoring anchoring) {
this.anchoring = anchoring;
return this;
}
/** Get anchoring */
@javax.annotation.Nullable
public Anchoring getAnchoring() {
return anchoring;
}
public SearchRulesParams setContext(String context) {
this.context = context;
return this;
}
/** Only return rules that match the context (exact match). */
@javax.annotation.Nullable
public String getContext() {
return context;
}
public SearchRulesParams setPage(Integer page) {
this.page = page;
return this;
}
/**
* Requested page of the API response. Algolia uses `page` and `hitsPerPage` to control how search
* results are displayed
* ([paginated](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/)).
* - `hitsPerPage`: sets the number of search results (_hits_) displayed per page. - `page`:
* specifies the page number of the search results you want to retrieve. Page numbering starts at
* 0, so the first page is `page=0`, the second is `page=1`, and so on. For example, to display 10
* results per page starting from the third page, set `hitsPerPage` to 10 and `page` to 2.
* minimum: 0
*/
@javax.annotation.Nullable
public Integer getPage() {
return page;
}
public SearchRulesParams setHitsPerPage(Integer hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
/**
* Maximum number of hits per page. Algolia uses `page` and `hitsPerPage` to control how search
* results are displayed
* ([paginated](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/)).
* - `hitsPerPage`: sets the number of search results (_hits_) displayed per page. - `page`:
* specifies the page number of the search results you want to retrieve. Page numbering starts at
* 0, so the first page is `page=0`, the second is `page=1`, and so on. For example, to display 10
* results per page starting from the third page, set `hitsPerPage` to 10 and `page` to 2.
* minimum: 1 maximum: 1000
*/
@javax.annotation.Nullable
public Integer getHitsPerPage() {
return hitsPerPage;
}
public SearchRulesParams setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* If `true`, return only enabled rules. If `false`, return only inactive rules. By default, _all_
* rules are returned.
*/
@javax.annotation.Nullable
public Boolean getEnabled() {
return enabled;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SearchRulesParams searchRulesParams = (SearchRulesParams) o;
return (
Objects.equals(this.query, searchRulesParams.query) &&
Objects.equals(this.anchoring, searchRulesParams.anchoring) &&
Objects.equals(this.context, searchRulesParams.context) &&
Objects.equals(this.page, searchRulesParams.page) &&
Objects.equals(this.hitsPerPage, searchRulesParams.hitsPerPage) &&
Objects.equals(this.enabled, searchRulesParams.enabled)
);
}
@Override
public int hashCode() {
return Objects.hash(query, anchoring, context, page, hitsPerPage, enabled);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SearchRulesParams {\n");
sb.append(" query: ").append(toIndentedString(query)).append("\n");
sb.append(" anchoring: ").append(toIndentedString(anchoring)).append("\n");
sb.append(" context: ").append(toIndentedString(context)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" hitsPerPage: ").append(toIndentedString(hitsPerPage)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy