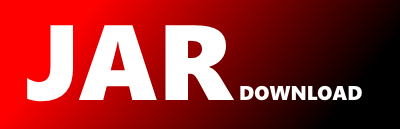
com.algolia.model.search.SearchUserIdsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** userIDs data. */
public class SearchUserIdsResponse {
@JsonProperty("hits")
private List hits = new ArrayList<>();
@JsonProperty("nbHits")
private Integer nbHits;
@JsonProperty("page")
private Integer page;
@JsonProperty("hitsPerPage")
private Integer hitsPerPage;
@JsonProperty("updatedAt")
private String updatedAt;
public SearchUserIdsResponse setHits(List hits) {
this.hits = hits;
return this;
}
public SearchUserIdsResponse addHits(UserHit hitsItem) {
this.hits.add(hitsItem);
return this;
}
/** User objects that match the query. */
@javax.annotation.Nonnull
public List getHits() {
return hits;
}
public SearchUserIdsResponse setNbHits(Integer nbHits) {
this.nbHits = nbHits;
return this;
}
/** Number of results (hits). */
@javax.annotation.Nonnull
public Integer getNbHits() {
return nbHits;
}
public SearchUserIdsResponse setPage(Integer page) {
this.page = page;
return this;
}
/** Page of search results to retrieve. minimum: 0 */
@javax.annotation.Nonnull
public Integer getPage() {
return page;
}
public SearchUserIdsResponse setHitsPerPage(Integer hitsPerPage) {
this.hitsPerPage = hitsPerPage;
return this;
}
/**
* Maximum number of hits per page. Algolia uses `page` and `hitsPerPage` to control how search
* results are displayed
* ([paginated](https://www.algolia.com/doc/guides/building-search-ui/ui-and-ux-patterns/pagination/js/)).
* - `hitsPerPage`: sets the number of search results (_hits_) displayed per page. - `page`:
* specifies the page number of the search results you want to retrieve. Page numbering starts at
* 0, so the first page is `page=0`, the second is `page=1`, and so on. For example, to display 10
* results per page starting from the third page, set `hitsPerPage` to 10 and `page` to 2.
* minimum: 1 maximum: 1000
*/
@javax.annotation.Nonnull
public Integer getHitsPerPage() {
return hitsPerPage;
}
public SearchUserIdsResponse setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/** Date and time when the object was updated, in RFC 3339 format. */
@javax.annotation.Nonnull
public String getUpdatedAt() {
return updatedAt;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SearchUserIdsResponse searchUserIdsResponse = (SearchUserIdsResponse) o;
return (
Objects.equals(this.hits, searchUserIdsResponse.hits) &&
Objects.equals(this.nbHits, searchUserIdsResponse.nbHits) &&
Objects.equals(this.page, searchUserIdsResponse.page) &&
Objects.equals(this.hitsPerPage, searchUserIdsResponse.hitsPerPage) &&
Objects.equals(this.updatedAt, searchUserIdsResponse.updatedAt)
);
}
@Override
public int hashCode() {
return Objects.hash(hits, nbHits, page, hitsPerPage, updatedAt);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SearchUserIdsResponse {\n");
sb.append(" hits: ").append(toIndentedString(hits)).append("\n");
sb.append(" nbHits: ").append(toIndentedString(nbHits)).append("\n");
sb.append(" page: ").append(toIndentedString(page)).append("\n");
sb.append(" hitsPerPage: ").append(toIndentedString(hitsPerPage)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy