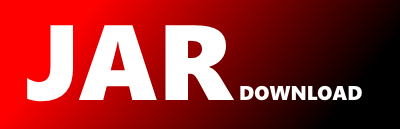
com.algolia.model.search.SecuredApiKeyRestrictions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SecuredApiKeyRestrictions */
public class SecuredApiKeyRestrictions {
@JsonProperty("searchParams")
private SearchParamsObject searchParams;
@JsonProperty("filters")
private String filters;
@JsonProperty("validUntil")
private Long validUntil;
@JsonProperty("restrictIndices")
private List restrictIndices;
@JsonProperty("restrictSources")
private String restrictSources;
@JsonProperty("userToken")
private String userToken;
public SecuredApiKeyRestrictions setSearchParams(SearchParamsObject searchParams) {
this.searchParams = searchParams;
return this;
}
/** Get searchParams */
@javax.annotation.Nullable
public SearchParamsObject getSearchParams() {
return searchParams;
}
public SecuredApiKeyRestrictions setFilters(String filters) {
this.filters = filters;
return this;
}
/**
* Filters that apply to every search made with the secured API key. Extra filters added at search
* time will be combined with `AND`. For example, if you set `group:admin` as fixed filter on your
* generated API key, and add `groups:visitors` to the search query, the complete set of filters
* will be `group:admin AND groups:visitors`.
*/
@javax.annotation.Nullable
public String getFilters() {
return filters;
}
public SecuredApiKeyRestrictions setValidUntil(Long validUntil) {
this.validUntil = validUntil;
return this;
}
/** Timestamp when the secured API key expires, measured in seconds since the Unix epoch. */
@javax.annotation.Nullable
public Long getValidUntil() {
return validUntil;
}
public SecuredApiKeyRestrictions setRestrictIndices(List restrictIndices) {
this.restrictIndices = restrictIndices;
return this;
}
public SecuredApiKeyRestrictions addRestrictIndices(String restrictIndicesItem) {
if (this.restrictIndices == null) {
this.restrictIndices = new ArrayList<>();
}
this.restrictIndices.add(restrictIndicesItem);
return this;
}
/**
* Index names or patterns that this API key can access. By default, an API key can access all
* indices in the same application. You can use leading and trailing wildcard characters (`*`): -
* `dev_*` matches all indices starting with \"dev_\". - `*_dev` matches all indices ending with
* \"_dev\". - `*_products_*` matches all indices containing \"_products_\".
*/
@javax.annotation.Nullable
public List getRestrictIndices() {
return restrictIndices;
}
public SecuredApiKeyRestrictions setRestrictSources(String restrictSources) {
this.restrictSources = restrictSources;
return this;
}
/**
* IP network that are allowed to use this key. You can only add a single source, but you can
* provide a range of IP addresses. Use this to protect against API key leaking and reuse.
*/
@javax.annotation.Nullable
public String getRestrictSources() {
return restrictSources;
}
public SecuredApiKeyRestrictions setUserToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* Pseudonymous user identifier to restrict usage of this API key to specific users. By default,
* rate limits are set based on IP addresses. This can be an issue if many users search from the
* same IP address. To avoid this, add a user token to each generated API key.
*/
@javax.annotation.Nullable
public String getUserToken() {
return userToken;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SecuredApiKeyRestrictions securedApiKeyRestrictions = (SecuredApiKeyRestrictions) o;
return (
Objects.equals(this.searchParams, securedApiKeyRestrictions.searchParams) &&
Objects.equals(this.filters, securedApiKeyRestrictions.filters) &&
Objects.equals(this.validUntil, securedApiKeyRestrictions.validUntil) &&
Objects.equals(this.restrictIndices, securedApiKeyRestrictions.restrictIndices) &&
Objects.equals(this.restrictSources, securedApiKeyRestrictions.restrictSources) &&
Objects.equals(this.userToken, securedApiKeyRestrictions.userToken)
);
}
@Override
public int hashCode() {
return Objects.hash(searchParams, filters, validUntil, restrictIndices, restrictSources, userToken);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SecuredApiKeyRestrictions {\n");
sb.append(" searchParams: ").append(toIndentedString(searchParams)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append(" validUntil: ").append(toIndentedString(validUntil)).append("\n");
sb.append(" restrictIndices: ").append(toIndentedString(restrictIndices)).append("\n");
sb.append(" restrictSources: ").append(toIndentedString(restrictSources)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy