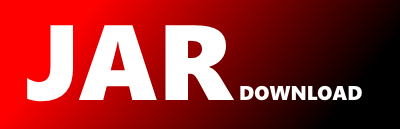
com.algolia.model.search.StandardEntries Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Key-value pairs of [supported language ISO
* codes](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/handling-natural-languages-nlp/in-depth/supported-languages/)
* and boolean values.
*/
public class StandardEntries {
@JsonProperty("plurals")
private Map plurals;
@JsonProperty("stopwords")
private Map stopwords;
@JsonProperty("compounds")
private Map compounds;
public StandardEntries setPlurals(Map plurals) {
this.plurals = plurals;
return this;
}
public StandardEntries putPlurals(String key, Boolean pluralsItem) {
if (this.plurals == null) {
this.plurals = new HashMap<>();
}
this.plurals.put(key, pluralsItem);
return this;
}
/** Key-value pair of a language ISO code and a boolean value. */
@javax.annotation.Nullable
public Map getPlurals() {
return plurals;
}
public StandardEntries setStopwords(Map stopwords) {
this.stopwords = stopwords;
return this;
}
public StandardEntries putStopwords(String key, Boolean stopwordsItem) {
if (this.stopwords == null) {
this.stopwords = new HashMap<>();
}
this.stopwords.put(key, stopwordsItem);
return this;
}
/** Key-value pair of a language ISO code and a boolean value. */
@javax.annotation.Nullable
public Map getStopwords() {
return stopwords;
}
public StandardEntries setCompounds(Map compounds) {
this.compounds = compounds;
return this;
}
public StandardEntries putCompounds(String key, Boolean compoundsItem) {
if (this.compounds == null) {
this.compounds = new HashMap<>();
}
this.compounds.put(key, compoundsItem);
return this;
}
/** Key-value pair of a language ISO code and a boolean value. */
@javax.annotation.Nullable
public Map getCompounds() {
return compounds;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StandardEntries standardEntries = (StandardEntries) o;
return (
Objects.equals(this.plurals, standardEntries.plurals) &&
Objects.equals(this.stopwords, standardEntries.stopwords) &&
Objects.equals(this.compounds, standardEntries.compounds)
);
}
@Override
public int hashCode() {
return Objects.hash(plurals, stopwords, compounds);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StandardEntries {\n");
sb.append(" plurals: ").append(toIndentedString(plurals)).append("\n");
sb.append(" stopwords: ").append(toIndentedString(stopwords)).append("\n");
sb.append(" compounds: ").append(toIndentedString(compounds)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy