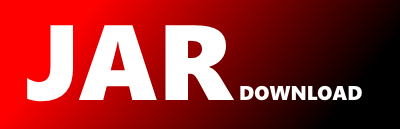
com.algolia.model.search.SynonymHit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
The newest version!
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.search;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** Synonym object. */
public class SynonymHit {
@JsonProperty("objectID")
private String objectID;
@JsonProperty("type")
private SynonymType type;
@JsonProperty("synonyms")
private List synonyms;
@JsonProperty("input")
private String input;
@JsonProperty("word")
private String word;
@JsonProperty("corrections")
private List corrections;
@JsonProperty("placeholder")
private String placeholder;
@JsonProperty("replacements")
private List replacements;
public SynonymHit setObjectID(String objectID) {
this.objectID = objectID;
return this;
}
/** Unique identifier of a synonym object. */
@javax.annotation.Nonnull
public String getObjectID() {
return objectID;
}
public SynonymHit setType(SynonymType type) {
this.type = type;
return this;
}
/** Get type */
@javax.annotation.Nonnull
public SynonymType getType() {
return type;
}
public SynonymHit setSynonyms(List synonyms) {
this.synonyms = synonyms;
return this;
}
public SynonymHit addSynonyms(String synonymsItem) {
if (this.synonyms == null) {
this.synonyms = new ArrayList<>();
}
this.synonyms.add(synonymsItem);
return this;
}
/** Words or phrases considered equivalent. */
@javax.annotation.Nullable
public List getSynonyms() {
return synonyms;
}
public SynonymHit setInput(String input) {
this.input = input;
return this;
}
/**
* Word or phrase to appear in query strings (for
* [`onewaysynonym`s](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/adding-synonyms/in-depth/one-way-synonyms/)).
*/
@javax.annotation.Nullable
public String getInput() {
return input;
}
public SynonymHit setWord(String word) {
this.word = word;
return this;
}
/**
* Word or phrase to appear in query strings (for [`altcorrection1` and
* `altcorrection2`](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/adding-synonyms/in-depth/synonyms-alternative-corrections/)).
*/
@javax.annotation.Nullable
public String getWord() {
return word;
}
public SynonymHit setCorrections(List corrections) {
this.corrections = corrections;
return this;
}
public SynonymHit addCorrections(String correctionsItem) {
if (this.corrections == null) {
this.corrections = new ArrayList<>();
}
this.corrections.add(correctionsItem);
return this;
}
/** Words to be matched in records. */
@javax.annotation.Nullable
public List getCorrections() {
return corrections;
}
public SynonymHit setPlaceholder(String placeholder) {
this.placeholder = placeholder;
return this;
}
/**
* [Placeholder
* token](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/adding-synonyms/in-depth/synonyms-placeholders/)
* to be put inside records.
*/
@javax.annotation.Nullable
public String getPlaceholder() {
return placeholder;
}
public SynonymHit setReplacements(List replacements) {
this.replacements = replacements;
return this;
}
public SynonymHit addReplacements(String replacementsItem) {
if (this.replacements == null) {
this.replacements = new ArrayList<>();
}
this.replacements.add(replacementsItem);
return this;
}
/**
* Query words that will match the [placeholder
* token](https://www.algolia.com/doc/guides/managing-results/optimize-search-results/adding-synonyms/in-depth/synonyms-placeholders/).
*/
@javax.annotation.Nullable
public List getReplacements() {
return replacements;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SynonymHit synonymHit = (SynonymHit) o;
return (
Objects.equals(this.objectID, synonymHit.objectID) &&
Objects.equals(this.type, synonymHit.type) &&
Objects.equals(this.synonyms, synonymHit.synonyms) &&
Objects.equals(this.input, synonymHit.input) &&
Objects.equals(this.word, synonymHit.word) &&
Objects.equals(this.corrections, synonymHit.corrections) &&
Objects.equals(this.placeholder, synonymHit.placeholder) &&
Objects.equals(this.replacements, synonymHit.replacements)
);
}
@Override
public int hashCode() {
return Objects.hash(objectID, type, synonyms, input, word, corrections, placeholder, replacements);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SynonymHit {\n");
sb.append(" objectID: ").append(toIndentedString(objectID)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" synonyms: ").append(toIndentedString(synonyms)).append("\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" word: ").append(toIndentedString(word)).append("\n");
sb.append(" corrections: ").append(toIndentedString(corrections)).append("\n");
sb.append(" placeholder: ").append(toIndentedString(placeholder)).append("\n");
sb.append(" replacements: ").append(toIndentedString(replacements)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy