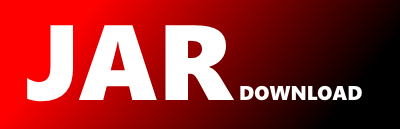
com.algolia.model.ingestion.SourceWatchResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algoliasearch Show documentation
Show all versions of algoliasearch Show documentation
Java client for Algolia Search API
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** SourceWatchResponse */
public class SourceWatchResponse {
@JsonProperty("runID")
private String runID;
@JsonProperty("data")
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy