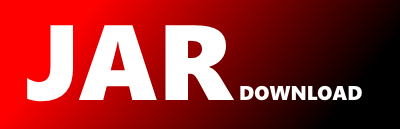
com.algolia.model.analytics.GetAverageClickPositionResponse Maven / Gradle / Ivy
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.analytics;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** GetAverageClickPositionResponse */
public class GetAverageClickPositionResponse {
@JsonProperty("average")
private Double average;
@JsonProperty("clickCount")
private Integer clickCount;
@JsonProperty("dates")
private List dates = new ArrayList<>();
public GetAverageClickPositionResponse setAverage(Double average) {
this.average = average;
return this;
}
/**
* Average position of a clicked search result in the list of search results. If null, Algolia
* didn't receive any search requests with `clickAnalytics` set to true. minimum: 1
*/
@javax.annotation.Nullable
public Double getAverage() {
return average;
}
public GetAverageClickPositionResponse setClickCount(Integer clickCount) {
this.clickCount = clickCount;
return this;
}
/** Number of clicks associated with this search. minimum: 0 */
@javax.annotation.Nonnull
public Integer getClickCount() {
return clickCount;
}
public GetAverageClickPositionResponse setDates(List dates) {
this.dates = dates;
return this;
}
public GetAverageClickPositionResponse addDates(DailyAverageClicks datesItem) {
this.dates.add(datesItem);
return this;
}
/** Daily average click positions. */
@javax.annotation.Nonnull
public List getDates() {
return dates;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetAverageClickPositionResponse getAverageClickPositionResponse = (GetAverageClickPositionResponse) o;
return (
Objects.equals(this.average, getAverageClickPositionResponse.average) &&
Objects.equals(this.clickCount, getAverageClickPositionResponse.clickCount) &&
Objects.equals(this.dates, getAverageClickPositionResponse.dates)
);
}
@Override
public int hashCode() {
return Objects.hash(average, clickCount, dates);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetAverageClickPositionResponse {\n");
sb.append(" average: ").append(toIndentedString(average)).append("\n");
sb.append(" clickCount: ").append(toIndentedString(clickCount)).append("\n");
sb.append(" dates: ").append(toIndentedString(dates)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy