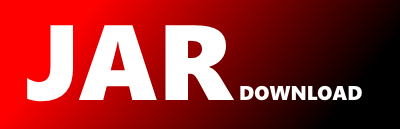
com.algolia.model.ingestion.CommercetoolsCustomFields Maven / Gradle / Ivy
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Custom fields from commercetools to add to the records. For more information, see [Using Custom
* Types and Custom Fields](https://docs.commercetools.com/tutorials/custom-types).
*/
public class CommercetoolsCustomFields {
@JsonProperty("inventory")
private List inventory;
@JsonProperty("price")
private List price;
@JsonProperty("category")
private List category;
public CommercetoolsCustomFields setInventory(List inventory) {
this.inventory = inventory;
return this;
}
public CommercetoolsCustomFields addInventory(String inventoryItem) {
if (this.inventory == null) {
this.inventory = new ArrayList<>();
}
this.inventory.add(inventoryItem);
return this;
}
/** Inventory custom fields. */
@javax.annotation.Nullable
public List getInventory() {
return inventory;
}
public CommercetoolsCustomFields setPrice(List price) {
this.price = price;
return this;
}
public CommercetoolsCustomFields addPrice(String priceItem) {
if (this.price == null) {
this.price = new ArrayList<>();
}
this.price.add(priceItem);
return this;
}
/** Price custom fields. */
@javax.annotation.Nullable
public List getPrice() {
return price;
}
public CommercetoolsCustomFields setCategory(List category) {
this.category = category;
return this;
}
public CommercetoolsCustomFields addCategory(String categoryItem) {
if (this.category == null) {
this.category = new ArrayList<>();
}
this.category.add(categoryItem);
return this;
}
/** Category custom fields. */
@javax.annotation.Nullable
public List getCategory() {
return category;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CommercetoolsCustomFields commercetoolsCustomFields = (CommercetoolsCustomFields) o;
return (
Objects.equals(this.inventory, commercetoolsCustomFields.inventory) &&
Objects.equals(this.price, commercetoolsCustomFields.price) &&
Objects.equals(this.category, commercetoolsCustomFields.category)
);
}
@Override
public int hashCode() {
return Objects.hash(inventory, price, category);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CommercetoolsCustomFields {\n");
sb.append(" inventory: ").append(toIndentedString(inventory)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy