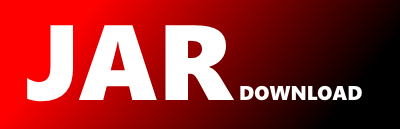
com.algolia.model.ingestion.TaskCreateV1 Maven / Gradle / Ivy
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.ingestion;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.Objects;
/**
* API request body for creating a task using the V1 shape, please use methods and types that don't
* contain the V1 suffix.
*
* @deprecated
*/
@Deprecated
public class TaskCreateV1 {
@JsonProperty("sourceID")
private String sourceID;
@JsonProperty("destinationID")
private String destinationID;
@JsonProperty("trigger")
private TaskCreateTrigger trigger;
@JsonProperty("action")
private ActionType action;
@JsonProperty("enabled")
private Boolean enabled;
@JsonProperty("failureThreshold")
private Integer failureThreshold;
@JsonProperty("input")
private TaskInput input;
@JsonProperty("cursor")
private String cursor;
public TaskCreateV1 setSourceID(String sourceID) {
this.sourceID = sourceID;
return this;
}
/** Universally uniqud identifier (UUID) of a source. */
@javax.annotation.Nonnull
public String getSourceID() {
return sourceID;
}
public TaskCreateV1 setDestinationID(String destinationID) {
this.destinationID = destinationID;
return this;
}
/** Universally unique identifier (UUID) of a destination resource. */
@javax.annotation.Nonnull
public String getDestinationID() {
return destinationID;
}
public TaskCreateV1 setTrigger(TaskCreateTrigger trigger) {
this.trigger = trigger;
return this;
}
/** Get trigger */
@javax.annotation.Nonnull
public TaskCreateTrigger getTrigger() {
return trigger;
}
public TaskCreateV1 setAction(ActionType action) {
this.action = action;
return this;
}
/** Get action */
@javax.annotation.Nonnull
public ActionType getAction() {
return action;
}
public TaskCreateV1 setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/** Whether the task is enabled. */
@javax.annotation.Nullable
public Boolean getEnabled() {
return enabled;
}
public TaskCreateV1 setFailureThreshold(Integer failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
/**
* Maximum accepted percentage of failures for a task run to finish successfully. minimum: 0
* maximum: 100
*/
@javax.annotation.Nullable
public Integer getFailureThreshold() {
return failureThreshold;
}
public TaskCreateV1 setInput(TaskInput input) {
this.input = input;
return this;
}
/** Get input */
@javax.annotation.Nullable
public TaskInput getInput() {
return input;
}
public TaskCreateV1 setCursor(String cursor) {
this.cursor = cursor;
return this;
}
/** Date of the last cursor in RFC 3339 format. */
@javax.annotation.Nullable
public String getCursor() {
return cursor;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskCreateV1 taskCreateV1 = (TaskCreateV1) o;
return (
Objects.equals(this.sourceID, taskCreateV1.sourceID) &&
Objects.equals(this.destinationID, taskCreateV1.destinationID) &&
Objects.equals(this.trigger, taskCreateV1.trigger) &&
Objects.equals(this.action, taskCreateV1.action) &&
Objects.equals(this.enabled, taskCreateV1.enabled) &&
Objects.equals(this.failureThreshold, taskCreateV1.failureThreshold) &&
Objects.equals(this.input, taskCreateV1.input) &&
Objects.equals(this.cursor, taskCreateV1.cursor)
);
}
@Override
public int hashCode() {
return Objects.hash(sourceID, destinationID, trigger, action, enabled, failureThreshold, input, cursor);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskCreateV1 {\n");
sb.append(" sourceID: ").append(toIndentedString(sourceID)).append("\n");
sb.append(" destinationID: ").append(toIndentedString(destinationID)).append("\n");
sb.append(" trigger: ").append(toIndentedString(trigger)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" failureThreshold: ").append(toIndentedString(failureThreshold)).append("\n");
sb.append(" input: ").append(toIndentedString(input)).append("\n");
sb.append(" cursor: ").append(toIndentedString(cursor)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy