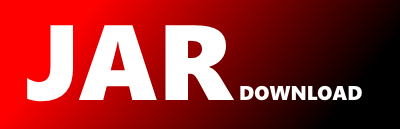
com.algolia.model.insights.PurchasedObjectIDs Maven / Gradle / Ivy
// Code generated by OpenAPI Generator (https://openapi-generator.tech), manual changes will be lost
// - read more on https://github.com/algolia/api-clients-automation. DO NOT EDIT.
package com.algolia.model.insights;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.databind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Use this event to track when users make a purchase unrelated to a previous Algolia request. For
* example, if you don't use Algolia to build your category pages, use this event. To track purchase
* events related to Algolia requests, use the \"Purchased object IDs after search\" event.
*/
@JsonDeserialize(as = PurchasedObjectIDs.class)
public class PurchasedObjectIDs implements EventsItems {
@JsonProperty("eventName")
private String eventName;
@JsonProperty("eventType")
private ConversionEvent eventType;
@JsonProperty("eventSubtype")
private PurchaseEvent eventSubtype;
@JsonProperty("index")
private String index;
@JsonProperty("objectIDs")
private List objectIDs = new ArrayList<>();
@JsonProperty("userToken")
private String userToken;
@JsonProperty("authenticatedUserToken")
private String authenticatedUserToken;
@JsonProperty("currency")
private String currency;
@JsonProperty("objectData")
private List objectData;
@JsonProperty("timestamp")
private Long timestamp;
@JsonProperty("value")
private Value value;
public PurchasedObjectIDs setEventName(String eventName) {
this.eventName = eventName;
return this;
}
/**
* Event name, up to 64 ASCII characters. Consider naming events consistently—for example, by
* adopting Segment's
* [object-action](https://segment.com/academy/collecting-data/naming-conventions-for-clean-data/#the-object-action-framework)
* framework.
*/
@javax.annotation.Nonnull
public String getEventName() {
return eventName;
}
public PurchasedObjectIDs setEventType(ConversionEvent eventType) {
this.eventType = eventType;
return this;
}
/** Get eventType */
@javax.annotation.Nonnull
public ConversionEvent getEventType() {
return eventType;
}
public PurchasedObjectIDs setEventSubtype(PurchaseEvent eventSubtype) {
this.eventSubtype = eventSubtype;
return this;
}
/** Get eventSubtype */
@javax.annotation.Nonnull
public PurchaseEvent getEventSubtype() {
return eventSubtype;
}
public PurchasedObjectIDs setIndex(String index) {
this.index = index;
return this;
}
/** Index name (case-sensitive) to which the event's items belong. */
@javax.annotation.Nonnull
public String getIndex() {
return index;
}
public PurchasedObjectIDs setObjectIDs(List objectIDs) {
this.objectIDs = objectIDs;
return this;
}
public PurchasedObjectIDs addObjectIDs(String objectIDsItem) {
this.objectIDs.add(objectIDsItem);
return this;
}
/** Object IDs of the records that are part of the event. */
@javax.annotation.Nonnull
public List getObjectIDs() {
return objectIDs;
}
public PurchasedObjectIDs setUserToken(String userToken) {
this.userToken = userToken;
return this;
}
/**
* Anonymous or pseudonymous user identifier. Don't use personally identifiable information in
* user tokens. For more information, see [User
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/).
*/
@javax.annotation.Nonnull
public String getUserToken() {
return userToken;
}
public PurchasedObjectIDs setAuthenticatedUserToken(String authenticatedUserToken) {
this.authenticatedUserToken = authenticatedUserToken;
return this;
}
/**
* Identifier for authenticated users. When the user signs in, you can get an identifier from your
* system and send it as `authenticatedUserToken`. This lets you keep using the `userToken` from
* before the user signed in, while providing a reliable way to identify users across sessions.
* Don't use personally identifiable information in user tokens. For more information, see [User
* token](https://www.algolia.com/doc/guides/sending-events/concepts/usertoken/).
*/
@javax.annotation.Nullable
public String getAuthenticatedUserToken() {
return authenticatedUserToken;
}
public PurchasedObjectIDs setCurrency(String currency) {
this.currency = currency;
return this;
}
/** Three-letter [currency code](https://www.iso.org/iso-4217-currency-codes.html). */
@javax.annotation.Nullable
public String getCurrency() {
return currency;
}
public PurchasedObjectIDs setObjectData(List objectData) {
this.objectData = objectData;
return this;
}
public PurchasedObjectIDs addObjectData(ObjectData objectDataItem) {
if (this.objectData == null) {
this.objectData = new ArrayList<>();
}
this.objectData.add(objectDataItem);
return this;
}
/**
* Extra information about the records involved in a purchase or add-to-cart event. If specified,
* it must have the same length as `objectIDs`.
*/
@javax.annotation.Nullable
public List getObjectData() {
return objectData;
}
public PurchasedObjectIDs setTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Timestamp of the event, measured in milliseconds since the Unix epoch. By default, the Insights
* API uses the time it receives an event as its timestamp.
*/
@javax.annotation.Nullable
public Long getTimestamp() {
return timestamp;
}
public PurchasedObjectIDs setValue(Value value) {
this.value = value;
return this;
}
/** Get value */
@javax.annotation.Nullable
public Value getValue() {
return value;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PurchasedObjectIDs purchasedObjectIDs = (PurchasedObjectIDs) o;
return (
Objects.equals(this.eventName, purchasedObjectIDs.eventName) &&
Objects.equals(this.eventType, purchasedObjectIDs.eventType) &&
Objects.equals(this.eventSubtype, purchasedObjectIDs.eventSubtype) &&
Objects.equals(this.index, purchasedObjectIDs.index) &&
Objects.equals(this.objectIDs, purchasedObjectIDs.objectIDs) &&
Objects.equals(this.userToken, purchasedObjectIDs.userToken) &&
Objects.equals(this.authenticatedUserToken, purchasedObjectIDs.authenticatedUserToken) &&
Objects.equals(this.currency, purchasedObjectIDs.currency) &&
Objects.equals(this.objectData, purchasedObjectIDs.objectData) &&
Objects.equals(this.timestamp, purchasedObjectIDs.timestamp) &&
Objects.equals(this.value, purchasedObjectIDs.value)
);
}
@Override
public int hashCode() {
return Objects.hash(
eventName,
eventType,
eventSubtype,
index,
objectIDs,
userToken,
authenticatedUserToken,
currency,
objectData,
timestamp,
value
);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PurchasedObjectIDs {\n");
sb.append(" eventName: ").append(toIndentedString(eventName)).append("\n");
sb.append(" eventType: ").append(toIndentedString(eventType)).append("\n");
sb.append(" eventSubtype: ").append(toIndentedString(eventSubtype)).append("\n");
sb.append(" index: ").append(toIndentedString(index)).append("\n");
sb.append(" objectIDs: ").append(toIndentedString(objectIDs)).append("\n");
sb.append(" userToken: ").append(toIndentedString(userToken)).append("\n");
sb.append(" authenticatedUserToken: ").append(toIndentedString(authenticatedUserToken)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" objectData: ").append(toIndentedString(objectData)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy