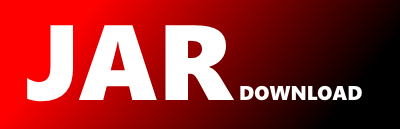
commonTest.selectable.list.TestSelectableListConnectView.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of instantsearch-core Show documentation
Show all versions of instantsearch-core Show documentation
InstantSearch Android is a library providing widgets and helpers to help you build the best instant-search experience on Android with Algolia. It is built on top of Algolia's Kotlin API Client to provide you a high-level solution to quickly build various search interfaces.
package selectable.list
import com.algolia.instantsearch.core.Callback
import com.algolia.instantsearch.core.selectable.list.SelectableItem
import com.algolia.instantsearch.core.selectable.list.SelectableListView
import com.algolia.instantsearch.core.selectable.list.SelectableListViewModel
import com.algolia.instantsearch.core.selectable.list.SelectionMode
import com.algolia.instantsearch.core.selectable.list.connectView
import shouldBeEmpty
import shouldEqual
import shouldNotBeNull
import kotlin.test.Test
class TestSelectableListConnectView {
private val string = "string"
private val items = listOf(string)
private val selections = setOf(string)
private class MockFilterListViewFacet : SelectableListView {
var list: List> = listOf()
override var onSelection: Callback? = null
override fun setItems(items: List>) {
list = items
}
}
@Test
fun connectShouldCallSetItem() {
val view = MockFilterListViewFacet()
val viewModel = SelectableListViewModel(items, SelectionMode.Multiple)
val connection = viewModel.connectView(view)
viewModel.selections.value = selections
connection.connect()
view.list shouldEqual listOf(string to true)
}
@Test
fun onItemsChangedShouldCallSetItem() {
val view = MockFilterListViewFacet()
val viewModel = SelectableListViewModel(emptyList(), SelectionMode.Multiple)
val connection = viewModel.connectView(view)
connection.connect()
viewModel.items.value.shouldBeEmpty()
viewModel.items.value = items
view.list shouldEqual listOf(string to false)
}
@Test
fun onSelectionsChangedShouldCallSetItems() {
val view = MockFilterListViewFacet()
val viewModel = SelectableListViewModel(items, SelectionMode.Multiple)
val connection = viewModel.connectView(view)
connection.connect()
viewModel.selections.value = selections
view.list shouldEqual listOf(string to true)
}
@Test
fun onClickShouldCallOnSelectionsComputed() {
val view = MockFilterListViewFacet()
val viewModel = SelectableListViewModel(items, SelectionMode.Multiple)
val connection = viewModel.connectView(view)
viewModel.eventSelection.subscribe { viewModel.selections.value = it }
connection.connect()
view.onSelection.shouldNotBeNull()
view.onSelection!!(string)
view.list shouldEqual listOf(string to true)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy