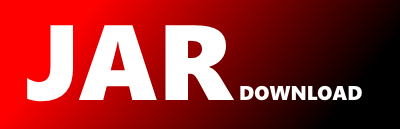
org.apache.flink.runtime.state.heap.HeapFoldingState Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.flink.runtime.state.heap;
import org.apache.flink.api.common.functions.FoldFunction;
import org.apache.flink.api.common.state.FoldingState;
import org.apache.flink.api.common.typeutils.TypeSerializer;
import org.apache.flink.runtime.state.StateTransformationFunction;
import org.apache.flink.runtime.state.internal.InternalFoldingState;
import org.apache.flink.util.Preconditions;
import java.io.IOException;
/**
* Heap-backed partitioned {@link FoldingState} that is snapshotted into files.
*
* @param The type of the key.
* @param The type of the namespace.
* @param The type of the values that can be folded into the state.
* @param The type of the value in the folding state.
*
* @deprecated will be removed in a future version
*/
@Deprecated
public class HeapFoldingState
extends AbstractHeapState>
implements InternalFoldingState {
/** The function used to fold the state */
private final FoldTransformation foldTransformation;
/**
* Creates a new key/value state for the given hash map of key/value pairs.
*
* @param stateTable The state table for which this state is associated to.
* @param keySerializer The serializer for the keys.
* @param valueSerializer The serializer for the state.
* @param namespaceSerializer The serializer for the namespace.
* @param defaultValue The default value for the state.
* @param foldFunction The fold function used for folding state.
*/
public HeapFoldingState(
StateTable stateTable,
TypeSerializer keySerializer,
TypeSerializer valueSerializer,
TypeSerializer namespaceSerializer,
ACC defaultValue,
FoldFunction foldFunction) {
super(stateTable, keySerializer, valueSerializer, namespaceSerializer, defaultValue);
this.foldTransformation = new FoldTransformation(foldFunction);
}
@Override
public TypeSerializer getKeySerializer() {
return keySerializer;
}
@Override
public TypeSerializer getNamespaceSerializer() {
return namespaceSerializer;
}
@Override
public TypeSerializer getValueSerializer() {
return valueSerializer;
}
// ------------------------------------------------------------------------
// state access
// ------------------------------------------------------------------------
@Override
public ACC get() {
return stateTable.get(currentNamespace);
}
@Override
public void add(T value) throws IOException {
if (value == null) {
clear();
return;
}
try {
stateTable.transform(currentNamespace, value, foldTransformation);
} catch (Exception e) {
throw new IOException("Could not add value to folding state.", e);
}
}
private final class FoldTransformation implements StateTransformationFunction {
private final FoldFunction foldFunction;
FoldTransformation(FoldFunction foldFunction) {
this.foldFunction = Preconditions.checkNotNull(foldFunction);
}
@Override
public ACC apply(ACC previousState, T value) throws Exception {
return foldFunction.fold((previousState != null) ? previousState : getDefaultValue(), value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy