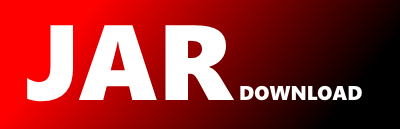
org.apache.flink.table.client.gateway.DescFormatUtil Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.flink.table.client.gateway;
import org.apache.flink.table.api.TableSchema;
/**
* Util methods to format output of "DESCRIBE" DDLs.
*/
public class DescFormatUtil {
public static final String FIELD_DELIM = "\t";
public static final String LINE_DELIM = "\n";
private static final int ALIGNMENT = 20;
private DescFormatUtil(){}
// keep in line with TableSchema.toString()
public static String colDesc(TableSchema tableSchema, String colName) {
int index = tableSchema.getColumnIndex(colName);
return " |-- name: " + colName + "\n" +
" |-- type: " + tableSchema.getFieldType(index).get() + "\n" +
" |-- isNullable: " + tableSchema.getFieldNullables()[index] + "\n";
}
public static void formatNamedValue(String name, String value, StringBuilder builder) {
builder.append(String.format("%-" + ALIGNMENT + "s", name)).append("\t");
int colNameLength = ALIGNMENT > name.length() ? ALIGNMENT : name.length();
indentMultilineValue(value, builder, new int[]{0, colNameLength}, true);
}
private static void indentMultilineValue(String value, StringBuilder tableInfo,
int[] columnWidths, boolean printNull) {
if (value == null) {
if (printNull) {
tableInfo.append(String.format("%-" + ALIGNMENT + "s", value));
}
tableInfo.append(LINE_DELIM);
} else {
String[] valueSegments = value.split("\n|\r|\r\n");
tableInfo.append(String.format("%-" + ALIGNMENT + "s", valueSegments[0])).append(LINE_DELIM);
for (int i = 1; i < valueSegments.length; i++) {
printPadding(tableInfo, columnWidths);
tableInfo.append(String.format("%-" + ALIGNMENT + "s", valueSegments[i]))
.append(LINE_DELIM);
}
}
}
private static void printPadding(StringBuilder tableInfo, int[] columnWidths) {
for (int columnWidth : columnWidths) {
if (columnWidth == 0) {
tableInfo.append(FIELD_DELIM);
} else {
tableInfo.append(String.format("%" + columnWidth + "s" + FIELD_DELIM, ""));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy