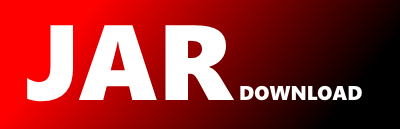
org.apache.calcite.runtime.ResultSetEnumerable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of calcite-core Show documentation
Show all versions of calcite-core Show documentation
Core Calcite APIs and engine.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.calcite.runtime;
import org.apache.calcite.linq4j.AbstractEnumerable;
import org.apache.calcite.linq4j.Enumerable;
import org.apache.calcite.linq4j.Enumerator;
import org.apache.calcite.linq4j.Linq4j;
import org.apache.calcite.linq4j.function.Function0;
import org.apache.calcite.linq4j.function.Function1;
import org.apache.calcite.linq4j.tree.Primitive;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.sql.Statement;
import java.sql.Types;
import java.util.ArrayList;
import java.util.List;
import javax.sql.DataSource;
/**
* Executes a SQL statement and returns the result as an {@link Enumerable}.
*
* @param Element type
*/
public class ResultSetEnumerable extends AbstractEnumerable {
private final DataSource dataSource;
private final String sql;
private final Function1> rowBuilderFactory;
private static final Logger LOGGER = LoggerFactory.getLogger(
ResultSetEnumerable.class);
private static final Function1> AUTO_ROW_BUILDER_FACTORY =
resultSet -> {
final ResultSetMetaData metaData;
final int columnCount;
try {
metaData = resultSet.getMetaData();
columnCount = metaData.getColumnCount();
} catch (SQLException e) {
throw new RuntimeException(e);
}
if (columnCount == 1) {
return () -> {
try {
return resultSet.getObject(1);
} catch (SQLException e) {
throw new RuntimeException(e);
}
};
} else {
//noinspection unchecked
return (Function0) () -> {
try {
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy