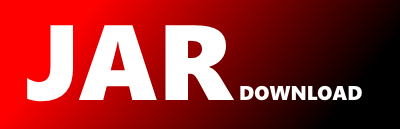
org.apache.calcite.runtime.CalciteResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of calcite-core Show documentation
Show all versions of calcite-core Show documentation
Core Calcite APIs and engine.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.calcite.runtime;
import org.apache.calcite.sql.validate.SqlValidatorException;
import static org.apache.calcite.runtime.Resources.BaseMessage;
import static org.apache.calcite.runtime.Resources.ExInst;
import static org.apache.calcite.runtime.Resources.ExInstWithCause;
import static org.apache.calcite.runtime.Resources.Inst;
import static org.apache.calcite.runtime.Resources.Property;
/**
* Compiler-checked resources for the Calcite project.
*/
public interface CalciteResource {
@BaseMessage("line {0,number,#}, column {1,number,#}")
Inst parserContext(int a0, int a1);
@BaseMessage("Bang equal ''!='' is not allowed under the current SQL conformance level")
ExInst bangEqualNotAllowed();
@BaseMessage("Percent remainder ''%'' is not allowed under the current SQL conformance level")
ExInst percentRemainderNotAllowed();
@BaseMessage("''LIMIT start, count'' is not allowed under the current SQL conformance level")
ExInst limitStartCountNotAllowed();
@BaseMessage("APPLY operator is not allowed under the current SQL conformance level")
ExInst applyNotAllowed();
@BaseMessage("Illegal {0} literal {1}: {2}")
ExInst illegalLiteral(String a0, String a1, String a2);
@BaseMessage("Length of identifier ''{0}'' must be less than or equal to {1,number,#} characters")
ExInst identifierTooLong(String a0, int a1);
@BaseMessage("not in format ''{0}''")
Inst badFormat(String a0);
@BaseMessage("BETWEEN operator has no terminating AND")
ExInst betweenWithoutAnd();
@BaseMessage("Geo-spatial extensions and the GEOMETRY data type are not enabled")
ExInst geometryDisabled();
@BaseMessage("Illegal INTERVAL literal {0}; at {1}")
@Property(name = "SQLSTATE", value = "42000")
ExInst illegalIntervalLiteral(String a0, String a1);
@BaseMessage("Illegal expression. Was expecting \"(DATETIME - DATETIME) INTERVALQUALIFIER\"")
ExInst illegalMinusDate();
@BaseMessage("Illegal overlaps expression. Was expecting expression on the form \"(DATETIME, EXPRESSION) OVERLAPS (DATETIME, EXPRESSION)\"")
ExInst illegalOverlaps();
@BaseMessage("Non-query expression encountered in illegal context")
ExInst illegalNonQueryExpression();
@BaseMessage("Query expression encountered in illegal context")
ExInst illegalQueryExpression();
@BaseMessage("CURSOR expression encountered in illegal context")
ExInst illegalCursorExpression();
@BaseMessage("ORDER BY unexpected")
ExInst illegalOrderBy();
@BaseMessage("Illegal binary string {0}")
ExInst illegalBinaryString(String a0);
@BaseMessage("''FROM'' without operands preceding it is illegal")
ExInst illegalFromEmpty();
@BaseMessage("ROW expression encountered in illegal context")
ExInst illegalRowExpression();
@BaseMessage("TABLESAMPLE percentage must be between 0 and 100, inclusive")
@Property(name = "SQLSTATE", value = "2202H")
ExInst invalidSampleSize();
@BaseMessage("Unknown character set ''{0}''")
ExInst unknownCharacterSet(String a0);
@BaseMessage("Failed to encode ''{0}'' in character set ''{1}''")
ExInst charsetEncoding(String a0, String a1);
@BaseMessage("UESCAPE ''{0}'' must be exactly one character")
ExInst unicodeEscapeCharLength(String a0);
@BaseMessage("UESCAPE ''{0}'' may not be hex digit, whitespace, plus sign, or double quote")
ExInst unicodeEscapeCharIllegal(String a0);
@BaseMessage("UESCAPE cannot be specified without Unicode literal introducer")
ExInst unicodeEscapeUnexpected();
@BaseMessage("Unicode escape sequence starting at character {0,number,#} is not exactly four hex digits")
ExInst unicodeEscapeMalformed(int a0);
@BaseMessage("No match found for function signature {0}")
ExInst validatorUnknownFunction(String a0);
@BaseMessage("Invalid number of arguments to function ''{0}''. Was expecting {1,number,#} arguments")
ExInst invalidArgCount(String a0, int a1);
@BaseMessage("At line {0,number,#}, column {1,number,#}")
ExInstWithCause validatorContextPoint(int a0,
int a1);
@BaseMessage("From line {0,number,#}, column {1,number,#} to line {2,number,#}, column {3,number,#}")
ExInstWithCause validatorContext(int a0, int a1,
int a2,
int a3);
@BaseMessage("Cast function cannot convert value of type {0} to type {1}")
ExInst cannotCastValue(String a0, String a1);
@BaseMessage("Unknown datatype name ''{0}''")
ExInst unknownDatatypeName(String a0);
@BaseMessage("Values passed to {0} operator must have compatible types")
ExInst incompatibleValueType(String a0);
@BaseMessage("Values in expression list must have compatible types")
ExInst incompatibleTypesInList();
@BaseMessage("Cannot apply {0} to the two different charsets {1} and {2}")
ExInst incompatibleCharset(String a0, String a1,
String a2);
@BaseMessage("ORDER BY is only allowed on top-level SELECT")
ExInst invalidOrderByPos();
@BaseMessage("Unknown identifier ''{0}''")
ExInst unknownIdentifier(String a0);
@BaseMessage("Unknown field ''{0}''")
ExInst unknownField(String a0);
@BaseMessage("Unknown target column ''{0}''")
ExInst unknownTargetColumn(String a0);
@BaseMessage("Target column ''{0}'' is assigned more than once")
ExInst duplicateTargetColumn(String a0);
@BaseMessage("Number of INSERT target columns ({0,number}) does not equal number of source items ({1,number})")
ExInst unmatchInsertColumn(int a0, int a1);
@BaseMessage("Column ''{0}'' has no default value and does not allow NULLs")
ExInst columnNotNullable(String a0);
@BaseMessage("Cannot assign to target field ''{0}'' of type {1} from source field ''{2}'' of type {3}")
ExInst typeNotAssignable(String a0, String a1,
String a2, String a3);
@BaseMessage("Table ''{0}'' not found")
ExInst tableNameNotFound(String a0);
@BaseMessage("Table ''{0}'' not found; did you mean ''{1}''?")
ExInst tableNameNotFoundDidYouMean(String a0,
String a1);
/** Same message as {@link #tableNameNotFound(String)} but a different kind
* of exception, so it can be used in {@code RelBuilder}. */
@BaseMessage("Table ''{0}'' not found")
ExInst tableNotFound(String tableName);
@BaseMessage("Object ''{0}'' not found")
ExInst objectNotFound(String a0);
@BaseMessage("Object ''{0}'' not found within ''{1}''")
ExInst objectNotFoundWithin(String a0, String a1);
@BaseMessage("Object ''{0}'' not found; did you mean ''{1}''?")
ExInst objectNotFoundDidYouMean(String a0, String a1);
@BaseMessage("Object ''{0}'' not found within ''{1}''; did you mean ''{2}''?")
ExInst objectNotFoundWithinDidYouMean(String a0,
String a1, String a2);
@BaseMessage("Table ''{0}'' is not a sequence")
ExInst notASequence(String a0);
@BaseMessage("Column ''{0}'' not found in any table")
ExInst columnNotFound(String a0);
@BaseMessage("Column ''{0}'' not found in any table; did you mean ''{1}''?")
ExInst columnNotFoundDidYouMean(String a0, String a1);
@BaseMessage("Column ''{0}'' not found in table ''{1}''")
ExInst columnNotFoundInTable(String a0, String a1);
@BaseMessage("Column ''{0}'' not found in table ''{1}''; did you mean ''{2}''?")
ExInst columnNotFoundInTableDidYouMean(String a0,
String a1, String a2);
@BaseMessage("Column ''{0}'' is ambiguous")
ExInst columnAmbiguous(String a0);
@BaseMessage("Operand {0} must be a query")
ExInst needQueryOp(String a0);
@BaseMessage("Parameters must be of the same type")
ExInst needSameTypeParameter();
@BaseMessage("Cannot apply ''{0}'' to arguments of type {1}. Supported form(s): {2}")
ExInst canNotApplyOp2Type(String a0, String a1,
String a2);
@BaseMessage("Expected a boolean type")
ExInst expectedBoolean();
@BaseMessage("ELSE clause or at least one THEN clause must be non-NULL")
ExInst mustNotNullInElse();
@BaseMessage("Function ''{0}'' is not defined")
ExInst functionUndefined(String a0);
@BaseMessage("Encountered {0} with {1,number} parameter(s); was expecting {2}")
ExInst wrongNumberOfParam(String a0, int a1,
String a2);
@BaseMessage("Illegal mixing of types in CASE or COALESCE statement")
ExInst illegalMixingOfTypes();
@BaseMessage("Invalid compare. Comparing (collation, coercibility): ({0}, {1} with ({2}, {3}) is illegal")
ExInst invalidCompare(String a0, String a1, String a2,
String a3);
@BaseMessage("Invalid syntax. Two explicit different collations ({0}, {1}) are illegal")
ExInst differentCollations(String a0, String a1);
@BaseMessage("{0} is not comparable to {1}")
ExInst typeNotComparable(String a0, String a1);
@BaseMessage("Cannot compare values of types ''{0}'', ''{1}''")
ExInst typeNotComparableNear(String a0, String a1);
@BaseMessage("Wrong number of arguments to expression")
ExInst wrongNumOfArguments();
@BaseMessage("Operands {0} not comparable to each other")
ExInst operandNotComparable(String a0);
@BaseMessage("Types {0} not comparable to each other")
ExInst typeNotComparableEachOther(String a0);
@BaseMessage("Numeric literal ''{0}'' out of range")
ExInst numberLiteralOutOfRange(String a0);
@BaseMessage("Date literal ''{0}'' out of range")
ExInst dateLiteralOutOfRange(String a0);
@BaseMessage("String literal continued on same line")
ExInst stringFragsOnSameLine();
@BaseMessage("Table or column alias must be a simple identifier")
ExInst aliasMustBeSimpleIdentifier();
@BaseMessage("List of column aliases must have same degree as table; table has {0,number,#} columns {1}, whereas alias list has {2,number,#} columns")
ExInst aliasListDegree(int a0, String a1, int a2);
@BaseMessage("Duplicate name ''{0}'' in column alias list")
ExInst aliasListDuplicate(String a0);
@BaseMessage("INNER, LEFT, RIGHT or FULL join requires a condition (NATURAL keyword or ON or USING clause)")
ExInst joinRequiresCondition();
@BaseMessage("Cannot specify condition (NATURAL keyword, or ON or USING clause) following CROSS JOIN")
ExInst crossJoinDisallowsCondition();
@BaseMessage("Cannot specify NATURAL keyword with ON or USING clause")
ExInst naturalDisallowsOnOrUsing();
@BaseMessage("Column name ''{0}'' in USING clause is not unique on one side of join")
ExInst columnInUsingNotUnique(String a0);
@BaseMessage("Column ''{0}'' matched using NATURAL keyword or USING clause has incompatible types: cannot compare ''{1}'' to ''{2}''")
ExInst naturalOrUsingColumnNotCompatible(String a0,
String a1, String a2);
@BaseMessage("OVER clause is necessary for window functions")
ExInst absentOverClause();
@BaseMessage("Window ''{0}'' not found")
ExInst windowNotFound(String a0);
@BaseMessage("Expression ''{0}'' is not being grouped")
ExInst notGroupExpr(String a0);
@BaseMessage("Argument to {0} operator must be a grouped expression")
ExInst groupingArgument(String a0);
@BaseMessage("{0} operator may only occur in an aggregate query")
ExInst groupingInAggregate(String a0);
@BaseMessage("{0} operator may only occur in SELECT, HAVING or ORDER BY clause")
ExInst groupingInWrongClause(String a0);
@BaseMessage("Expression ''{0}'' is not in the select clause")
ExInst notSelectDistinctExpr(String a0);
@BaseMessage("Aggregate expression is illegal in {0} clause")
ExInst aggregateIllegalInClause(String a0);
@BaseMessage("Windowed aggregate expression is illegal in {0} clause")
ExInst windowedAggregateIllegalInClause(String a0);
@BaseMessage("Aggregate expressions cannot be nested")
ExInst nestedAggIllegal();
@BaseMessage("FILTER must not contain aggregate expression")
ExInst aggregateInFilterIllegal();
@BaseMessage("Aggregate expression is illegal in ORDER BY clause of non-aggregating SELECT")
ExInst aggregateIllegalInOrderBy();
@BaseMessage("{0} clause must be a condition")
ExInst condMustBeBoolean(String a0);
@BaseMessage("HAVING clause must be a condition")
ExInst havingMustBeBoolean();
@BaseMessage("OVER must be applied to aggregate function")
ExInst overNonAggregate();
@BaseMessage("FILTER must be applied to aggregate function")
ExInst filterNonAggregate();
@BaseMessage("Cannot override window attribute")
ExInst cannotOverrideWindowAttribute();
@BaseMessage("Column count mismatch in {0}")
ExInst columnCountMismatchInSetop(String a0);
@BaseMessage("Type mismatch in column {0,number} of {1}")
ExInst columnTypeMismatchInSetop(int a0, String a1);
@BaseMessage("Binary literal string must contain an even number of hexits")
ExInst binaryLiteralOdd();
@BaseMessage("Binary literal string must contain only characters ''0'' - ''9'', ''A'' - ''F''")
ExInst binaryLiteralInvalid();
@BaseMessage("Illegal interval literal format {0} for {1}")
ExInst unsupportedIntervalLiteral(String a0,
String a1);
@BaseMessage("Interval field value {0,number} exceeds precision of {1} field")
ExInst intervalFieldExceedsPrecision(Number a0,
String a1);
@BaseMessage("RANGE clause cannot be used with compound ORDER BY clause")
ExInst compoundOrderByProhibitsRange();
@BaseMessage("Data type of ORDER BY prohibits use of RANGE clause")
ExInst orderByDataTypeProhibitsRange();
@BaseMessage("Data Type mismatch between ORDER BY and RANGE clause")
ExInst orderByRangeMismatch();
@BaseMessage("Window ORDER BY expression of type DATE requires range of type INTERVAL")
ExInst dateRequiresInterval();
@BaseMessage("ROWS value must be a non-negative integral constant")
ExInst rowMustBeNonNegativeIntegral();
@BaseMessage("Window specification must contain an ORDER BY clause")
ExInst overMissingOrderBy();
@BaseMessage("PARTITION BY expression should not contain OVER clause")
ExInst partitionbyShouldNotContainOver();
@BaseMessage("ORDER BY expression should not contain OVER clause")
ExInst orderbyShouldNotContainOver();
@BaseMessage("UNBOUNDED FOLLOWING cannot be specified for the lower frame boundary")
ExInst badLowerBoundary();
@BaseMessage("UNBOUNDED PRECEDING cannot be specified for the upper frame boundary")
ExInst badUpperBoundary();
@BaseMessage("Upper frame boundary cannot be PRECEDING when lower boundary is CURRENT ROW")
ExInst currentRowPrecedingError();
@BaseMessage("Upper frame boundary cannot be CURRENT ROW when lower boundary is FOLLOWING")
ExInst currentRowFollowingError();
@BaseMessage("Upper frame boundary cannot be PRECEDING when lower boundary is FOLLOWING")
ExInst followingBeforePrecedingError();
@BaseMessage("Window name must be a simple identifier")
ExInst windowNameMustBeSimple();
@BaseMessage("Duplicate window names not allowed")
ExInst duplicateWindowName();
@BaseMessage("Empty window specification not allowed")
ExInst emptyWindowSpec();
@BaseMessage("Duplicate window specification not allowed in the same window clause")
ExInst dupWindowSpec();
@BaseMessage("ROW/RANGE not allowed with RANK, DENSE_RANK or ROW_NUMBER functions")
ExInst rankWithFrame();
@BaseMessage("RANK or DENSE_RANK functions require ORDER BY clause in window specification")
ExInst funcNeedsOrderBy();
@BaseMessage("PARTITION BY not allowed with existing window reference")
ExInst partitionNotAllowed();
@BaseMessage("ORDER BY not allowed in both base and referenced windows")
ExInst orderByOverlap();
@BaseMessage("Referenced window cannot have framing declarations")
ExInst refWindowWithFrame();
@BaseMessage("Type ''{0}'' is not supported")
ExInst typeNotSupported(String a0);
@BaseMessage("DISTINCT/ALL not allowed with {0} function")
ExInst functionQuantifierNotAllowed(String a0);
@BaseMessage("Some but not all arguments are named")
ExInst someButNotAllArgumentsAreNamed();
@BaseMessage("Duplicate argument name ''{0}''")
ExInst duplicateArgumentName(String name);
@BaseMessage("DEFAULT is only allowed for optional parameters")
ExInst defaultForOptionalParameter();
@BaseMessage("DEFAULT not allowed here")
ExInst defaultNotAllowed();
@BaseMessage("Not allowed to perform {0} on {1}")
ExInst accessNotAllowed(String a0, String a1);
@BaseMessage("The {0} function does not support the {1} data type.")
ExInst minMaxBadType(String a0, String a1);
@BaseMessage("Only scalar sub-queries allowed in select list.")
ExInst onlyScalarSubQueryAllowed();
@BaseMessage("Ordinal out of range")
ExInst orderByOrdinalOutOfRange();
@BaseMessage("Window has negative size")
ExInst windowHasNegativeSize();
@BaseMessage("UNBOUNDED FOLLOWING window not supported")
ExInst unboundedFollowingWindowNotSupported();
@BaseMessage("Cannot use DISALLOW PARTIAL with window based on RANGE")
ExInst cannotUseDisallowPartialWithRange();
@BaseMessage("Interval leading field precision ''{0,number,#}'' out of range for {1}")
ExInst intervalStartPrecisionOutOfRange(int a0,
String a1);
@BaseMessage("Interval fractional second precision ''{0,number,#}'' out of range for {1}")
ExInst intervalFractionalSecondPrecisionOutOfRange(
int a0, String a1);
@BaseMessage("Duplicate relation name ''{0}'' in FROM clause")
ExInst fromAliasDuplicate(String a0);
@BaseMessage("Duplicate column name ''{0}'' in output")
ExInst duplicateColumnName(String a0);
@BaseMessage("Duplicate name ''{0}'' in column list")
ExInst duplicateNameInColumnList(String a0);
@BaseMessage("Internal error: {0}")
ExInst internal(String a0);
@BaseMessage("Argument to function ''{0}'' must be a literal")
ExInst argumentMustBeLiteral(String a0);
@BaseMessage("Argument to function ''{0}'' must be a positive integer literal")
ExInst argumentMustBePositiveInteger(String a0);
@BaseMessage("Validation Error: {0}")
ExInst validationError(String a0);
@BaseMessage("Locale ''{0}'' in an illegal format")
ExInst illegalLocaleFormat(String a0);
@BaseMessage("Argument to function ''{0}'' must not be NULL")
ExInst argumentMustNotBeNull(String a0);
@BaseMessage("Illegal use of ''NULL''")
ExInst nullIllegal();
@BaseMessage("Illegal use of dynamic parameter")
ExInst dynamicParamIllegal();
@BaseMessage("''{0}'' is not a valid boolean value")
ExInst invalidBoolean(String a0);
@BaseMessage("Argument to function ''{0}'' must be a valid precision between ''{1,number,#}'' and ''{2,number,#}''")
ExInst argumentMustBeValidPrecision(String a0, int a1,
int a2);
@BaseMessage("Wrong arguments for table function ''{0}'' call. Expected ''{1}'', actual ''{2}''")
ExInst illegalArgumentForTableFunctionCall(String a0,
String a1, String a2);
@BaseMessage("''{0}'' is not a valid datetime format")
ExInst invalidDatetimeFormat(String a0);
@BaseMessage("Cannot INSERT into generated column ''{0}''")
ExInst insertIntoAlwaysGenerated(String a0);
@BaseMessage("Argument to function ''{0}'' must have a scale of 0")
ExInst argumentMustHaveScaleZero(String a0);
@BaseMessage("Statement preparation aborted")
ExInst preparationAborted();
@BaseMessage("SELECT DISTINCT not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_E051_01();
@BaseMessage("EXCEPT not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_E071_03();
@BaseMessage("UPDATE not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_E101_03();
@BaseMessage("Transactions not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_E151();
@BaseMessage("INTERSECT not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_F302();
@BaseMessage("MERGE not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_F312();
@BaseMessage("Basic multiset not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_S271();
@BaseMessage("TABLESAMPLE not supported")
@Property(name = "FeatureDefinition", value = "SQL:2003 Part 2 Annex F")
Feature sQLFeature_T613();
@BaseMessage("Execution of a new autocommit statement while a cursor is still open on same connection is not supported")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
ExInst sQLConformance_MultipleActiveAutocommitStatements();
@BaseMessage("Descending sort (ORDER BY DESC) not supported")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
Feature sQLConformance_OrderByDesc();
@BaseMessage("Sharing of cached statement plans not supported")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
ExInst sharedStatementPlans();
@BaseMessage("TABLESAMPLE SUBSTITUTE not supported")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
Feature sQLFeatureExt_T613_Substitution();
@BaseMessage("Personality does not maintain table''s row count in the catalog")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
ExInst personalityManagesRowCount();
@BaseMessage("Personality does not support snapshot reads")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
ExInst personalitySupportsSnapshots();
@BaseMessage("Personality does not support labels")
@Property(name = "FeatureDefinition", value = "Eigenbase-defined")
ExInst personalitySupportsLabels();
@BaseMessage("Require at least 1 argument")
ExInst requireAtLeastOneArg();
@BaseMessage("Map requires at least 2 arguments")
ExInst mapRequiresTwoOrMoreArgs();
@BaseMessage("Map requires an even number of arguments")
ExInst mapRequiresEvenArgCount();
@BaseMessage("Incompatible types")
ExInst incompatibleTypes();
@BaseMessage("Number of columns must match number of query columns")
ExInst columnCountMismatch();
@BaseMessage("Column has duplicate column name ''{0}'' and no column list specified")
ExInst duplicateColumnAndNoColumnList(String s);
@BaseMessage("Declaring class ''{0}'' of non-static user-defined function must have a public constructor with zero parameters")
ExInst requireDefaultConstructor(String className);
@BaseMessage("In user-defined aggregate class ''{0}'', first parameter to ''add'' method must be the accumulator (the return type of the ''init'' method)")
ExInst firstParameterOfAdd(String className);
@BaseMessage("FilterableTable.scan returned a filter that was not in the original list: {0}")
ExInst filterableTableInventedFilter(String s);
@BaseMessage("FilterableTable.scan must not return null")
ExInst filterableTableScanReturnedNull();
@BaseMessage("Cannot convert table ''{0}'' to stream")
ExInst cannotConvertToStream(String tableName);
@BaseMessage("Cannot convert stream ''{0}'' to relation")
ExInst cannotConvertToRelation(String tableName);
@BaseMessage("Streaming aggregation requires at least one monotonic expression in GROUP BY clause")
ExInst streamMustGroupByMonotonic();
@BaseMessage("Streaming ORDER BY must start with monotonic expression")
ExInst streamMustOrderByMonotonic();
@BaseMessage("Set operator cannot combine streaming and non-streaming inputs")
ExInst streamSetOpInconsistentInputs();
@BaseMessage("Cannot stream VALUES")
ExInst cannotStreamValues();
@BaseMessage("Cannot resolve ''{0}''; it references view ''{1}'', whose definition is cyclic")
ExInst cyclicDefinition(String id, String view);
@BaseMessage("Modifiable view must be based on a single table")
ExInst modifiableViewMustBeBasedOnSingleTable();
@BaseMessage("Modifiable view must be predicated only on equality expressions")
ExInst modifiableViewMustHaveOnlyEqualityPredicates();
@BaseMessage("View is not modifiable. More than one expression maps to column ''{0}'' of base table ''{1}''")
ExInst moreThanOneMappedColumn(String columnName, String tableName);
@BaseMessage("View is not modifiable. No value is supplied for NOT NULL column ''{0}'' of base table ''{1}''")
ExInst noValueSuppliedForViewColumn(String columnName, String tableName);
@BaseMessage("Modifiable view constraint is not satisfied for column ''{0}'' of base table ''{1}''")
ExInst viewConstraintNotSatisfied(String columnName, String tableName);
@BaseMessage("Not a record type. The ''*'' operator requires a record")
ExInst starRequiresRecordType();
@BaseMessage("FILTER expression must be of type BOOLEAN")
ExInst filterMustBeBoolean();
@BaseMessage("Cannot stream results of a query with no streaming inputs: ''{0}''. At least one input should be convertible to a stream")
ExInst cannotStreamResultsForNonStreamingInputs(String inputs);
@BaseMessage("MINUS is not allowed under the current SQL conformance level")
ExInst minusNotAllowed();
@BaseMessage("SELECT must have a FROM clause")
ExInst selectMissingFrom();
@BaseMessage("Group function ''{0}'' can only appear in GROUP BY clause")
ExInst groupFunctionMustAppearInGroupByClause(String funcName);
@BaseMessage("Call to auxiliary group function ''{0}'' must have matching call to group function ''{1}'' in GROUP BY clause")
ExInst auxiliaryWithoutMatchingGroupCall(String func1, String func2);
@BaseMessage("Pattern variable ''{0}'' has already been defined")
ExInst patternVarAlreadyDefined(String varName);
@BaseMessage("Cannot use PREV/NEXT in MEASURE ''{0}''")
ExInst patternPrevFunctionInMeasure(String call);
@BaseMessage("Cannot nest PREV/NEXT under LAST/FIRST ''{0}''")
ExInst patternPrevFunctionOrder(String call);
@BaseMessage("Cannot use aggregation in navigation ''{0}''")
ExInst patternAggregationInNavigation(String call);
@BaseMessage("Invalid number of parameters to COUNT method")
ExInst patternCountFunctionArg();
@BaseMessage("The system time period specification expects Timestamp type but is ''{0}''")
ExInst illegalExpressionForTemporal(String type);
@BaseMessage("Table ''{0}'' is not a temporal table, can not be queried in system time period specification")
ExInst notTemporalTable(String tableName);
@BaseMessage("Cannot use RUNNING/FINAL in DEFINE ''{0}''")
ExInst patternRunningFunctionInDefine(String call);
@BaseMessage("Multiple pattern variables in ''{0}''")
ExInst patternFunctionVariableCheck(String call);
@BaseMessage("Function ''{0}'' can only be used in MATCH_RECOGNIZE")
ExInst functionMatchRecognizeOnly(String call);
@BaseMessage("Null parameters in ''{0}''")
ExInst patternFunctionNullCheck(String call);
@BaseMessage("Unknown pattern ''{0}''")
ExInst unknownPattern(String call);
@BaseMessage("Interval must be non-negative ''{0}''")
ExInst intervalMustBeNonNegative(String call);
@BaseMessage("Must contain an ORDER BY clause when WITHIN is used")
ExInst cannotUseWithinWithoutOrderBy();
@BaseMessage("First column of ORDER BY must be of type TIMESTAMP")
ExInst firstColumnOfOrderByMustBeTimestamp();
@BaseMessage("Extended columns not allowed under the current SQL conformance level")
ExInst extendNotAllowed();
@BaseMessage("Rolled up column ''{0}'' is not allowed in {1}")
ExInst rolledUpNotAllowed(String column, String context);
@BaseMessage("Schema ''{0}'' already exists")
ExInst schemaExists(String name);
@BaseMessage("Invalid schema type ''{0}''; valid values: {1}")
ExInst schemaInvalidType(String type, String values);
@BaseMessage("Table ''{0}'' already exists")
ExInst tableExists(String name);
// If CREATE TABLE does not have "AS query", there must be a column list
@BaseMessage("Missing column list")
ExInst createTableRequiresColumnList();
// If CREATE TABLE does not have "AS query", a type must be specified for each
// column
@BaseMessage("Type required for column ''{0}'' in CREATE TABLE without AS")
ExInst createTableRequiresColumnTypes(String columnName);
@BaseMessage("View ''{0}'' already exists and REPLACE not specified")
ExInst viewExists(String name);
@BaseMessage("Schema ''{0}'' not found")
ExInst schemaNotFound(String name);
@BaseMessage("View ''{0}'' not found")
ExInst viewNotFound(String name);
@BaseMessage("Type ''{0}'' not found")
ExInst typeNotFound(String name);
@BaseMessage("Dialect does not support feature: ''{0}''")
ExInst dialectDoesNotSupportFeature(String featureName);
}
// End CalciteResource.java
© 2015 - 2024 Weber Informatics LLC | Privacy Policy