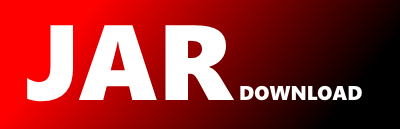
com.alibaba.antx.util.configuration.Configuration Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2012 Alibaba Group Holding Limited.
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.antx.util.configuration;
public interface Configuration {
/**
* Return the name of the node.
*
* @return name of the Configuration
node.
*/
String getName();
/**
* Return a string describing location of Configuration. Location can be
* different for different mediums (ie "file:line" for normal XML files or
* "table:primary-key" for DB based configurations);
*
* @return a string describing location of Configuration
*/
Location getLocation();
/**
* Returns a string indicating which namespace this Configuration node
* belongs to.
*
* What this returns is dependent on the configuration file and the
* Configuration builder. If the Configuration builder does not support
* namespaces, this method will return a blank string.
*
*
* In the case of {@link DefaultConfigurationBuilder}, the namespace will be
* the URI associated with the XML element. Eg.,:
*
*
*
* <foo xmlns:x="http://blah.com">
* <x:bar/>
* </foo>
*
*
* The namespace of foo
will be "", and the namespace of
* bar
will be "http://blah.com".
*
*
* @return a String identifying the namespace of this Configuration.
* @throws ConfigurationException if an error occurs
* @since 4.1
*/
String getNamespace() throws ConfigurationException;
/**
* Return a new Configuration
instance encapsulating the
* specified child node.
*
* If no such child node exists, an empty Configuration
will be
* returned, allowing constructs such as
* conf.getChild("foo").getChild("bar").getChild("baz").{@link
* #getValue(String) getValue}("default");
*
*
* If you wish to get a null
return when no element is present,
* use {@link #getChild(String, boolean) getChild("foo", false)}.
*
*
* @param child The name of the child node.
* @return Configuration
*/
Configuration getChild(String child);
/**
* Return a Configuration
instance encapsulating the specified
* child node.
*
* @param child The name of the child node.
* @param createNew If true
, a new Configuration
* will be created and returned if the specified child does not
* exist. If false
, null
will be
* returned when the specified child doesn't exist.
* @return Configuration
*/
Configuration getChild(String child, boolean createNew);
/**
* Return an Array
of Configuration
elements
* containing all node children. The array order will reflect the order in
* the source config file.
*
* @return All child nodes
*/
Configuration[] getChildren();
/**
* Return an Array
of Configuration
elements
* containing all node children with the specified name. The array order
* will reflect the order in the source config file.
*
* @param name The name of the children to get.
* @return The child nodes with name name
*/
Configuration[] getChildren(String name);
/**
* Return an array of all attribute names.
*
* The order of attributes in this array can not be relied on. As
* with XML, a Configuration
's attributes are an
* unordered set. If your code relies on order, eg
* conf.getAttributeNames()[0], then it is liable to break if a
* different XML parser is used.
*
*
* @return a String[]
value
*/
String[] getAttributeNames();
/**
* Return the value of specified attribute.
*
* @param paramName The name of the parameter you ask the value of.
* @return String value of attribute.
* @throws ConfigurationException If no attribute with that name exists.
*/
String getAttribute(String paramName) throws ConfigurationException;
/**
* Return the int
value of the specified attribute contained in
* this node.
*
* @param paramName The name of the parameter you ask the value of.
* @return int value of attribute
* @throws ConfigurationException If no parameter with that name exists. or
* if conversion to int
fails.
*/
int getAttributeAsInteger(String paramName) throws ConfigurationException;
/**
* Returns the value of the attribute specified by its name as a
* long
.
*
* @param name The name of the parameter you ask the value of.
* @return long value of attribute
* @throws ConfigurationException If no parameter with that name exists. or
* if conversion to long
fails.
*/
long getAttributeAsLong(String name) throws ConfigurationException;
/**
* Return the float
value of the specified parameter contained
* in this node.
*
* @param paramName The name of the parameter you ask the value of.
* @return float value of attribute
* @throws ConfigurationException If no parameter with that name exists. or
* if conversion to float
fails.
*/
float getAttributeAsFloat(String paramName) throws ConfigurationException;
/**
* Return the boolean
value of the specified parameter
* contained in this node.
*
* @param paramName The name of the parameter you ask the value of.
* @return boolean value of attribute
* @throws ConfigurationException If no parameter with that name exists. or
* if conversion to boolean
fails.
*/
boolean getAttributeAsBoolean(String paramName) throws ConfigurationException;
/**
* Return the String
value of the node.
*
* @return the value of the node.
* @throws ConfigurationException if an error occurs
*/
String getValue() throws ConfigurationException;
/**
* Return the int
value of the node.
*
* @return the value of the node.
* @throws ConfigurationException If conversion to int
fails.
*/
int getValueAsInteger() throws ConfigurationException;
/**
* Return the float
value of the node.
*
* @return the value of the node.
* @throws ConfigurationException If conversion to float
fails.
*/
float getValueAsFloat() throws ConfigurationException;
/**
* Return the boolean
value of the node.
*
* @return the value of the node.
* @throws ConfigurationException If conversion to boolean
* fails.
*/
boolean getValueAsBoolean() throws ConfigurationException;
/**
* Return the long
value of the node.
*
* @return the value of the node.
* @throws ConfigurationException If conversion to long
fails.
*/
long getValueAsLong() throws ConfigurationException;
/**
* Returns the value of the configuration element as a String
.
* If the configuration value is not set, the default value will be used.
*
* @param defaultValue The default value desired.
* @return String value of the Configuration
, or default if none
* specified.
*/
String getValue(String defaultValue);
/**
* Returns the value of the configuration element as an int
. If
* the configuration value is not set, the default value will be used.
*
* @param defaultValue The default value desired.
* @return int value of the Configuration
, or default if none
* specified.
*/
int getValueAsInteger(int defaultValue);
/**
* Returns the value of the configuration element as a long
. If
* the configuration value is not set, the default value will be used.
*
* @param defaultValue The default value desired.
* @return long value of the Configuration
, or default if none
* specified.
*/
long getValueAsLong(long defaultValue);
/**
* Returns the value of the configuration element as a float
.
* If the configuration value is not set, the default value will be used.
*
* @param defaultValue The default value desired.
* @return float value of the Configuration
, or default if none
* specified.
*/
float getValueAsFloat(float defaultValue);
/**
* Returns the value of the configuration element as a boolean
.
* If the configuration value is not set, the default value will be used.
*
* @param defaultValue The default value desired.
* @return boolean value of the Configuration
, or default if
* none specified.
*/
boolean getValueAsBoolean(boolean defaultValue);
/**
* Returns the value of the attribute specified by its name as a
* String
, or the default value if no attribute by that name
* exists or is empty.
*
* @param name The name of the attribute you ask the value of.
* @param defaultValue The default value desired.
* @return String value of attribute. It will return the default value if the
* named attribute does not exist, or if the value is not set.
*/
String getAttribute(String name, String defaultValue);
/**
* Returns the value of the attribute specified by its name as a
* int
, or the default value if no attribute by that name
* exists or is empty.
*
* @param name The name of the attribute you ask the value of.
* @param defaultValue The default value desired.
* @return int value of attribute. It will return the default value if the
* named attribute does not exist, or if the value is not set.
*/
int getAttributeAsInteger(String name, int defaultValue);
/**
* Returns the value of the attribute specified by its name as a
* long
, or the default value if no attribute by that name
* exists or is empty.
*
* @param name The name of the attribute you ask the value of.
* @param defaultValue The default value desired.
* @return long value of attribute. It will return the default value if the
* named attribute does not exist, or if the value is not set.
*/
long getAttributeAsLong(String name, long defaultValue);
/**
* Returns the value of the attribute specified by its name as a
* float
, or the default value if no attribute by that name
* exists or is empty.
*
* @param name The name of the attribute you ask the value of.
* @param defaultValue The default value desired.
* @return float value of attribute. It will return the default value if the
* named attribute does not exist, or if the value is not set.
*/
float getAttributeAsFloat(String name, float defaultValue);
/**
* Returns the value of the attribute specified by its name as a
* boolean
, or the default value if no attribute by that name
* exists or is empty.
*
* @param name The name of the attribute you ask the value of.
* @param defaultValue The default value desired.
* @return boolean value of attribute. It will return the default value if
* the named attribute does not exist, or if the value is not set.
*/
boolean getAttributeAsBoolean(String name, boolean defaultValue);
}