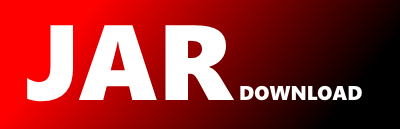
com.alibaba.toolkit.util.collection.ListMap Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2002-2012 Alibaba Group Holding Limited.
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.toolkit.util.collection;
import java.util.List;
import java.util.Map;
/**
*
* 有序的java.util.Map
.
*
*
* 除了拥有java.util.Map
的所有特性以外, ListMap
中的 项(
* Map.Entry
)是有序的. 也就是说, 它既能以键值(key)来访问, 也可以用索引(index)来访问. 例如,
*
*
* 通过key访问:
*
*
*
* Object value1 = listMap.get("key1");
*
*
* 通过整数index, 取得key和value:
*
*
*
* Object value2 = listMap.get(2);
* Object key2 = listMap.getKey(2);
*
*
* 通过整数index, 删除一项, 并返回被删除的项:
*
*
*
* Map.Entry removedEntry = listMap.remove(3);
*
*
* 此外, 它还提供了三个方法: keyList()
, valueList()
和
* entryList()
, 用来取得key, value和entry的List
. 相对于
* Map.keySet()
, Map.values()
以及
* Map.entrySet()
, 后者只提供了取得无序的key和entry的Set
,
* 以及取得value的Collection
的方法.
*
*
* @author Michael Zhou
* @version $Id: ListMap.java,v 1.1 2003/07/03 07:26:16 baobao Exp $
*/
public interface ListMap extends Map {
/**
* 返回指定index处的value. 如果index超出范围, 则掷出IndexOutOfBoundsException
.
*
* @param index 要返回的value的索引值.
* @return 指定index处的value对象
*/
Object get(int index);
/**
* 返回指定index处的key. 如果index超出范围, 则返回IndexOutOfBoundsException
.
*
* @param index 要返回的key的索引值.
* @return 指定index处的key对象
*/
Object getKey(int index);
/**
* 删除指定index处的项. 如果index超出范围, 则返回IndexOutOfBoundsException
.
*
* @param index 要删除的项的索引值.
* @return 被删除的Map.Entry
项.
*/
Map.Entry remove(int index);
/**
* 返回所有key的List
.
*
* @return 所有key的List
.
*/
List keyList();
/**
* 返回所有value的List
.
*
* @return 所有value的List
.
*/
List valueList();
/**
* 返回所有entry的List
.
*
* @return 所有entry的List
.
*/
List entryList();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy