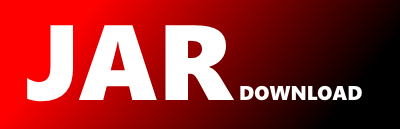
com.aliyun.LoadConfigManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-maven-plugin Show documentation
Show all versions of toolkit-maven-plugin Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun;
import com.aliyun.bean.AliyunConfigBean;
import com.aliyun.bean.DefaultConfigBean;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.project.MavenProject;
import java.io.*;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class LoadConfigManager {
private static final String ACCESS_KEY_ID = "access_key_id";
private static final String ACCESS_KEY_SECRET = "access_key_secret";
private static final String DEFAULT_CONFIG_FILE = ".edas_config.yaml";
String SEPARATOR = System.getProperty("file.separator");
private MavenProject project;
private Log logger;
private ObjectMapper yamlMapper;
private ObjectMapper jsonMapper;
public LoadConfigManager(MavenProject project, Log logger) {
this.project = project;
this.logger = logger;
//yaml
yamlMapper = new ObjectMapper(new YAMLFactory());
yamlMapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
yamlMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
// yamlMapper.enable(DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT);
//json
jsonMapper = new ObjectMapper();
jsonMapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
jsonMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
// jsonMapper.enable(DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT);
}
public DefaultConfigBean loadProperties(
String configFile,
String accessKeyFile,
String accessKeyId, String accessKeySecret) throws MojoExecutionException {
//load config
DefaultConfigBean config = loadConfigFile(configFile);
//check ak/sk
checkAndOverwriteAccessKey(config, accessKeyFile, accessKeyId, accessKeySecret);
//check param
checkParamValid(config);
//adjust properties
setDefaultParam(config);
return config;
}
private DefaultConfigBean loadConfigFile(String configFile) throws MojoExecutionException {
File file;
if (configFile != null) {
file = new File(configFile);
} else {
File baseDir = project.getBasedir();
String defaultConfigFile = baseDir.getPath() + SEPARATOR + DEFAULT_CONFIG_FILE;
file = new File(defaultConfigFile);
}
if (!file.exists()) {
throw new MojoExecutionException("No config file is found: " + file.getAbsolutePath());
}
DefaultConfigBean config;
try {
config = yamlMapper.readValue(file, DefaultConfigBean.class);
if (config.getEnv() == null) {
config.setEnv(new DefaultConfigBean.Env());
}
} catch (Exception ex) {
logger.error(ex);
throw new MojoExecutionException(ex.getMessage());
}
return config;
}
private void setDefaultParam(DefaultConfigBean config) {
//env
DefaultConfigBean.Env env = config.getEnv();
if (env.getDomain() == null) {
env.setDomain("edas." + env.getRegionId() + ".aliyuncs.com");
}
//app
DefaultConfigBean.App app = config.getApp();
if (app.getDeployType() == null) {
app.setDeployType("url");
}
if (app.getBatch() == null) {
app.setBatch(1);
}
if (app.getGroupId() == null) {
app.setGroupId("all");
}
int batchWaitTime = (app.getBatchWaitTime() != null) ? app.getBatchWaitTime() : 0;
if (app.getStageTimeout() == null) {
app.setStageTimeout(batchWaitTime * 60 + 300);
} else {
int seconds = batchWaitTime * 60 + app.getStageTimeout() * 60;
app.setStageTimeout(seconds);
}
if (app.getPackageVersion() == null) {
String pv = String.format("%s (%s)", project.getVersion(), CommonUtils.getCurrentTime());
app.setPackageVersion(pv);
}
app.setServiceStageTimeout(app.getStageTimeout());
app.setInstanceStageTimeout(app.getStageTimeout());
}
private void checkAndOverwriteAccessKey(
DefaultConfigBean config,
String accessKeyFile,
String accessKeyId, String accessKeySecret) throws MojoExecutionException {
if (loadAkSkFromCommandLine(config, accessKeyId, accessKeySecret)) {
return;
}
if (loadAkSkFromCustomFile(config, accessKeyFile)) {
return;
}
if (loadAkSkFromEdasConfig(config)) {
return;
}
if (loadAkSkFromAliyunConfig(config)) {
return;
}
if (loadAkSkFromSystemEnv(config)) {
return;
}
throw new MojoExecutionException(
String.format("'%s' or '%s' is not specified.", ACCESS_KEY_ID, ACCESS_KEY_SECRET) );
}
private boolean loadAkSkFromSystemEnv(DefaultConfigBean config) {
String ak = System.getenv(ACCESS_KEY_ID);
String sk = System.getenv(ACCESS_KEY_SECRET);
if (CommonUtils.isNotEmpty(ak) && CommonUtils.isNotEmpty(sk)) {
config.getEnv().setAccessKeyId(ak);
config.getEnv().setAccessKeySecret(sk);
logger.info("Use the ak/sk specified in system environment.");
return true;
}
return false;
}
private boolean loadAkSkFromEdasConfig(DefaultConfigBean config) {
return config.getEnv().getAccessKeyId() != null && config.getEnv().getAccessKeySecret() != null;
}
private boolean loadAkSkFromCommandLine(
DefaultConfigBean config,
String accessKeyId, String accessKeySecret) {
//use -Daccess_key_id and -Daccess_key_secret
if (accessKeyId != null && accessKeySecret != null) {
config.getEnv().setAccessKeyId(accessKeyId);
config.getEnv().setAccessKeySecret(accessKeySecret);
logger.info("Use the ak/sk specified in maven command line parameters.");
return true;
}
return false;
}
private boolean loadAkSkFromCustomFile(
DefaultConfigBean config,
String accessKeyFile) throws MojoExecutionException {
//use -Daccess_key_file=xx
try {
if (accessKeyFile != null) {
Map map = yamlMapper.readValue(new File(accessKeyFile), Map.class);
String ak = String.valueOf(map.get(ACCESS_KEY_ID));
String sk = String.valueOf(map.get(ACCESS_KEY_SECRET));
if (ak == null) {
throw new MojoExecutionException(
String.format("No '%s' is found in %s", ACCESS_KEY_ID, accessKeyFile));
}
if (sk == null) {
throw new MojoExecutionException(
String.format("No '%s' is found in %s", ACCESS_KEY_SECRET, accessKeyFile));
}
config.getEnv().setAccessKeyId(ak);
config.getEnv().setAccessKeySecret(sk);
logger.info("Use the ak/sk specified in the file: " + accessKeyFile);
return true;
}
} catch (FileNotFoundException ex) {
logger.error(ex);
throw new MojoExecutionException(accessKeyFile + " does not exists.");
} catch (Exception ex) {
logger.error(ex);
String msg = String.format("%s load failed, please confirm that it is a valid yaml file "
+ ", contains '%s' and '%s' field", accessKeyFile, ACCESS_KEY_ID, ACCESS_KEY_SECRET);
throw new MojoExecutionException(msg);
}
return false;
}
private boolean loadAkSkFromAliyunConfig(DefaultConfigBean config) throws MojoExecutionException {
String userHome = System.getProperty("user.home");
String separator = System.getProperty("file.separator");
//use ~/.aliyuncli/credentials
Pattern akPattern = Pattern.compile("aliyun_access_key_id\\s*=\\s*(.+)");
Pattern skPattern = Pattern.compile("aliyun_access_key_secret\\s*=\\s*(.+)");
String aliyunCliCredentials = userHome + separator + ".aliyuncli" + separator + "credentials";
File credentialFile = new File(aliyunCliCredentials);
BufferedReader reader = null;
if (credentialFile.exists()) {
try {
String ak = null, sk = null;
String line;
reader = new BufferedReader(new FileReader(credentialFile));
while ((line = reader.readLine()) != null) {
Matcher akMatcher = akPattern.matcher(line);
if (akMatcher.find()) {
ak = akMatcher.group(1).trim();
continue;
}
Matcher skMatcher = skPattern.matcher(line);
if (skMatcher.find()) {
sk = skMatcher.group(1).trim();
}
}
if (ak != null && sk != null) {
config.getEnv().setAccessKeyId(ak);
config.getEnv().setAccessKeySecret(sk);
logger.info("Use the ak/sk specified in the aliyuncli default credential: " + aliyunCliCredentials);
return true;
}
} catch (Exception ex) {
logger.warn(String.format("Load ak/sk from %s failed: %s", aliyunCliCredentials, ex.getMessage()));
} finally {
CommonUtils.close(reader);
}
}
//use ~/.aliyun/config.json
String aliyunConfigJson = userHome + separator + ".aliyun" + separator + "config.json";
File configJsonFile = new File(aliyunConfigJson);
if (configJsonFile.exists()) {
try {
AliyunConfigBean aliyunConfig = jsonMapper.readValue(configJsonFile, AliyunConfigBean.class);
List profiles = aliyunConfig.getProfiles();
if (profiles == null) {
return false;
}
for (AliyunConfigBean.Profile profile: profiles) {
if (CommonUtils.isNotEmpty(profile.getAccessKeyId())
&& CommonUtils.isNotEmpty(profile.getAccessKeySecret())) {
config.getEnv().setAccessKeyId(profile.getAccessKeyId());
config.getEnv().setAccessKeySecret(profile.getAccessKeySecret());
logger.info(String.format(
"Use the ak/sk specified in the aliyun config[%s]: %s",
profile.getName(),
aliyunConfigJson));
return true;
}
}
} catch (IOException ex) {
logger.warn(String.format("Load ak/sk from %s failed: %s", aliyunConfigJson, ex.getMessage()));
}
}
return false;
}
private void checkParamValid(DefaultConfigBean config) throws MojoExecutionException {
if (config.getApp() != null) {
checkAppParamValid(config.getApp());
}
}
private void checkAppParamValid(DefaultConfigBean.App app) throws MojoExecutionException {
if (app.getAppId() == null) {
throw new MojoExecutionException("'app_id' is not set");
}
if (app.getDeployType() != null && !"url".equals(app.getDeployType())) {
throw new MojoExecutionException("Currently this plugin only support 'url' deploy type");
}
}
private boolean equals(String a, String b) {
return a != null && b != null && a.equals(b);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy